Question
*Person code* /** * ITEC 3150 - Text File I/O Example Person Class - This is the object we will * read and write to
*Person code*
/** * ITEC 3150 - Text File I/O Example Person Class - This is the object we will * read and write to file public class Person { public String firstName; public String lastName; public int idNum; public String city;
/** * Default constructor used to create empty attributes */ public Person() { firstName = ""; lastName = ""; idNum =0; city =""; }
/** * @param firstName * @param lastName * @param idNum * @param city */ public Person(String firstName, String lastName, int idNum, String city) { this.firstName = firstName; this.lastName = lastName; this.idNum = idNum; this.city = city; }
/* (non-Javadoc) * @see java.lang.Object#toString() */ @Override public String toString() { return "Person [firstName=" + firstName + ", lastName=" + lastName + ", idNum=" + idNum + ", city=" + city + "]"; }
/** * @return the firstName */ public String getFirstName() { return firstName; }
/** * @param firstName the firstName to set */ public void setFirstName(String firstName) { this.firstName = firstName; }
/** * @return the lastName */ public String getLastName() { return lastName; }
/** * @param lastName the lastName to set */ public void setLastName(String lastName) { this.lastName = lastName; }
/** * @return the idNum */ public int getIdNum() { return idNum; }
/** * @param idNum the idNum to set */ public void setIdNum(int idNum) { this.idNum = idNum; }
/** * @return the city */ public String getCity() { return city; }
/** * @param city the city to set */ public void setCity(String city) { this.city = city; }
} /////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
*Starter code*
import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.io.PrintWriter; import java.util.ArrayList; import java.util.Scanner;
public class ReadPeopleFromFile {
/** * @param args */ public static void main(String[] args) { ArrayList
/* * TODO 1: * Create a scanner for reading from a file "peopleFile.txt" that * contains the information for persons */ File peopleFile = new File("peopleFile.txt"); Scanner peopleReader = null; try { peopleReader = new Scanner(peopleFile); } catch (FileNotFoundException e) { System.out.println("File not found - terminating program"); System.exit(0); } /* TODO 2: * Use the scanner to read one person at a time. For each person, * create a Person object and add the object to the array list people. */ while (peopleReader.hasNext()) { String firstName = peopleReader.next(); String lastName = peopleReader.next(); String city = peopleReader.next(); int id = peopleReader.nextInt(); Person p = new Person(firstName, lastName, id, city); people.add(p); } // print info to user System.out.println("The people from the file are:"); System.out.println(people);
/* * TODO 3: * Create a printer writer that will save the persons' info to "secondPeople.txt" */ File secondPeopleFile = new File("secondPeople.txt"); PrintWriter out = null; try { out = new PrintWriter("secondPeople.txt"); } catch (FileNotFoundException e) { System.out.println("unable to open file to write to program ending"); System.exit(0); } /* TODO 4: * Use a for loop to write the persons' info to the file in the same format * as in input file "peopleFile.txt". */ for (Person p: people) { out.println(p.getFirstName() + " " + p.getLastName()+ " " + p.getCity() + " " + p.getIdNum()); } /* * TODO 5: close the print writer */ out.close(); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
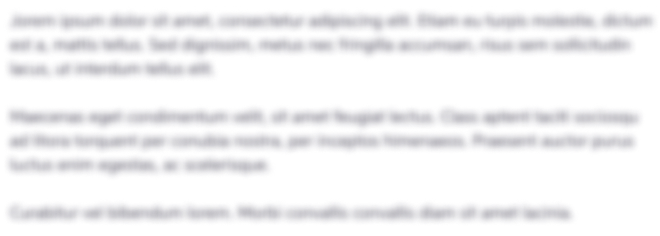
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started