Question
Part 1 #include using namespace std; void practiceCharMethod1(char c); void practiceCharMethod2(char c); void practiceCharMethod3(char c); void practiceCharMethod4(char c); int main() { practiceCharMethod1('f'); // Test with
Part 1
#include
void practiceCharMethod1(char c); void practiceCharMethod2(char c); void practiceCharMethod3(char c); void practiceCharMethod4(char c);
int main() { practiceCharMethod1('f'); // Test with different characters practiceCharMethod2('%'); // Test with different characters practiceCharMethod3('L'); // Test with different characters practiceCharMethod4('a'); // Test with different characters return 0; }
// ONLY COPY & PASTE THE SPECIFIC METHOD AS YOUR ANSWER
/* The function must print the inverse capitalization of the character passed as an argument */ void practiceCharMethod1(char character) { int result = 0; //YOUR CODE GOES HERE cout << "Inverse Capitalization: " << (char) result << endl; } /* Print only digits or alphanumeric characters. If other print "none". */ void practiceCharMethod2(char character) { cout << "The character is: "; //YOUR CODE GOES HERE }
/* You will need to verify the character passed as an argument. Some times it can only be a space or a punctuation character. Validate it before using it. If the character is other than a space or a punctuation, then it is okay to use it. */ void practiceCharMethod3(char character) { bool okToUse; //YOUR CODE GOES HERE cout << (okToUse ? "Character is valid" : "Character is not valid") << endl; } /* You will need to verify if the character passed as an argument is equal to 'x', 'y', or 'z' and if so, print "last three letters". It must be CASE INSENSITIVE, so if 'X', 'Y' or 'Z' is given, it must also print "last three letters". Otherwise you must print "none". */ void practiceCharMethod4(char character) { // YOUR CODE HERE }
Part 2
#include
void radius(double x1, double y1, double xCenter, double yCenter); void maxRoundedValue(double first, double second); void degreeAngleCosine (double d);
int main() {
radius(14.25, 13.68, 25.678, 10.32547); maxRoundedValue(12.445, 5.112); degreeAngleCosine(30);
return 0; }
// ONLY COPY & PASTE THE SPECIFIC METHOD AS YOUR ANSWER
/* It computes the radius r of a circle. Using functions from the Math Library complete the function that computes the radius of a circle using the formula r^2=(x-h)^2 +(y-k)^2 (see formula in moodle for reference), given the (x,y) coordinates of one point on its circumference and the (h,k) coordinates of its center. */ void radius(double x1, double y1, double xCenter, double yCenter) { double result = 0; //YOUR CODE GOES HERE cout << "The radius is: " << result << endl; };
/* This function prints the biggest value of two values. But first you need to round down the first argument and round up the second one. */ void maxRoundedValue(double first, double second) { cout << "The maximum rounded value is: "; //YOUR CODE GOES HERE
};
/* It prints the cosine of the angle given d, note that d is given in degrees and that you might want to turn it into radians for it to be useful. HINT: The value of pi is given as a variable in the starter code. The equation from degrees to radian is : x radians = (d degreees)* pi/180 */ void degreeAngleCosine (double d) { double pi = 2*acos(0.0); cout << "YOUR CODE HERE" << endl; };
Part 3
#include
void stringPractice1(string password); void stringPractice2(string name, string department, string college); void stringPractice3(string twoWords); void stringPractice4(string word);
int main() { stringPractice1("1234"); stringPractice2("Pedro", "CIIC", "Engineering"); stringPractice3("Two Words"); stringPractice4("Vehicle"); return 0; }
/* The function must verify the length of the string passed as an argument. If it is too long (more than 16 characters), or too short (less than 8 characters) it should print "password does not meet requirements", otherwise print "none". */
void stringPractice1(string password) { cout << "password does not meet requirements" << endl; }
/* The function must concatenate the string arguments in order to print a string in the following way: "Welcome
void stringPractice2(string name, string department, string college) { cout << "YOUR CODE HERE" << endl; }
/* The function must receive the string and replace the space in it with "&" (you may assume that the string has only one space) and then print the word with the space replaced by "&" */
void stringPractice3(string twoWords) { // YOUR CODE HERE cout << twoWords << endl; }
/* This function must erase the first 4 characters of a string, and append "***" at the end of the string. If given the word is "Vehicle", the result would be: "cle***". The altered string must be printed. */
void stringPractice4(string word) { // YOUR CODE HERE cout << word << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
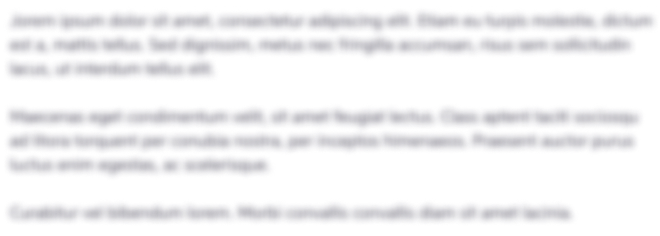
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started