Question
Part B: 1. To examine the differences between the code, we will implement the same code in Java, C++ or C#. Example Java Code: Qsort
Part B:
1. To examine the differences between the code, we will implement the same code in Java, C++ or C#.
Example Java Code:
Qsort class:
class Qsort {
/* This function takes last element and places it in the correct position in the sorted array. Then places smaller elements to the left and greater to the right
*/
int partition(int arr[], int low, int high)
{
int pivot = arr[high];
int i = (low-1); // index of smaller element
for (int j=low; j
{
// If current element is smaller than or
// equal to pivot element
if (arr[j] <= pivot)
{
i++;
// swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// swap array places
int temp = arr[i+1];
arr[i+1] = arr[high];
arr[high] = temp;
return i+1;
}
/* The main function that implements QuickSort()
arr[] --> Array to be sorted,
low --> Starting index,
high --> Ending index */
void sort(int arr[], int low, int high)
{
if (low < high)
{
/* pi is partitioning index, arr[pi] is
now at right place */
int pi = partition(arr, low, high);
// Recursively sort elements before
// partition and after partition
sort(arr, low, pi-1);
sort(arr, pi+1, high);
}
}
}
Quicksort class (including main):
public class Quicksort {
public static void main(String[] args) {
int arr[] = {1, 2, 5, 8, 0, 4, 3};
int n = arr.length;
Qsort ob = new Qsort();
ob.sort(arr, 0, n-1);
System.out.println("sorted array");
printArray(arr);
}
static void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i
System.out.print(arr[i]+" ");
System.out.println();
}
}
Test the array above (same as in scheme) and your own input:
Screenshot of output below:
Screenshot of your own input for the array:
2. Implement Fibonacci sequence in Java, C# or C++
Java Example below:
public class FibSequence {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
int count = 16;
int[] feb = new int[count];
feb[0] = 0;
feb[1] = 1;
for(int i = 2; i < count; i++) {
feb[i] = feb[i-1] + feb[i-2];
}
//include code here to print out the 10thelement (actually feb[10] and the 15thelement)
}
}
Include screenshot of the code showing the results for printing the 10thelement and the 15thelements:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
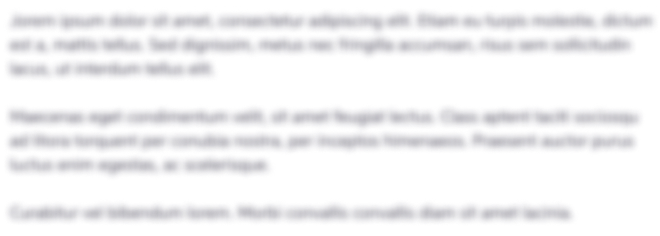
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started