Part 2: Generating a series of random numbers can be achieved in Java by using the java.util.Random class. However, the numbers generated are not truly random, they are pseudorandom. That is, they are produced by algorithms that generate a fixed but random-looking sequence of numbers. In this assignment, you will learn the basic ideas behind generating pseudorandom numbers and implement your own pseudorandom number generator. Knowing how pseudorandom numbers are generated and their limitations may help you in the future when you use pseudorandom numbers in simulations and other applications.
Do programming projects 11, 12, and 13 on pages 99-100 of the textbook. In project 13 you are asked to experiment with different values of the multiplier, increment, and modulus. Find your own values of constants (different from the ones in the book)that are good (which result in about 10% of the numbers in each interval). Also, find values of multiplier, increment, and modulus that are not good (which result in some intervals having no numbers).
Use Eclipse to create a new project with the name, say, Lab2. In the project create three new classes as described below.
1)Pseudorandom.java - implements a class that generates pseudorandom numbers. You must use the principle of information hiding in your implementation (instance variables must be private).
Instance variables of the Pseudorandom class should be multiplier, increment, modulus, and seed (of type int).
You need to implement the followingmethods (that are described in the projects 11 and 12):
- constructor with initial seed, multiplier, increment, and modulus as parameters,
- changeSeed(int new_seed)
- nextInt()that generates and returns a pseudorandom integer number ,
- nextDouble()that generates and returns a pseudorandom doublenumber in the range [0..1) (project 12 from the textbook). Note that modulusmust not be equal to 0 in order for nextIntandnextDoublemethods to work.
2)TestPseudorandom.java - tests Pseudorandomclass. It should prompt the user to enter initial values for seed, multiplier, increment, and modulus, and include test calls to all of the constructors and methods.
3)TestDistribution.java -determines distribution of numbers (project 13 from the textbook). It should prompt the user to enter initial values for seed, multiplier, increment, and modulus, and output a table with numbers of occurrences for each range.
Use your TestDistribution class from project 13 to experiment with different values of the multiplier, increment, and modulus. Prepare a text file (*.txt file) which contains
- good values of the multiplier, increment, and modulus that you found (should be different from the values provided in the textbook), together with the output of your TestDistribution program when run with these numbers, and
- not good values of the multiplier, increment, and modulus that you found, together with the output of your TestDistribution program when run with these numbers.
Mark which values are good and which are not good in the text file.
- Specifications for all your methods should be included as comments in your code. Method specifications should follow the guidelines from Section 1.1 (pp.7-8), that is, include a short introduction, parameter description, preconditions (if any), postcondition or returns condition, the throws list (if any). Also, please use inline comments, meaningful variable names, indentation, formatting and whitespace throughout your program to improve its readability. Tips for naming variables.
- Thoroughly test your code.
