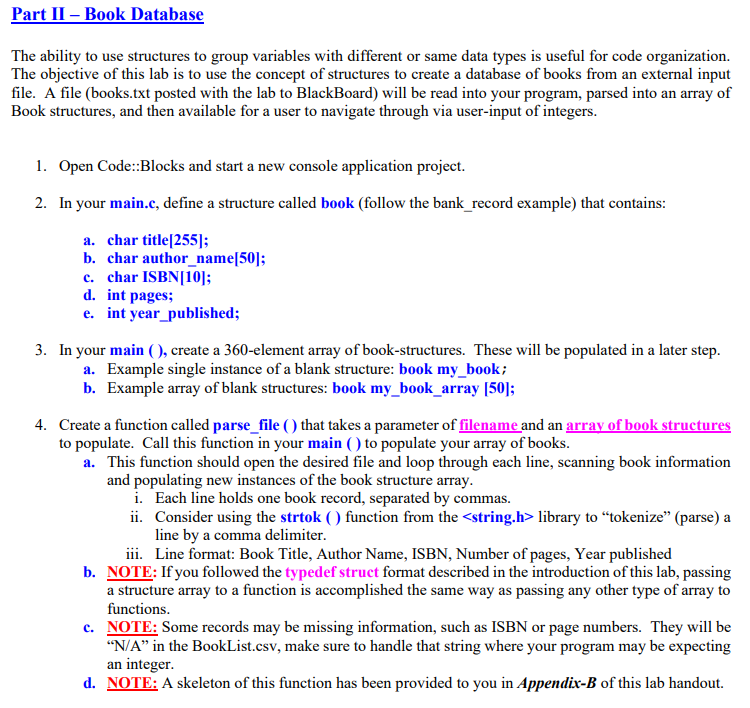
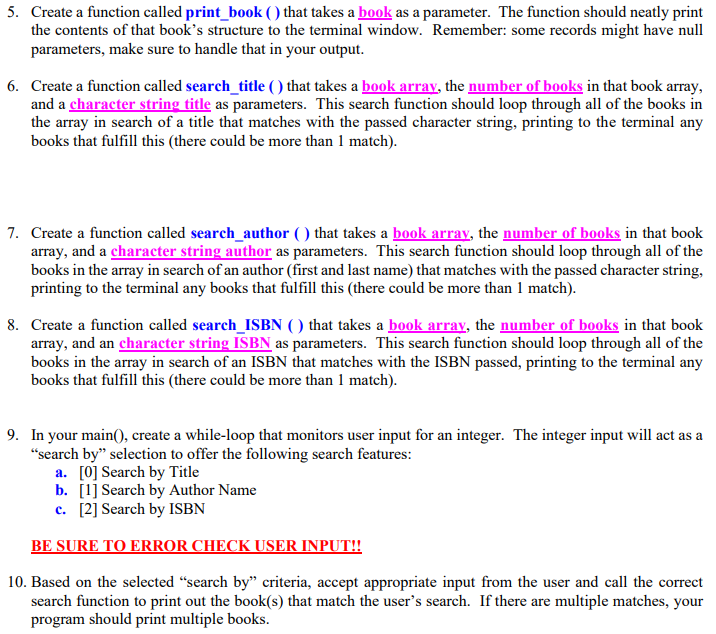
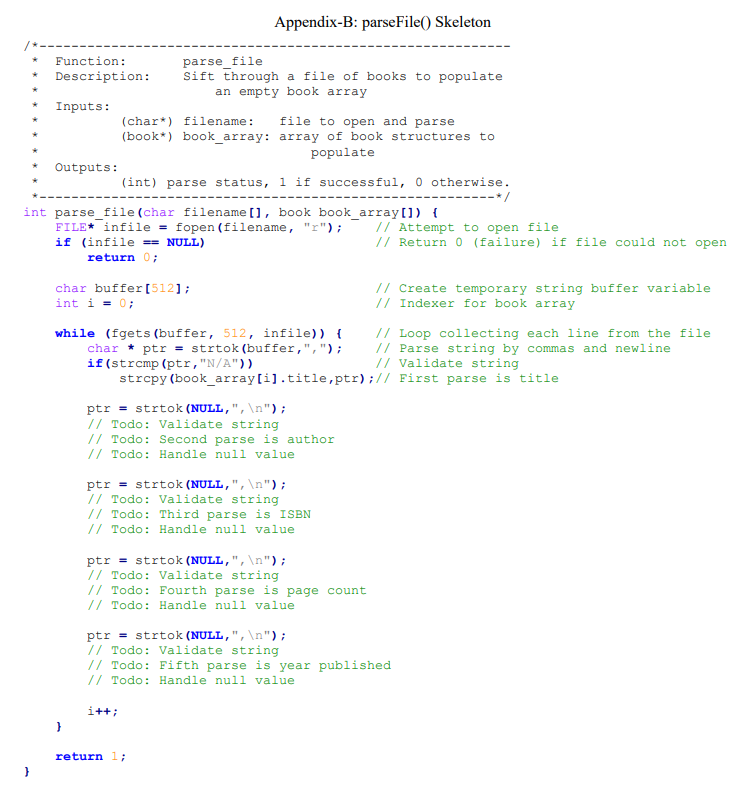
Part II Book Database The ability to use structures to group variables with different or same data types is useful for code organization. The objective of this lab is to use the concept of structures to create a database of books from an external input file. A file (books.txt posted with the lab to BlackBoard) will be read into your program, parsed into an array of Book structures, and then available for a user to navigate through via user-input of integers. 1. Open Code::Blocks and start a new console application project. 2. In your main.c, define a structure called book (follow the bank_record example) that contains: a. char title[255]; b. char author_name[50]; c. char ISBN[10]; d. int pages; e. int year_published; 3. In your main (), create a 360-element array of book-structures. These will be populated in a later step. a. Example single instance of a blank structure: book my_book; b. Example array of blank structures: book my_book_array [50]; 4. Create a function called parse_file() that takes a parameter of filename and an array of book structures to populate. Call this function in your main () to populate your array of books. a. This function should open the desired file and loop through each line, scanning book information and populating new instances of the book structure array. i. Each line holds one book record, separated by commas. ii. Consider using the strtok () function from the
library to tokenize (parse) a line by a comma delimiter. iii. Line format: Book Title, Author Name, ISBN, Number of pages, Year published b. NOTE: If you followed the typedef struct format described in the introduction of this lab, passing a structure array to a function is accomplished the same way as passing any other type of array to functions. c. NOTE: Some records may be missing information, such as ISBN or page numbers. They will be "N/A in the BookList.csv, make sure to handle that string where your program may be expecting an integer. d. NOTE: A skeleton of this function has been provided to you in Appendix-B of this lab handout. 5. Create a function called print_book () that takes a book as a parameter. The function should neatly print the contents of that book's structure to the terminal window. Remember: some records might have null parameters, make sure to handle that in your output. 6. Create a function called search_title() that takes a book array, the number of books in that book array, and a character string title as parameters. This search function should loop through all of the books in the array in search of a title that matches with the passed character string, printing to the terminal any books that fulfill this there could be more than 1 match). 7. Create a function called search_author (that takes a book array, the number of books in that book array, and a character string author as parameters. This search function should loop through all of the books in the array in search of an author (first and last name) that matches with the passed character string, printing to the terminal any books that fulfill this there could be more than 1 match). 8. Create a function called search_ISBN () that takes a book array, the number of books in that book array, and an character string ISBN as parameters. This search function should loop through all of the books in the array in search of an ISBN that matches with the ISBN passed, printing to the terminal any books that fulfill this there could be more than 1 match). 