Question
Person.java>> import java.util.Calendar; public class Person { //Attributes are declared below int id; String title,firstName,lastName; Calendar birthDate; //No-arg constructor initializes the attributes to some default
Person.java>>
import java.util.Calendar; public class Person { //Attributes are declared below int id; String title,firstName,lastName; Calendar birthDate; //No-arg constructor initializes the attributes to some default value Person() { this.id=0; this.title=""; this.firstName=""; this.lastName=""; this.birthDate=Calendar.getInstance(); } //This parameterized constructor takes 5 arguments corresponding to its attributes and initializes attributes accordingly Person(int id, String title, String firstName, String lastName, Calendar birthDate) { this.id=id; this.title=title; this.firstName=firstName; this.lastName=lastName; this.birthDate=birthDate; } //Mutator methods are given below void setId(int id) { this.id=id; } void setTitle(String title) { this.title=title; } void setFirstName(String firstName) { this.firstName=firstName; } void setLastName(String lastName) { this.lastName=lastName; } void setBirthDate(Calendar birthDate) { this.birthDate=birthDate; } //Accessor methods are given below int getId() { return this.id; } String getTitle() { return this.title; } String getFirstName() { return this.firstName; } String getLastName() { return this.lastName; } Calendar getBirthDate() { return this.birthDate; } //Overridden toString() method is given below which returns a string containing all attributes in readable form //Note how we are using birthDate attribute - We are using get() to get parts of date public String toString() { return "id: "+this.id+" | title: "+this.title+" | firstName: "+this.firstName+" | lastName: "+this.lastName+" | birthDate: "+this.birthDate.get(Calendar.DAY_OF_MONTH)+"-"+(this.birthDate.get(Calendar.MONTH)+1)+"-"+this.birthDate.get(Calendar.YEAR); } //displayName() method returns the name of Person with title in the proper form String displayName() { return this.title+" "+this.firstName+" "+this.lastName; } }
Faculty>>
import java.util.ArrayList; import java.util.Calendar; //Class Faculty extends Person so attributes/methods of Person are inherited by Faculty public class Faculty extends Person { //Additional Attribute declared ArrayList degree; /o-arg constructor initializes attributes to default values. It calls parent class's no-arg constructor to initialize inherited attributes Faculty() { super(); this.degree=new ArrayList(); } //This parameterized constructor takes 5 arguments corresponding to inherited attribute. It calls the parameterized constructor of parent class to initialize them accordingly //Faculty's own attribute is initialized to default value Faculty(int id, String title, String firstName, String lastName, Calendar birthDate) { super(id,title,firstName,lastName,birthDate); this.degree=new ArrayList(); } //This parameterized constructor takes 6 arguments corresponding to all attributes. It calls the parameterized constructor of parent class to initialize inherited ones //Faculty's own attribute is initialized according to the corresponding argument value Faculty(int id, String title, String firstName, String lastName, Calendar birthDate, ArrayList degree) { super(id,title,firstName,lastName,birthDate); this.degree=degree; } //Mutator And Accessor for degree attribute void setDegree(ArrayList degree) { this.degree=degree; } ArrayList getDegree() { return this.degree; } //Overridden toString() method is given below which returns a string containing all attributes in readable form //Note how we use toString() of super class to rest inherited attributes directly //We use for-each loop to concatenate all values of degree to output string public String toString() { String str = super.toString()+" | degree: "; for(String i : this.degree) { str=str+i+" "; } return str; } //displayName() method returns the name of Person with title in the proper form String displayName() { return this.title+" "+this.firstName+" "+this.lastName+", "+this.degree.get(0); } }
Student.java>>
import java.util.Calendar;
