Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Part 2: Mutual Fund InvestorTrack App (50 pts) For the next part of your lab, you will use the BST class you wrote above to
Part 2: Mutual Fund InvestorTrack App (50 pts)
- For the next part of your lab, you will use the BST class you wrote above to create an application that helps users to track investments in mutual funds.
- Using the starter code below, fill in the empty method bodies. Note that you are not allowed to add any additional methods or member variables to these class (including no DecimalFormat member variables) or you will not receive credit for this part of the assignment.
- You may not make any additional import statements at the top of any of the below files
MutualFund.java
/** * MutualFund.java * @author PARTNER_NAME_1 * @author PARTNER_NAME_1 * CIS 22C, Lab 5 */ import java.text.DecimalFormat; import java.util.Comparator; public class MutualFund { private final String fundName; private final String ticker; private double pricePerShare; /**CONSTRUCTORS*/ /** * One-argument constructor that assigns * a fundName and "no ticker" to the ticker * and -1 to pricePerShare * @param fundName the fund name */ public MutualFund(String fundName) { //fill in here } /** * Three-argument constructor * @param fundName the mutual fund name * @param ticker the ticker symbol * @param pricePerShare the price per share */ public MutualFund(String fundName, String ticker, double pricePerShare) { //fill in here } /**ACCESSORS*/ /** * Accesses the name of the fund * @return the fund name */ public String getFundName() { return null; } /** * Accesses the ticker symbol * @return the ticker symbol */ public String getTicker() { return null; } /** * Accesses the price per share * @return the price per share */ public double getPricePerShare() { return -1; } /**MUTATORS*/ /** * Updates the share price * @param pricePerShare the new share price */ public void setPricePerShare(double pricePerShare) { //fill in here } /**ADDITIONAL OPERATIONS*/ /** * Creates a String of the mutual fund information * in the format: ** * Share Price: $ * */ @Override public String toString() { return " "; } }
MutualFundAccount.java
/** * MutualFundAccount.java * @author PARTNER_NAME_1 * @author PARTNER_NAME_2 * CIS 22C, Lab 5 */ import java.text.DecimalFormat; import java.util.Comparator; public class MutualFundAccount { private double numShares; private MutualFund mf; /**CONSTRUCTORS*/ /** * One-argument constructor * @param mf the mutual fund for this account * Assigns numShares to 0 */ public MutualFundAccount(MutualFund mf) { //fill in here } /** * Two-argument constructor * @param numShares total shares of the mutual fund * @param mf the mutual fund */ public MutualFundAccount(double numShares, MutualFund mf) { //fill in here } /** * Two-argument constructor * @param mf the mutual fund * @param numShares total shares of the mutual fund */ public MutualFundAccount(MutualFund mf, double numShares) { //fill in here } /**ACCESSORS*/ /** * Accesses the total number of shares * @return the total shares */ public double getNumShares() { return -1.0; } /** * Accesses the mutual fund * @return the mutual fund */ public MutualFund getMf() { return null; } /**MUTATORS*/ /** * Increases/Decreases the total shares * by the given amount * @param numShares the amount to increase * or decrease */ public void updateShares(double numShares) { //fill in here } /** * Creates a String of the mutual fund * account information in the following format: ** Total Shares: * Value */ @Override public String toString() { return " "; } } //end class MutualFundAccount class NameComparator implements Comparator { /** * Compares the two mutual fund accounts by name of the fund * uses the String compareTo method to make the comparison * @param account1 the first MutualFundAccount * @param account2 the second MutualFundAccount */ @Override public int compare(MutualFundAccount account1, MutualFundAccount account2) { return -1; } } //end class NameComparator class ValueComparator implements Comparator { /** * Compares the two mutual fund accounts by total value * determines total value as number of shares times price * per share * uses the static Double compare method to make the * comparison * @param account1 the first MutualFundAccount * @param account2 the second MutualFundAccount */ @Override public int compare(MutualFundAccount account1, MutualFundAccount account2) { return -1; } }
List.java
- Add the following methods to your List.java class from Lab 3:
/** * Points the iterator at first * and then advances it to the * specified index * @param index the index where * the iterator should be placed * @precondition 0 < index <= length * @throws IndexOutOfBoundsException * when precondition is violated */ public void iteratorToIndex(int index) throws IndexOutOfBoundsException{ //fill in here } /** * Searches the List for the specified * value using the linear search algorithm * @param value the value to search for * @return the location of value in the * List or -1 to indicate not found * Note that if the List is empty we will * consider the element to be not found * post: position of the iterator remains * unchanged */ public int linearSearch(T value) { return -1; }
CustomerInterface.java
- Note that you may add as many additional methods and variables as you would like to the below starter code
- You must use the given BSTs and List objects given in the starter code, and no other structures
- You may not make any additional import statements at the top of this file
- You must use your BST class from Part 1 of this lab and your List class from Lab 3 to receive credit.
/** * CustomerInterface.java * @author PARTNER1_NAME_HERE * @authoer PARTNER2_NAME_HERE * CIS 22C, Lab 5 */ import java.io.*; import java.text.DecimalFormat; import java.util.Scanner; public class CustomerInterface { public static void main(String[] args) { BSTaccount_value = new BST<>(); BST account_name = new BST<>(); List funds = new List<>(); String first, last, email, password, mutualName, ticker; double cash, sharePrice, numShares, fee; File file = new File("mutual_funds.txt"); Scanner input = new Scanner(file); //code to read in from file input.close(); input = new Scanner(System.in); input.close(); } }
mutual_funds.txt
- Copy and paste the below contents into a text file in Eclipse named mutual_funds.txt
- Rick-click the project folder.
- Go to File->New->File
Total Bond Market Index Admiral Shares VBTLX 11.61 Intermediate-Term Investment-Grade VFICX 10.64 Mid-Cap Index Admiral Shares VIMAX 230.09 Municipal Money Market VMSXX 1.00 Total Stock Market Index Admiral Shares VTSAX 86.49 International Growth VWIGX 44.20 U.S. Growth VWUSX 62.41
Step by Step Solution
There are 3 Steps involved in it
Step: 1
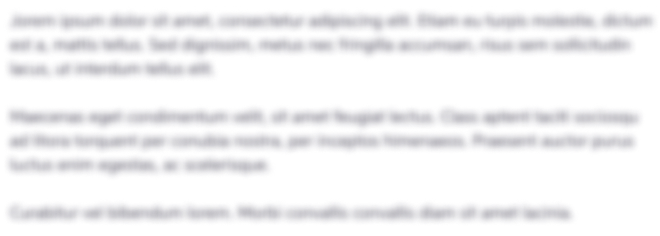
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started