9. In your main(), create a while-loop that monitors user input for an integer. The integer input will act as a "search by selection to offer the following search features: a. [0] Search by Title b. [1] Search by Author Name c. [2] Search by ISBN BE SURE TO ERROR CHECK USER INPUT!! 10. Based on the selected search by criteria, accept appropriate input from the user and call the correct search function to print out the book(s) that match the user's search. If there are multiple matches, your program should print multiple books. Appendix-B: parseFile() Skeleton Function: parse file Description: Sift through a file of books to populate an empty book array Inputs: (char*) filename: file to open and parse (book*) book_array: array of book structures to populate Outputs: (int) parse status, i if successful, 0 otherwise. int parse_file(char filename[], book book_array[]) { FILE* infile = fopen(filename, // Attempt to open file if (infile == NULL) // Return 0 (failure) if file could not open return 0; char buffer[512]; int i = 0; // Create temporary string buffer variable // Indexer for book array while (fgets (buffer, 512, infile)) { // Loop collecting each line from the file char * ptr = strtok (buffer,", "); // Parse string by commas and newline if (strcmp (ptr,"N/A")) // Validate string strcpy(book_array[i].title,ptr);// First parse is title ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Second parse is author // Todo: Handle null value ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Third parse is ISBN // Todo: Handle null value ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Fourth parse is page count // Todo: Handle null value ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Fifth parse is year published // Todo: Handle null value i++; } return 1; } Part II Book Database The ability to use structures to group variables with different or same data types is useful for code organization. The objective of this lab is to use the concept of structures to create a database of books from an external input file. A file (books.txt posted with the lab to BlackBoard) will be read into your program, parsed into an array of Book structures, and then available for a user to navigate through via user-input of integers. 1. Open Code::Blocks and start a new console application project. 2. In your main.c, define a structure called book (follow the bank_record example) that contains: a. char title[255]; b. char author_name[50]; c. char ISBN[10]; d. int pages; e. int year_published; 3. In your main (), create a 360-element array of book-structures. These will be populated in a later step. a. Example single instance of a blank structure: book my_book; b. Example array of blank structures: book my_book_array [50]; 4. Create a function called parse_file() that takes a parameter of filename and an array of book structures to populate. Call this function in your main () to populate your array of books. a. This function should open the desired file and loop through each line, scanning book information and populating new instances of the book structure array. i. Each line holds one book record, separated by commas. ii. Consider using the strtok () function from the library to tokenize (parse) a line by a comma delimiter. iii. Line format: Book Title, Author Name, ISBN, Number of pages, Year published b. NOTE: If you followed the typedef struct format described in the introduction of this lab, passing a structure array to a function is accomplished the same way as passing any other type of array to functions. c. NOTE: Some records may be missing information, such as ISBN or page numbers. They will be "N/A in the BookList.csv, make sure to handle that string where your program may be expecting an integer. d. NOTE: A skeleton of this function has been provided to you in Appendix-B of this lab handout. 