PROGRAMMING PROJECTS 11- 13
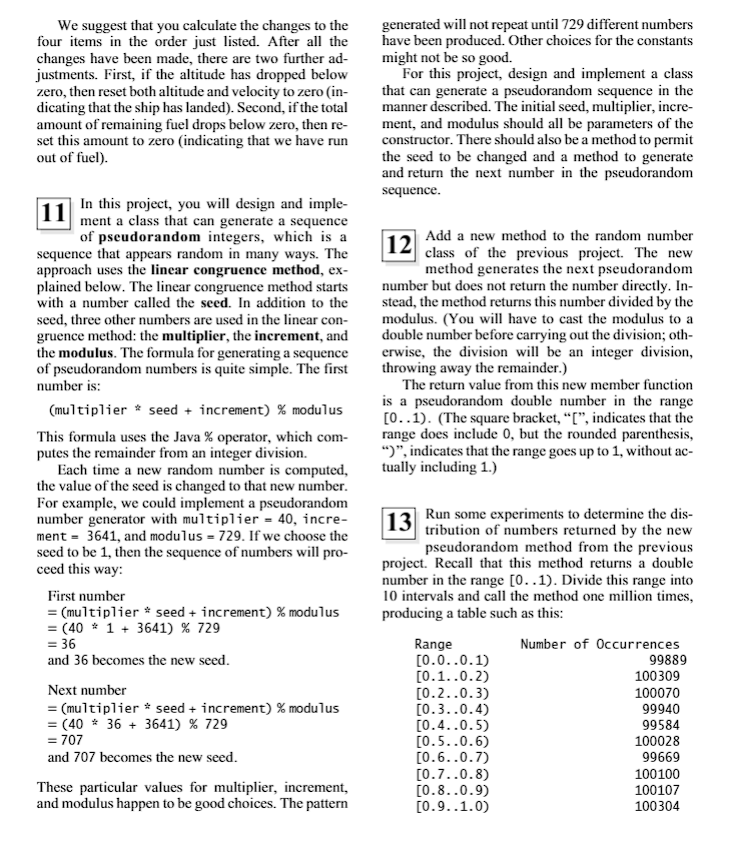
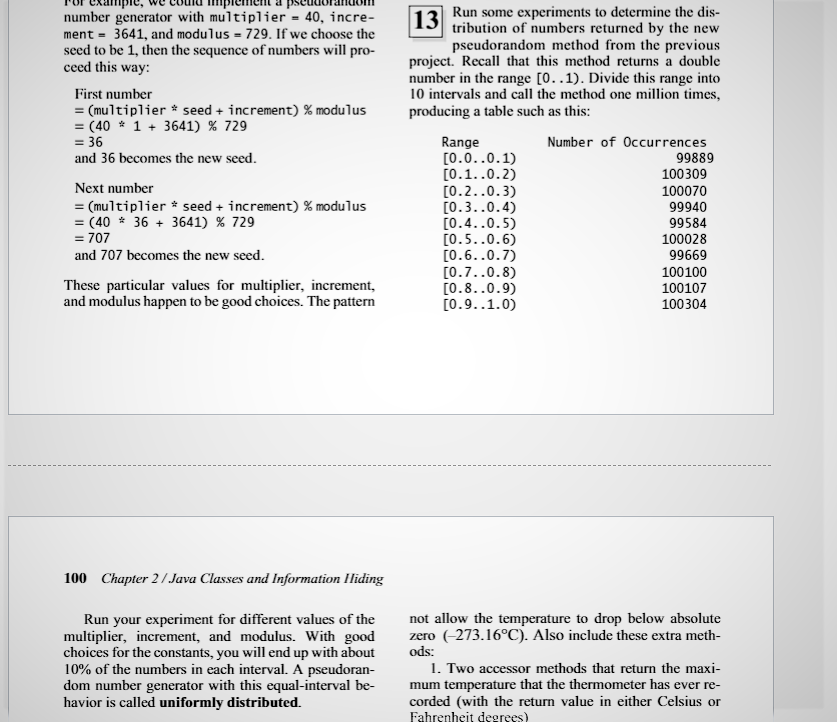
We suggest that you calculate the changes to the four items in the order just listed. After all the changes have been made, there are two further ad- justments. First, if the altitude has dropped below zero, then reset both altitude and velocity to zero in- dicating that the ship has landed). Second, if the total amount of remaining fuel drops below zero, then re- set this amount to zero (indicating that we have run out of fuel). generated will not repeat until 729 different numbers have been produced. Other choices for the constants might not be so good. For this project, design and implement a class that can generate a pseudorandom sequence in the manner described. The initial seed, multiplier, incre- ment, and modulus should all be parameters of the constructor. There should also be a method to permit the seed to be changed and a method to generate and return the next number in the pseudorandom sequence. 12 Add a new method to the random number class of the previous project. The new method generates the next pseudorandom number but does not return the number directly. In- stead, the method returns this number divided by the modulus. (You will have to cast the modulus to a double number before carrying out the division; oth- erwise, the division will be an integer division, throwing away the remainder.) The return value from this new member function is a pseudorandom double number in the range [0..1). (The square bracket, "[", indicates that the range does include 0, but the rounded parenthesis, )", indicates that the range goes up to 1, without ac- tually including 1.) 11 In this project, you will design and imple- ment a class that can generate a sequence of pseudorandom integers, which is a sequence that appears random in many ways. The approach uses the linear congruence method, ex- plained below. The linear congruence method starts with a number called the seed. In addition to the seed, three other numbers are used in the linear con- gruence method: the multiplier, the increment, and the modulus. The formula for generating a sequence of pseudorandom numbers is quite simple. The first number is: (multiplier * seed + increment) % modulus This formula uses the Java % operator, which com- putes the remainder from an integer division. Each time a new random number is computed, the value of the seed is changed to that new number. For example, we could implement a pseudorandom number generator with multiplier = 40, incre- ment = 3641, and modulus = 729. If we choose the seed to be 1, then the sequence of numbers will pro- ceed this way: First number = (multiplier * seed + increment) % modulus = (40 * 1 + 3641) % 729 = 36 and 36 becomes the new seed. Next number = (multiplier * seed + increment) % modulus = (40 * 36 + 3641) % 729 = 707 and 707 becomes the new seed. These particular values for multiplier, increment, and modulus happen to be good choices. The pattern 13 Run some experiments to determine the dis- tribution of numbers returned by the new pseudorandom method from the previous project. Recall that this method returns a double number in the range [0..1). Divide this range into 10 intervals and call the method one million times, producing a table such as this: Range Number of Occurrences [0.0..0.1) 