Question
(Part A) : Sports Analytics 60 points The data analyst for ESPN needs to calculate the statistics for the NBA Los Angeles Clippers. Write a
(Part A) : Sports Analytics 60 points
The data analyst for ESPN needs to calculate the statistics for the NBA Los Angeles Clippers. Write a program to calculate the two and three point field goal percentages made by the Team based on the number of total shots attempted and total shots made. You can enter any statistics to test program.
In addition to main() (worth 25 pts), also use the following seven functions in your program
(worth 5pts each) :
getTotalNumberOfTwoPointsAttempted() : a value-returning function that asks for the total number of two points attempted. Use a return statement to send it to main().
getNumberOfTwoPointsMade() : a value-returning function that asks for the number of two points made, relative to the number of two points attempted. Use a return statement to send it to main().
getTotalNumberOfThreePointsAttempted() : a value-returning function that asks for the total number of three points attempted. Use a return statement to send it to main().
getNumberOfThreePointsMade() : a value-returning function that asks for the number of three points made, relative to the number of three points attempted. Use a return statement to send it to main().
getPercentageOfTwoPointsMade() : a value-returning function that calculates the the percentage of two points made. Use a return statement to send it to main().
getPercentageOfThreePointsMade() : a value-returning function that calculates the the percentage of three points made. Use a return statement to send it to main().
IsBetterThanChance() : a value-returning function that returns true or false (true: if the team made 50% or above percentages of total shots and false: if the team made less than 50% percentages of shots) Display message in main() based on this return value.
NOTE:
- The problem requires that you determine the parameters needed for each function.
- The main() function should display the statistics on the computer screen.
- No Global non-constant variables should be used.
(Part B) : Pass by value versus pass by reference 40 points
Write a C++ program which prompts user to choose from the two alternative funtions specified below, each of which returns a random message. The random number generator function should be called from within the functions.
The two functions are:
- Function messageByValue is a value returning function that returns the message by value and
- Function messageByReference is a void function that returns the message by reference.
Each function returns one of four random messages:
- Sorry. Youre not close enough! returned 25% of the time
- Oh, youre almost there? returned 15% of the time
- Aw cmon, you can do better than that! returned 20% of the time
- Youre up big. Getting closer to breaking your old record returned 40% of the time
NOTE: Use a while loop to continually run program until a -1 is entered to Terminate program.
- The problem requires that you determine the parameters needed for each function.
- The message should be displayed in main().
- No Global non-constant variables should be used.
C++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
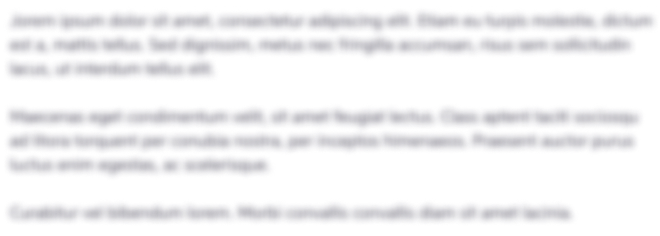
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started