Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Part C: The Tile Type [35% = 31% test program + 4% code] In Part C, you will create a structured record (struct) to represent
Part C: The Tile Type [35% = 31% test program + 4% code] In Part C, you will create a structured record (struct) to represent a tile, as well as related constants and functions. You will have an interface file named Tile.h and an implementation file named Tile.cpp. Each tile will have unsigned integer member fields for the tile owner, genus, and species. We will use a special value to indicate that a tile has no owner. Tile will be an abstract data type (ADT), so client code will only operate on Tile variables using the functions we provide. By the end of Part C, you will have functions with the following prototypes: Tile tileCreate (unsigned int genus1, unsigned int species1); bool tileIsOwner (const Tile & tile); unsigned int tileGetAffectedPlayer (const Tile& tile, unsigned int whose_turn); unsigned int tileGetGenus (const Tile & tile); unsigned int tileGetSpecies (const Tile & tile); void tileSetOwner (Tile& tile, unsigned int owner1); void tileActivate (const Tile & tile, unsigned int whose_turn); void tilePrint (const Tile& tile); void tilePrint OwnerChar (const Tile & tile); void tilePrintGenusChar (const Perform the following steps: 1. In Tile.h, define a record to represent a tile. 2. In Tile.h, define unsigned integer constants for the different kinds of tile genus. We will create all the constants for the whole CS 115 course now so that we never have to change them. The constants are GENUS_COUNT, GENUS_MONEY, GENUS_DICE, GENUS_POINTS, and GENUS-BOMB, and they have the respective values 4, 0, 1, 2, and 3. 3. Copy in the function prototypes shown above. 4. In Tile.cpp, define unsigned integer constant named NO_OWNER to indicate that a tile has no owner. It should have a very large value so that there is no danger of a player having that number. 5. Add an implementation for the tileCreate function. First, it should create a local variable of the Tile type. Next, it should set the genus and species of the tile variable based on the parameters. Then, it should set the tile owner to NO_OWNER. Finally, the function should return the tile. 6. Add an implementation for the tile Is Owner function that returns false if the tile owner is NO_OWNER and true otherwise. 7. Add implementations for the tileSetOwner function that sets the tile owner to owner1. Note: The Tile parameter for this function is not const. If it was, we wouldn't be able to change the owner. 8. Add implementations for the tileGetGenus and tileGet Species functions. They should return the values of the appropriate fields of the Tile parameter. Note: These functions will not be used by the game, but they will be used by the test programs. 9. Add implementations for the tileGetAffectedPlayer function. If the tile has an owner, it should return the owner. Otherwise, it should return whose_turn. 0. Add an implementation for the tilePrint OwnerChar function. If the tile has no owner, it should print a space (' '). Otherwise, it should call the playerGet TileChar function and print the value that function returns. 1. Add an implementation for the tilePrintGenusChar function. If the tile genus is GENUS_MONEY, it should print a dollar sign ('$'). If the tile genus is GENUS_POINTS, it should print a star ('*'). Otherwise, it doesn't need to do anything. Note: We will print things for the other kinds of genus in later assignments. 2. Add an implementation for the tilePrint SpeciesChar function. It should just print the tile species as a number. 3. Add an implementation for the tilePrint function to display three characters representing a tile. It should call the tilePrint*Char functions. Hint: Remember * means "anything". In this case, there are three tilePrint*Char functions. 4. Add an implementation for the tileActivate function to handle what happens when a tile is selected by a dice roll. It should start by determining which player is affected. Then it should check the tile genus. If the tile is a money tile, increase the affected player's money by an amount equal to the tile's species. If the tile is a points tile, increase that player's points. Hint: Call a Tile function to determine the affected player. Hint: Call a Player function to increase a player's money or points. 5. Add a precondition to the tileCreate function. It should require that the genus parameter is strictly less than GENUS_COUNT. 6. Test your Tile module using the TestTile2.cpp program provided. You will need the TestHelper.h and TestHelper.cpp, files. #include "Tile.h" #include "Tile.h" #include errors // repeated to test for #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
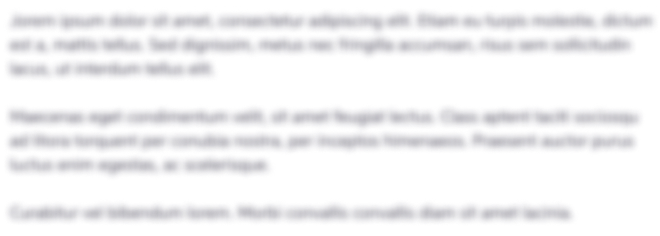
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started