Question
Part II: PA3: Car Dealership (40 points) For Programming Assignment 3 you will be creating a program to manage cars in a dealership. This will
Part II:
PA3: Car Dealership (40 points)
For Programming Assignment 3 you will be creating a program to
manage cars in a dealership.
This will again be a menu driven system.
You will make a
vector
of Dealers (there can be zero to
many dealers). Each dealer can have zero to many cars on the lot, represented not by a vector,
but by a
dynamic array
.
The following is your menu:
1.
Read
Dealers and Cars
from file
2.
Displ
ay Dealers
3.
Choose a Dealer Number, D
isplay Cars
4.
Choose a Dealer Number, Add Car
5.
Choose a Dealer Number, List Cars and Modify a Car
6.
Choose a Dealer, Sort cars
by VIN
(EXTRA CREDIT)
7.
Write Dealers and Cars
to file
(EXTRA CREDIT)
8.
E
xit
Your program will be
object oriented
(using classes
, not stru
cts
)
with the following classes
defined in Dealer.h and Car.h
Page
2
of
7
Dealer
.h
#ifndef _DEALER
#define _DEALER
c
lass Dealer {
private:
Dealer ( )
// Dont forget to add a new car pointer when you use the Dealer default / constructor
Dealer (
string _dealer
Name
,
int _dealerN
umber)
;
string
d
ealerName
;
int
d
ealer
Number
;
int
numberOfCars
;
public:
Car *carArrayPtr;
//
pointer to a Dynamic Car Array (Note: make sure this is set to nullptr
in the
constructors
)
. T
his would normally be a private, but this takes away a
little complexity)
void
set
Dealer
Name
(
string _
dealerName
)
;
void
set
DealerNumber
(
string
_dealerNumber
)
;
void
setNumberOfCars ( int _numberOfCars)
;
string
get
Dealer
Name
( )
;
int
getDealerNumber
( )
;
int
getNumberOfCars
( );
+ friend
ostream &
operator <<
(
ostream &
out
, Dealer
_dealer
)
;
//Print the Dealer Name and Number and Blank line for a specific dealer.
}
#endif //_DEALER
Page
3
of
7
Car
.h
class Car
{
#ifndef _CAR
#define _CAR
private:
string vin;
string
make
;
string
model
;
int
year
;
doubler
price
;
public:
Car
( )
;
Car
(string _vin
,
string _make
,
string m
odel,
int y
ear,
double p
rice)
;
+ getVIN( ):string
+ getMake( ):string
+ getModel( ):string
+ getYear( ):int
+ getPrice( ):double
+ setVIN(_VIN
:string):void
+ set
Make(_make
:string):void
+ setMode
l(_
model
:string):void
+ setYear(_year
:int):void
+ setPrice(_price
:double):void
+ friend operator <<
(out: ostream &,
Car
:
_
car
):ostream &
//Print the VIN, Make, Model, Year, Price and Blank line for a specific car.
#endif
Page
4
of
7
You will have four files for your program (Use these file names!): main.cpp, functions.h,
Dealer.h, Dealer
.cpp. Place into a file folder named LastnamePA3, the
n
zip the
content and hand
in a zip file to canvas
main.cpp: this will be your driver file
.
functions.
h: this will contain your
functi
ons for each of the menu items
.
Dealer
.h: this will contain the class declarations for
car
and dealer
including the operator
<<
.
Dealer
.cpp: this will contain the class implementations for
car
and dealer.
Remember,
you do not #include Dealer.cpp, just #include functions.h and #include
Dealer.h
You will be storing your
dealer
objects in a
vector
. In the main function, you will create a vector
of
Dealers
. When the menu
is
called, you will have to check your vector and ensure that
it is not
empty
(size == 0)
before referring to the index number
of the vector.
Your Dealer vector points
to a
dynamic array
of
car
s
(remember dynamic arrays use the
keyword new)
.
W
hen you access
the
car
s class you should verify that it is not set to nullptr
before accessing it
.
You
may not use
any global variables
, so must pass vectors and arrays into parameters. If you
want to change a vector you must pass by reference (&). If you have arrays, y
ou must pass size
and the array, but arrays are already pointers, so do not use & when passing an array
.
Each menu item will have a corresponding function, and the definition of the function will be
found in the file functions.h.
Also, your operator << fun
ction for Dealer and Car will be
implemented in your functions.h.
All input/output
will be done in the functions and not in main
(ex
cept
asking for what menu option the user wants).