5. Create a function called print_book () that takes a book as a parameter. The function should neatly print the contents of that book's structure to the terminal window. Remember: some records might have null parameters, make sure to handle that in your output. 6. Create a function called search_title() that takes a book array, the number of books in that book array, and a character string title as parameters. This search function should loop through all of the books in the array in search of a title that matches with the passed character string, printing to the terminal any books that fulfill this there could be more than 1 match). 7. Create a function called search_author (that takes a book array, the number of books in that book array, and a character string author as parameters. This search function should loop through all of the books in the array in search of an author (first and last name) that matches with the passed character string, printing to the terminal any books that fulfill this there could be more than 1 match). 8. Create a function called search_ISBN () that takes a book array, the number of books in that book array, and an character string ISBN as parameters. This search function should loop through all of the books in the array in search of an ISBN that matches with the ISBN passed, printing to the terminal any books that fulfill this there could be more than 1 match). 9. In your main(), create a while-loop that monitors user input for an integer. The integer input will act as a "search by selection to offer the following search features: a. [0] Search by Title b. [1] Search by Author Name c. [2] Search by ISBN BE SURE TO ERROR CHECK USER INPUT!! 10. Based on the selected search by criteria, accept appropriate input from the user and call the correct search function to print out the book(s) that match the user's search. If there are multiple matches, your program should print multiple books. Appendix-B: parseFile() Skeleton Function: parse file Description: Sift through a file of books to populate an empty book array Inputs: (char*) filename: file to open and parse (book*) book_array: array of book structures to populate Outputs: (int) parse status, i if successful, 0 otherwise. int parse_file(char filename[], book book_array[]) { FILE* infile = fopen(filename, // Attempt to open file if (infile == NULL) // Return 0 (failure) if file could not open return 0; char buffer[512]; int i = 0; // Create temporary string buffer variable // Indexer for book array while (fgets (buffer, 512, infile)) { // Loop collecting each line from the file char * ptr = strtok (buffer,", "); // Parse string by commas and newline if (strcmp (ptr,"N/A")) // Validate string strcpy(book_array[i].title,ptr);// First parse is title ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Second parse is author // Todo: Handle null value ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Third parse is ISBN // Todo: Handle null value ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Fourth parse is page count // Todo: Handle null value ptr = strtok (NULL,", "); // Todo: Validate string // Todo: Fifth parse is year published // Todo: Handle null value i++; } return 1; }