Answered step by step
Verified Expert Solution
Question
1 Approved Answer
//part b /** * This is array based circular queue which is generic type * * @param */ public class CircularQueue { private final int
//part b
/** * This is array based circular queue which is generic type * * @param*/ public class CircularQueue { private final int DEFCAP = 100; // default capacity private T[] queue; // array that holds queue elements private int front; // index of front of queue private int back; // index of back of queue /** * Parameterized constructor which take size for initializing queue * @param maxSize */ public CircularQueue(int maxSize) { queue = (T[]) new Object[maxSize]; //setting front and back to same at starting front = -1; back = -1; } /** * This constructor initializes the queue with default capacity */ public CircularQueue() { queue = (T[]) new Object[DEFCAP]; //setting front and back to same at starting front = -1; back = -1; } /** * This method add element in queue */ public void enqueue(T element){ //Check if size is same as max capacity of queue if yes then give error Message if(isFull()) { System.out.println("Queue is full can't add more elements."); return; } if (back == front && front == -1) { front += 1; } //incrementing back position back = (back+1) % queue.length; queue[back] = element; } /** * This method remove element from queue */ public T dequeue() { //If size is 0 then print the message if(isEmpty()) { System.out.println("Queue is empty."); return null; } //getting element in front before remove T data = queue[front]; if(front == back) { front = -1; back = -1; } else { //setting front to next element in queue front = (front+1) % queue.length; } return data; } /** * This method will check if queue is full or not, if full return true otherwise false */ public boolean isFull() { return ((back + 1)% queue.length == front); } /** * This method return true if queue is empty otherwise false */ public boolean isEmpty() { return (front == back && back ==-1); } /** * This method returns number of elements present in queue */ public int size() { if(isFull()) { return queue.length; } return ((back-front) + queue.length)%queue.length; } /** * This method will return front element from queue * @return */ public T front() { return isEmpty() ? null : queue[front]; } }
/** * This is test class to test CircularQueue * */ public class CircularQueueDriver { public static void main(String[] args) { //creating CircularQueue with size of 5 CircularQueueModify your implementation for part B so that each teller has a separate queue. When customers arrive, they enter the shortest queue (and stay in that one, never switching queues). Run both versions of the algorithm for an identical set of 100 customers with the same arrival and transaction times. Compare the average wait time for the two methods. Which seems to be better? Mservers with Mqueues Mservers with 1 queue V.S. Wait Here 023 000queue = new CircularQueue (5); //checking queue status System.out.println("Is queue empty: "+queue.isEmpty()); System.out.println("Is queue full: "+queue.isFull()); System.out.println("Size of queue: "+queue.size()); System.out.println("Front of queue: "+queue.front()); System.out.println("======================================"); //adding elements into queue queue.enqueue("Apple"); queue.enqueue("Banana"); queue.enqueue("Grapes"); queue.enqueue("Orange"); queue.enqueue("Watermelon"); //trying to add extra element in full queue queue.enqueue("Cherry"); //checking queue status after insertion of elements System.out.println("Queue after adding 5 elements"); System.out.println("Is queue empty: "+queue.isEmpty()); System.out.println("Is queue full: "+queue.isFull()); System.out.println("Size of queue: "+queue.size()); System.out.println("Front of queue: "+queue.front()); System.out.println("======================================"); System.out.println("Dequeued: "+queue.dequeue()); System.out.println("Front after dequeue: "+queue.front()); System.out.println("Dequeued: "+queue.dequeue()); System.out.println("Front after dequeue: "+queue.front()); System.out.println("Dequeued: "+queue.dequeue()); System.out.println("Front after dequeue: "+queue.front()); System.out.println("Dequeued: "+queue.dequeue()); System.out.println("Front after dequeue: "+queue.front()); System.out.println("Dequeued: "+queue.dequeue()); System.out.println("Front after dequeue: "+queue.front()); //trying to dequeue empty queue System.out.println("Dequeued: "+queue.dequeue()); //checking queue status after removing of elements System.out.println("Queue after dequeuing 5 elements"); System.out.println("Is queue empty: "+queue.isEmpty()); System.out.println("Is queue full: "+queue.isFull()); System.out.println("Size of queue: "+queue.size()); System.out.println("Front of queue: "+queue.front()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
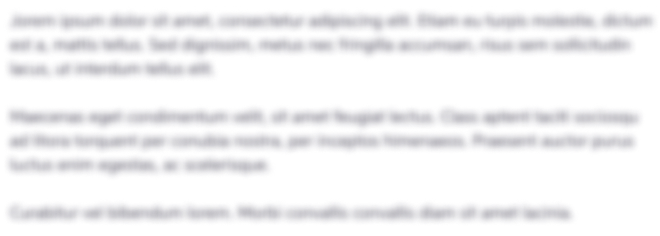
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started