The following are the details for each of your menu options:
1.
Read
Dealers and Cars
from file
2.
Displ
ay Dealers
3.
Choose a Dealer Number, Display Car
s
4.
Choose a Dealer Number, Add Car
5.
Choose a Dealer Number, List Cars and Modify a Car
6.
Choose a Dealer, Sort cars
by VIN
(EXTRA CREDIT)
7.
Write Dealers and Cars
to file
(EXTRA CREDIT
)
8.
E
xit
1.
Pass the vector of dealers (from main) into the function by reference.
Each
Dealer will
have a name and number followed by the number of cars in the dealership (on separate
lines). Then you will read in a Vin, Make, Model, Year and Price for each car (on
separate lines). You can assume that the number of cars as well as the Vin
, Make,
Page
5
of
7
Model, Year and Price will be complete for each car. Each dealer can have
Your file
format will be as follows
:
Dealer Name
Dealer Number
2
Car1 Vin
Car1 Make
Car1 Model
Car1 Year
Car1 Price
Car2 Vin
Car2 Make
Car2 Model
Car2 Year
Car2 Price
Dealer
Name
Dealer Number
1
Car1 Vin
Car1 Make
Car1 Model
Car1 Year
Car1 Price
Dont forget, between << and a getline you must use a .ignore( ) (e.g. infile.ignore( ) )
Page
6
of
7
Here is an example
in.txt
file
(You MUST read in this file name)
:
John Elway Dodge
1
23
2
VI
N123
Dodge
Ram 1500 Quad Cab
2017
45186.00
VIN456
Chevrolet
Traverse Premier SUV
2017
47868.00
Tesla Cherry Creek
234
1
VIN789
Tesla
Model X
2
017
105200
2.
Pass the vector of dealers (from main) into the function by reference.
Display the Dealer Name
and
Number for each dealer (put a blank line between dealers)
. Use the << operator with cout
(cout << dealer[x];)
3.
Pass the vector of dealers (from main) into the function by reference.
Display the
Dealer
Names and Numbers (menu #2). Ask the user for the de
aler number, display all of the cars
by
using a for loop (from zero to size of the car array)
using the << operator with cout
( cout <<
dealer[x].
carArrayPtr[x];)
4.
Pass the vector of dealers (from main) into the function by reference.
a)
D
isplay the Dea
ler
Names and Numbers (menu #2).
b
)
Ask the user for
the dealer number then the new
car
information
.
Follow the steps found in CSCI 1411 Lab 09 to increase the dynamic array
.
(Essentially you will make a new array, one bigger than
the previous car array.
Then you will
copy everything over from the old car array to the new one. Then you will point the car pointer
to the new array)
You can earn
up to
20%
extra credit
(48/40)
for completing 5 and 6 as long as you get at least
80% on the required tasks.
5.
Pass the vector of dealers (from main) into the function by reference.
D
isplay the Dealer
Names and Numbers (menu #2).
6.
Ask the user for the dealer number. Sort the cars by vin and
display them (with blank lines between each car
)
Page
7
of
7
6.
Pass the vector of de
alers (from main) into the function by reference. Also pass in the
filestream (from main) by reference
. Call the output file out.txt
.
Write all of the Dealers and
Cars to a
separate
output file
in the same format as in item #1
. This should include all ch
anges to
the vector and dynamic array (including add, delete, modify, sorting etc.)
7
.
Exit out of the program
What to Hand
-
In:
Submit to canvas main.cpp, functions.h, Dealer.h and Dealer.cpp and an in.txt files. Each must be
complete with your name on i
t. If you complete the extra credit, you must have 7 menu items and this
must be documented in your main.cpp.
Note: You must document ALL code that you took from ANY source (including the internet, your book,
someone else). Failure to do so may result in a
grade of zero for EACH student
with too similar code
.
You can work together with big ideas, but all code MUST be your own.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
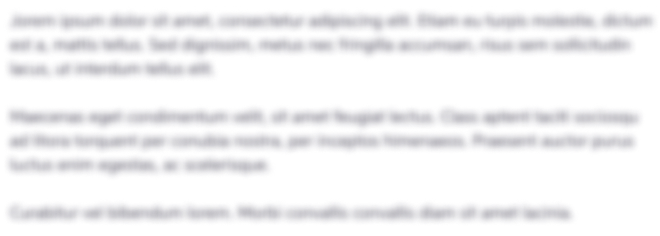
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started