Question
Part B: Class Time You will develop a new data type to model the time on a 24 hour clock, where the three data members
Part B: Class Time
You will develop a new data type to model the time on a 24 hour clock, where the three data members represent the hour, the minutes and the seconds. Use the date.py module as a guide.
It will be named class Time, and each object of that type will have three instance variables (__hour,
_mins and _secs). The module will be named clock.py.
7. Develop the function member named from_str which will be used to update an object of type Time after it has been created. That function will receive two parameters: a reference to the current object (self) and a string of the form hh:mm:ss.
a. Assume that the string parameter can be split into three integers.
That is, assume that the parameter is in the format specifiedno errors.
b. Assign those three integers to the three instance variables.
c. Add a doc string to the constructor documenting the purpose of the function.
8. Save the file containing your class as clock.py, then experiment with your module in the iPython shell:
>>> import clock
>>> A = clock.Time()
>>> print( A )
>>> A.from_str('07:12:03')
>>> print( A )
>>> A
9. We provide the Python program named clockDemo.py that demonstrates the use of your class Time. Test your class using clockDemo.py.
10. Create a new file named lab11b.py which contains the source code from the files named
clock.py and clockDemo.py. That is, create a file which contains both the definition of class Time and the demonstration program. The class definition should be at the top of the file. To create that stand alone file you will need to remove (or comment out) the import clock line (you dont need to import it because it is already in the file). Also, since you have not imported clock you need to change clock.Time to Time. Test that your file is working before submitting.
The date.py file
###########################################################################
## Class Date
###########################################################################
class Date( object ):
__name = [ "Invalid", "January", "February", "March", "April",
"May", "June", "July", "August", "September", "October",
"November", "December" ]
__days = [ 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 ]
def __init__( self, month=0, day=0, year=0 ):
""" Construct a Date using three integers. """
self.__month = month
self.__day = day
self.__year = year
self.__valid = self.__validate()
def __repr__( self ):
""" Return a string as the formal representation a Date. """
out_str = "Class Date: {:02d}/{:02d}/{:04d}" \
.format( self.__month, self.__day, self.__year )
return out_str
def __str__( self ):
""" Return a string (mm/dd/yyyy) to represent a Date. """
out_str = "{:02d}/{:02d}/{:04d}" \
.format( self.__month, self.__day, self.__year )
return out_str
def __validate( self ):
# Check the month, day and year for validity
# (does not account for leap years)
flag = False
if (1 <= self.__month <= 12) and \
(1 <= self.__day <= Date.__days[self.__month] ) and \
(0 <= self.__year):
flag = True
return flag
def is_valid( self ):
""" Return Boolean flag (valid date?) """
return self.__valid
def from_iso( self, iso_str ):
""" Convert a string (yyyy-mm-dd) into a Date. """
year, month, day = [ int( n ) for n in iso_str.split( '-' )]
self.__month = month
self.__day = day
self.__year = year
self.__valid = self.__validate()
def from_mdy( self, mdy_str ):
""" Convert a string (Mmmmm dd, yyyy) into a Date. """
mdy_list = mdy_str.replace(",","").split()
self.__month = Date.__name.index( mdy_list[0] )
self.__day = int( mdy_list[1].strip() )
self.__year = int( mdy_list[2].strip() )
self.__valid = self.__validate()
def to_iso( self ):
""" Return a string (yyyy-mm-dd) to represent a Date. """
iso_str = "{:04d}-{:02d}-{:02d}" \
.format( self.__year, self.__month, self.__day )
return iso_str
def to_mdy( self ):
""" Return a string (Mmmmm dd, yyyy) to represent a Date. """
mdy_str = "{:s} {:d}, {:04d}" \
.format( Date.__name[self.__month], self.__day, self.__year )
return mdy_str
clockDemo.pyfile
import clock
A = clock.Time( 12, 25, 30 )
print( A )
print( repr( A ) )
print( str( A ) )
print()
B = clock.Time( 2, 25, 3 )
print( B )
print( repr( B ) )
print( str( B ) )
print()
C = clock.Time( 2, 25 )
print( C )
print( repr( C ) )
print( str( C ) )
print()
D = clock.Time()
print( D )
print( repr( D ) )
print( str( D ) )
print()
D.from_str( "03:09:19" )
print( D )
print( repr( D ) )
print( str( D ) )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
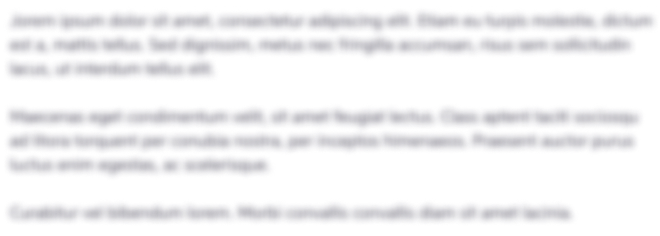
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started