Question
Part 1: Housekeeping You should read this lab all the way through BEFORE completing it. This lab is broken into multiple parts. Your goal is
Part 1: Housekeeping
You should read this lab all the way through BEFORE completing it.
This lab is broken into multiple parts. Your goal is to complete as many parts as you can by the end of the lab. If you do not complete all of them, you can still get full points; show that you have worked hard and put in effort and complete the minimum amount of problems (Part 2, 5, 6 and function main which tests your code) and this will be accomplishable.
The lab will be a continuation of our lectures on classes in Python. We will be implementing a new class called Point, which represents a point on the cartesian plane. We will introduce accessor and mutator methods, operator overloading, and class variables.
Part 2: Instance Variables and Class Representation (5 minutes)
Create the Point class. Have your constructor method construct the Point object with two instance variables x and y. These will represent the coordinates of the Point. Their default values should be 0 and 0. Then, create a __str__ method that returns a string representation of the Point, which should be in the form (x, y).
Part 3: Accessor and Mutator Methods
Create methods getX() and getY() that access a given Points respective coordinates.
Create methods setX() and setY() that mutate the respective coordinates.
Part 4: Class Variables (10 minutes)
Until now, weve learned that an instance of a class has its own variables, called instance variables. What if we wanted to keep a variable the same, or static, across all instances of a class, such as the count of the total instances that exist of the given class? This can be done with class variables, which are initialized outside of the constructor but within the class body.
For the Point class, create a class variable called COUNT and initialize it to 0. The purpose of this variable is to keep track of the number of Point objects that have been created. Add code within the constructor that increments COUNT by 1 every time a new Point object is created.
Part 5: More Methods (10 minutes)
Create a method called distanceFromPoint(aPoint) that returns the distance from this point to another point.
Create a method called distanceFromOrigin() that returns the distance from this point to the origin.
Part 6: Operator Overloading (50 minutes)
Until this point, the use of operators has been fairly straightforward. Integers can be compared with integers, strings with strings, and so on. However, with classes that are created by the user, it isnt so simple. For example, lets take a familiar class, the class Rectangle. If we were to instantiate two objects rect1 and rect2 of type Rectangle and attempt to execute the following command:
rect1 < rect2 |
How would the interpreter know what aspects of the objects to compare? Should it compare widths? Heights? Areas? Location on a plane? Unfortunately, the interpreter cannot read the programmers mind and therefore cannot execute this command without operator overloading. Operator overloading is needed when we need to use an operator for a different purpose than the interpreter intends. We can overload many of the operators found in Python, including (but not limited to) <, >, <=, >=, ==, +, -, *, and /. NOTE: When overloading an operator, it is only overloaded for the class in which the overloading is defined. For example, when we overload the < operator for our Point class, it will still work properly when executing a more simple statement like point1 < point2, where point1 and point2 are objects of the Point class.
Include in the Point class appropriate code so that Point objects can be compared using the comparison operators <, >, <=, >=, and ==, based on their distance from the origin. You may use your distanceFromOrigin() method to simplify this process!
Part 7: Testing Your Code (20 minutes)
Create a main function that does the following:
- Creates a Point object, named p1, with the coordinates (3, 4).
- Print the object p1. Remember that if p1 is an object of type Point, then the statement print(p1) automatically calls the __str__ method of the class. The output from this line should be (3, 4).
- Creates another Point object, named p2, with coordinates at (3, 0).
- Print the x and y coordinates of p2 using the getX() and getY() methods.
- Find and print the distance between p1 and p2.
- Print the results of comparing p1 and p2. Make sure to test all 6 comparison operators.
- Your code should allow using == and != to compare a Point object with an object of a different type. Print the result of using the equality and inequality operators to compare p1 with the string Hello.
- Using the class variable, print out the number of Point objects that have been created by the program.
Sample program output is as follows:
(3, 4) 3 0 The distance between (3, 4) and (3, 0) is 4.0 Testing the comparison operators in the order <, <=, >, >=, ==, and != p1 < p2? False p1 <= p2? False p1 > p2? True p1 >= p2? True p1 == p2? False p1 != p2? True p1 == "Hello"? False p1 != "Hello"? True Number of point objects created is 2 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
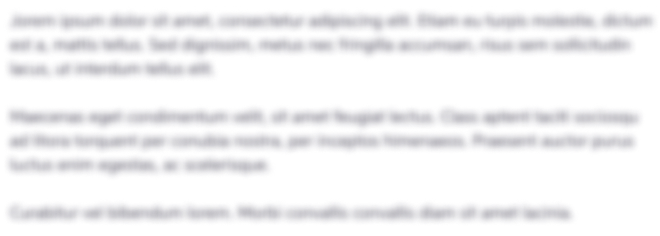
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started