Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Part 2: A Bar Chart Points: 35% Required API Implement class Chart, to represent a Bar Chart. Each Chart has: An array of objects of
Part 2: A Bar Chart
Points: 35%
Required API
Implement class Chart, to represent a Bar Chart. Each Chart has:
An array of objects of type Bar. This is provided by the user of the class through the constructor.
A title. This is a string that describes the chart.
A maximum size. This is a constant positive integer that controls the size of the chart.
You must implement the following public functions:
// constructor (n is the size of the array).
Chart(Bar b[], int n, string title, int MAX_SIZE);
// copy constructor
Chart(const Chart& other);
// destructor
~Chart();
// sorts the bars in the chart in "descending" order based on their length.
void sort();
// displays the top m bars in the chart.
void show(string caption, int m) const;
Constructors and Destructors
Use dynamic memory allocation in the constructor to create the array of Bar objects and copy into it the elements of the received array. Each Chart object must store and manage its own array of Bar objects, such that the array is not modifiable through any reference outside the class.
Implement the copy constructor also such that the object gets its own independent copy of the array of Bar objects in the other Chart.
The destructor must deallocate any dynamically allocated memory.
Sorting
The function sort() must sort the bars in the chart in decreasing order based on their lengths. Modify the following implementation of Bubble Sort to achieve this:
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(arr[j], arr[j+1]);
Displaying the Chart
The function show(string caption, int m) must print out the chart as follows:
Print the title of the chart on a separate line.
Print the first m Bar objects in the array of bars using the print function in the Bar class. Use a scaling factor as described below.
Print the given caption on a separate line.
To compute the rescaling factor, you need to find the length of the longest bar in the chart.
Assume the length of the longest bar is max_length, the scaling factor is then MAX_SIZE / max_length.
Example:
Assume the chart has 3 bars with:
lengths 10, 50 and 100.
labels "label 1", "label 2" and "label 3".
colors DARK_RED, DARK_BLUE and DARK_GREEN.
Assume also that MAX_SIZE = 50 and the title is "This is a test title".
The scaling factor sent to the print() function should be 50 / 100 = 0.5 .
Calling show("This is a test caption", 3) should produce the following:
Note the blank lines between the bars.
Test Client
In order to test your implementation of class Chart, write a program to perform the following:
Create an array of 16 objects of type Bar. For each object, perform the following:
Assign a random length between 100 and 300 inclusive.
Assign a random color between 1 and 16 inclusive.
Assign the label "test" .
Use the created array of Bar objects to create an object of type Chart and set the following:
Title: "Title: A Random Chart."
Maximum Size = 60.
Show the chart (all the bars) with the caption "A random chart".
Sort the chart and then show the top 10 bars with the caption "A random chart after sorting".
The output of the program should look something like this (colors differ based on the used console):
Implementation Requirements
Separate the interface from the implementation, but both must be in the same file. I.e., all of your code (header + implementation + main) must be in Chart.cpp. You will need to copy your implementation of class Bar into Bar.cpp without the main() function, as Chart.cpp will have the main() function.
Use assertions to ensure that the array received in the constructor is not NULL and the length of the array and MAX_SIZE are greater than 0. Use assertions also to ensure in show(string caption, int m) that 0 <= m <= n.
You are allowed to add private data members and private functions as you wish. You are not allowed to add any public data members or functions beside what the assignment requires.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
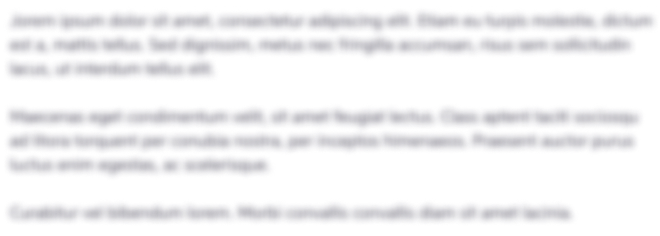
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started