Question
Part C: Write Java Code for the following case scenarios: Design a class named Employee. The class should keep the following information in fields: Employee
Part C: Write Java Code for the following case scenarios:
Design a class named Employee. The class should keep the following information in fields:
Employee name
Employee number in the format XXX-L, where each X is a digit within the range 0-9 and the L is a letter within the range A_M.
Hire date
Write one or more constructors and the appropriate accessor and mutator methods for the class.
Next, write a class named ProductionWorker that extends the Employee class. The ProductionWorker class should have fields to hold the following information:
Shift (an integer)
Hourly pay rate (double)
The workday is divided into two shifts: day and night. The shift field will be an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write one or more constructors and the appropriate accessor and mutator methods for the class. Demonstarte the classes by writing a program that uses a ProductionWorker object.
In a particular factory, a team leader is an hourly paid production worker that leads a small team. In addition to hourly pay, team leaders earn a fixed monthly bonus. Team leaders are required to attend a minimum number of hours of training per year. Design a TeamLeader class that extends the ProductionWorker class you designed in the previous question. The TeamLeader class should have fields for the monthly bonus amount, the required number of training hours, and the number of training hours that the team leader has attended. Write one or more constructors and the appropriate accesors and mutator methods for the class. Demonstrate the class by writing a program that uses a TeamLeader object
3. Identify the output of the following Java code. Justify the syntax.
File Name:CompSciStudentDemo.java
/**
This program demonstrates the CompSciStudent class.
*/
public class CompSciStudentDemo
{
public static void main(String[] args)
{
// Create a CompSciStudent object.
CompSciStudent csStudent =
new CompSciStudent("Jennifer Haynes",
"167W98337", 2015);
// Store values for math, CS, and gen ed hours.
csStudent.setMathHours(12);
csStudent.setCsHours(20);
csStudent.setGenEdHours(40);
// Display the student's data.
System.out.println(csStudent);
// Display the number of remaining hours.
System.out.println("Hours remaining: " +
csStudent.getRemainingHours());
}
}
File Name:CompSciStudent.java
/**
This class holds data for a computer science student.
*/
public class CompSciStudent extends Student
{
// Required hours
private final int MATH_HOURS = 20; // Math hours
private final int CS_HOURS = 40; // Comp sci hours
private final int GEN_ED_HOURS = 60; // Gen ed hours
// Hours taken
private int mathHours; // Math hours taken
private int csHours; // Comp sci hours taken
private int genEdHours; // General ed hours taken
/**
The Constructor sets the student's name,
ID number, and the year admitted.
@param n The student's name.
@param id The student's ID number.
@param year The year the student was admitted.
*/
public CompSciStudent(String n, String id, int year)
{
super(n, id, year);
}
/**
The setMathHours method sets the number of
math hours taken.
@param math The math hours taken.
*/
public void setMathHours(int math)
{
mathHours = math;
}
/**
The setCsHours method sets the number of
computer science hours taken.
@param cs The computer science hours taken.
*/
public void setCsHours(int cs)
{
csHours = cs;
}
/**
The setGenEdHours method sets the number of
general ed hours taken.
@param genEd The general ed hours taken.
*/
public void setGenEdHours(int genEd)
{
genEdHours = genEd;
}
/**
The getRemainingHours method returns the
the number of hours remaining to be taken.
@return The hours remaining for the student.
*/
@Override
public int getRemainingHours()
{
int reqHours, // Total required hours
remainingHours; // Remaining hours
// Calculate the required hours.
reqHours = MATH_HOURS + CS_HOURS + GEN_ED_HOURS;
// Calculate the remaining hours.
remainingHours = reqHours - (mathHours + csHours
+ genEdHours);
return remainingHours;
}
/**
The toString method returns a string containing
the student's data.
@return A reference to a String.
*/
@Override
public String toString()
{
String str;
str = super.toString() +
" Major: Computer Science" +
" Math Hours Taken: " + mathHours +
" Computer Science Hours Taken: " + csHours +
" General Ed Hours Taken: " + genEdHours;
return str;
}
}
File Name:Student.java
/**
The Student class is an abstract class that holds
general data about a student. Classes representing
specific types of students should inherit from
this class.
*/
public abstract class Student
{
private String name; // Student name
private String idNumber; // Student ID
private int yearAdmitted; // Year admitted
/**
The Constructor sets the student's name,
ID number, and year admitted.
@param n The student's name.
@param id The student's ID number.
@param year The year the student was admitted.
*/
public Student(String n, String id, int year)
{
name = n;
idNumber = id;
yearAdmitted = year;
}
/**
The toString method returns a String containing
the student's data.
@return A reference to a String.
*/
public String toString()
{
String str;
str = "Name: " + name
+ " ID Number: " + idNumber
+ " Year Admitted: " + yearAdmitted;
return str;
}
/**
The getRemainingHours method is abstract.
It must be overridden in a subclass.
@return The hours remaining for the student.
*/
public abstract int getRemainingHours();
}
Homework:
Write a Java code for a class named SportsVenuePass. This class will be used to instantiate objects that represent the right to access a performance in the sports venue. The class will have 2 private instance variables: a boolean variable named used and an integer variable named passId. The boolean variable used will be initialised to false and changed to true by the method useThisPass() which will be called when the pass is presented at the sports ground. When the pass is created, a unique new id number is allocated to passId. This id number will be generated by adding one to the previously used passId number, which is kept stored in a variable shared by all SportsVenuePass objects
Step by Step Solution
There are 3 Steps involved in it
Step: 1
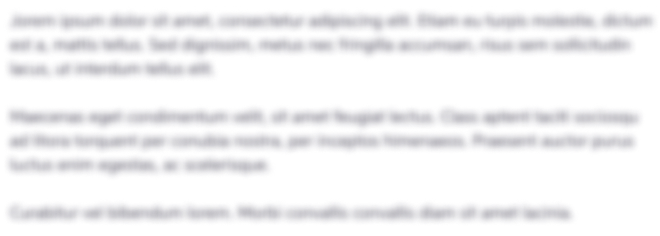
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started