99889 [0.1..0.2) 100309 [0.2..0.3) 100070 [0.3..0.4) 99940 [0.4..0.5) 99584 [0.5..0.6) 100028 [0.6..0.7) 99669 [0.7..0.8) 100100 [0.8..0.9) 100107 [0.9..1.0) 100304 number generator with multiplier = 40, incre- ment = 3641, and modulus = 729. If we choose the seed to be 1, then the sequence of numbers will pro- ceed this way: First number = (multiplier * seed + increment) % modulus = (40 * 1 + 3641) % 729 = 36 and 36 becomes the new seed. Next number = (multiplier * seed + increment) % modulus = (40 * 36 + 3641) % 729 = 707 and 707 becomes the new seed. These particular values for multiplier, increment, and modulus happen to be good choices. The pattern 13 Runsome experiments to determine the dis- pseudorandom method from the previous project. Recall that this method returns a double number in the range [0..1). Divide this range into 10 intervals and call the method one million times, producing a table such as this: Range Number of Occurrences [0.0..0.1) 99889 [0.1..0.2) 100309 [0.2..0.3) 100070 [0.3..0.4) 99940 [0.4..0.5) 99584 [0.5..0.6) 100028 [0.6..0.7) 99669 [0.7..0.8) 100100 [0.8..0.9) 100107 [0.9..1.0) 100304 100 Chapter 27 Java Classes and Information Iliding Run your experiment for different values of the multiplier, increment, and modulus. With good choices for the constants, you will end up with about 10% of the numbers in each interval. A pseudoran- dom number generator with this equal-interval be- havior is called uniformly distributed. not allow the temperature to drop below absolute zero (-273.16C). Also include these extra meth- ods: 1. Two accessor methods that return the maxi- mum temperature that the thermometer has ever re- corded (with the return value in either Celsius or Fahrsnheit deerees) We suggest that you calculate the changes to the four items in the order just listed. After all the changes have been made, there are two further ad- justments. First, if the altitude has dropped below zero, then reset both altitude and velocity to zero in- dicating that the ship has landed). Second, if the total amount of remaining fuel drops below zero, then re- set this amount to zero (indicating that we have run out of fuel). generated will not repeat until 729 different numbers have been produced. Other choices for the constants might not be so good. For this project, design and implement a class that can generate a pseudorandom sequence in the manner described. The initial seed, multiplier, incre- ment, and modulus should all be parameters of the constructor. There should also be a method to permit the seed to be changed and a method to generate and return the next number in the pseudorandom sequence. 12 Add a new method to the random number class of the previous project. The new method generates the next pseudorandom number but does not return the number directly. In- stead, the method returns this number divided by the modulus. (You will have to cast the modulus to a double number before carrying out the division; oth- erwise, the division will be an integer division, throwing away the remainder.) The return value from this new member function is a pseudorandom double number in the range [0..1). (The square bracket, "[", indicates that the range does include 0, but the rounded parenthesis, )", indicates that the range goes up to 1, without ac- tually including 1.) 