Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Part B (100 points): Implement a Rock-Paper-Scissors Game Write a Python script that allows a human player to play the Rock-Paper-Scissors game against the computer.
Part B (100 points): Implement a Rock-Paper-Scissors Game
- Write a Python script that allows a human player to play the Rock-Paper-Scissors game against the computer.
- To play the game, the human player chooses one of "rock," "paper," or "scissors." The computer also chooses, randomly with equal probability, one of these three plays.
- These phases determine the winner for each combination: "rock crushes scissors", "scissors cuts paper," and "paper covers rock."
- In this diagram, each arrow points from the winner to the user.
- If both the human and the computer choose the same play, there is a tie.
- Here is a sample script output. The human player's choices are shown in red.
Enter human play (rock, paper, scissors): paper Computer plays rock. Paper covers rock. Human wins. Score: Human 1, Computer 0. Enter human play (rock, paper, scissors): scissors Computer plays scissors. Tie. Score: Human 1.5, Computer 0.5. Enter human play (rock, paper, scissors): scissors Computer plays rock. Rock crushes scissors. Computer wins. Score: Human 1.5, Computer 1.5.
- You can base your script on this pseudocode:
set human score to 0.0 set computer score to 0.0 while true input human play with prompt choose random number from 0, 1, or 2 if random number is 0 set computer play to rock else if random number is 1 set computer play to paper else if random number is 2 set computer play to scissors end if human plays rock and computer plays rock Result is tie. Add 0.5 to each score. else if human plays rock and computer plays paper Result is computer wins. Add 1 to computer score. else if human plays rock and computer plays scissors Result is human wins. Add 1 to human score. else if human plays paper and computer plays rock Result is human wins. Add 1 to human score. else if human plays paper and computer plays paper Result is tie. Add 0.5 to each score. else if human plays paper and computer plays scissors Result is computer wins. Add 1 to computer score. else if human plays scissors and computer plays rock Result is computer wins. Add 1 to computer score. else if human plays scissors and computer plays paper Result is human wins. Add 1 to human score. else if human plays scissors and computer plays scissors Result is tie. Add 0.5 to each score. end display human score and computer score end
- Use Control-C to interrupt and terminate your script when you are finished playing the game.
- If hope to get an A in this class, you should implement this enhanced game called Rock-Paper-Scissors-Lizard-Spock,from the Big Bang Theory TV show. The rules of the enhanced game are shown in this diagram. Whereas the original game has 9 (3 3) possible move combinations, the enhanced game has 25 (5 5) move combinations. You can even order a shirt with the Rock-Paper-Scissors-Lizard-Spock diagram.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
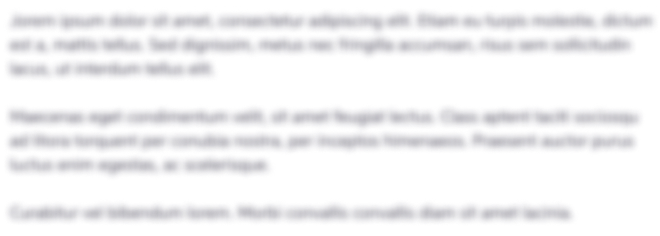
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started