11 In this project, you will design and imple- ment a class that can generate a sequence of pseudorandom integers, which is a sequence that appears random in many ways. The approach uses the linear congruence method, ex- plained below. The linear congruence method starts with a number called the seed. In addition to the seed, three other numbers are used in the linear con- gruence method: the multiplier, the increment, and the modulus. The formula for generating a sequence of pseudorandom numbers is quite simple. The first number is: (multiplier * seed + increment) % modulus This formula uses the Java % operator, which com- putes the remainder from an integer division. Each time a new random number is computed, the value of the seed is changed to that new number. For example, we could implement a pseudorandom number generator with multiplier = 40, incre- ment = 3641, and modulus = 729. If we choose the seed to be 1, then the sequence of numbers will pro- ceed this way: First number = (multiplier * seed + increment) % modulus = (40 * 1 + 3641) % 729 = 36 and 36 becomes the new seed. Next number = (multiplier * seed + increment) % modulus = (40 * 36 + 3641) % 729 = 707 and 707 becomes the new seed. These particular values for multiplier, increment, and modulus happen to be good choices. The pattern 13 Run some experiments to determine the dis- tribution of numbers returned by the new pseudorandom method from the previous project. Recall that this method returns a double number in the range [0..1). Divide this range into 10 intervals and call the method one million times, producing a table such as this: Range Number of Occurrences [0.0..0.1) 99889 [0.1..0.2) 100309 [0.2..0.3) 100070 [0.3..0.4) 99940 [0.4..0.5) 99584 [0.5..0.6) 100028 [0.6..0.7) 99669 [0.7..0.8) 100100 [0.8..0.9) 100107 [0.9..1.0) 100304 number generator with multiplier = 40, incre- ment = 3641, and modulus = 729. If we choose the seed to be 1, then the sequence of numbers will pro- ceed this way: First number = (multiplier * seed + increment) % modulus = (40 * 1 + 3641) % 729 = 36 and 36 becomes the new seed. Next number = (multiplier * seed + increment) % modulus = (40 * 36 + 3641) % 729 = 707 and 707 becomes the new seed. These particular values for multiplier, increment, and modulus happen to be good choices. The pattern 13 Runsome experiments to determine the dis- pseudorandom method from the previous project. Recall that this method returns a double number in the range [0..1). Divide this range into 10 intervals and call the method one million times, producing a table such as this: Range Number of Occurrences [0.0..0.1) 99889 [0.1..0.2) 100309 [0.2..0.3) 100070 [0.3..0.4) 99940 [0.4..0.5) 99584 [0.5..0.6) 100028 [0.6..0.7) 99669 [0.7..0.8) 100100 [0.8..0.9) 100107 [0.9..1.0) 100304 100 Chapter 27 Java Classes and Information Iliding Run your experiment for different values of the multiplier, increment, and modulus. With good choices for the constants, you will end up with about 10% of the numbers in each interval. A pseudoran- dom number generator with this equal-interval be- havior is called uniformly distributed. not allow the temperature to drop below absolute zero (-273.16C). Also include these extra meth- ods: 1. Two accessor methods that return the maxi- mum temperature that the thermometer has ever re- corded (with the return value in either Celsius or Fahrsnheit deerees)