//Class Student extends Person so attributes/methods of Person are inherited by Student public class Student extends Person { //Additional Attribute declared String classification; /o-arg constructor initializes attributes to default values. It calls parent class's no-arg constructor to initialize inherited attributes Student() { super(); this.classification=""; } //This parameterized constructor takes 5 arguments corresponding to inherited attribute. It calls the parameterized constructor of parent class to initialize them accordingly //Student's own attribute is initialized to default value Student(int id, String title, String firstName, String lastName, Calendar birthDate) { super(id,title,firstName,lastName,birthDate); this.classification=""; } //This parameterized constructor takes 6 arguments corresponding to all attributes. It calls the parameterized constructor of parent class to initialize inherited ones //Student's own attribute is initialized according to the corresponding argument value Student(int id, String title, String firstName, String lastName, Calendar birthDate, String classification) { super(id,title,firstName,lastName,birthDate); this.classification=classification; } //Mutator And Accessor for Classification attribute void setClassification(String classification) { this.classification=classification; } String getClassification() { return this.classification; } //Overridden toString() method is given below which returns a string containing all attributes in readable form //Note how we use toString() of super class to rest inherited attributes directly public String toString() { return super.toString()+" | classification: "+this.classification; } }
Lab2.java
public class AddPersonGUI extends Application { TextField txtId,txtFirstName,txtLastName,txtDegree1, txtDegree2,txtDegree3,txtDegree4,txtDegree5; Label lblId,lblTitle,lblFirstName,lblLastName,lblBirtDate, lblType,lblClass,lblDegrees; Button btnSubmit,btnReset; ToggleGroup btnGroup; RadioButton rdfaculty,rdStudent; DatePicker birthDatePicker; ComboBox comboBoxID,comboBoxClass;
@Override public void start(Stage primaryStage) throws Exception { ObservableList
//Setting the padding gridPane.setPadding(new Insets(10, 10, 10, 10));
//Setting the vertical and horizontal gaps between the columns gridPane.setVgap(5); gridPane.setHgap(5);
//Setting the Grid alignment gridPane.setAlignment(Pos.TOP_LEFT); //============= lblId = new Label("ID: "); txtId = new TextField(); gridPane.add(lblId,0,0,1,1); gridPane.add(txtId,1,0,1,1);
//========================= lblTitle= new Label("Title: "); comboBoxID= new ComboBox(); comboBoxID.setItems(options); gridPane.add(lblTitle,0,1,1,1); gridPane.add(comboBoxID,1,1,1,1);
//========================== lblFirstName = new Label("FirstName: "); txtFirstName= new TextField(); gridPane.add(lblFirstName,0,2,1,1); gridPane.add(txtFirstName,1,2,1,1); //========================== lblLastName = new Label("LastName: "); txtLastName= new TextField(); gridPane.add(lblLastName,0,3,1,1); gridPane.add(txtLastName,1,3,1,1); //===================================== lblBirtDate = new Label("BirthDate: "); birthDatePicker = new DatePicker(); gridPane.add(lblBirtDate,0,4,1,1); gridPane.add(birthDatePicker,1,4,1,1);
//============================ btnGroup= new ToggleGroup(); lblType = new Label("Type: "); rdfaculty = new RadioButton("Faculty"); rdStudent = new RadioButton("Student"); //add to button group rdStudent.setToggleGroup(btnGroup); rdfaculty.setToggleGroup(btnGroup); gridPane.add(lblType,0,5); gridPane.add(rdfaculty,1,5,2,1); gridPane.add(rdStudent,2,5); //============================= lblClass= new Label("Class: "); comboBoxClass = new ComboBox(); gridPane.add(lblClass,0,6); gridPane.add(comboBoxClass,1,6);
//=====================
lblDegrees = new Label("Degrees: "); txtDegree1 = new TextField(); txtDegree2 = new TextField(); txtDegree3 = new TextField(); txtDegree4 = new TextField(); txtDegree5 = new TextField(); gridPane.add(lblDegrees,0,7); gridPane.add(txtDegree1,1,7); gridPane.add(txtDegree2,1,8); gridPane.add(txtDegree3,1,9); gridPane.add(txtDegree4,1,10); gridPane.add(txtDegree5,1,11); //========================
btnSubmit = new Button("Submit"); btnReset = new Button("Reset"); gridPane.add(btnSubmit,0,12); gridPane.add(btnReset,1,12);
Scene scene = new Scene(gridPane);
primaryStage.setTitle("Person Add"); primaryStage.setHeight(500); primaryStage.setWidth(350); primaryStage.setResizable(false); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
Assignment: Augment the GUI from Lab 2 so you can create the appropriate object from Lab 1. 1) Create a new project Lab3. 2) Copy the Person/Faculty/Student classes from Lab1 and incorporate them in Lab 3. 3) Copy/rename your Lab2 main driver into this project as Lab3.java: a) Add a label/text field for Display Name: b) Add a label/textarea for toString c) Make the Faculty/Student radio button toggle the visibility of the classification and degree GUI components accordingly. (eng. when Student is selected, classification components should be visible and the degree components should *not* be visible) d) A submit button should show validate the contents, and if successful, should create the appropriate object from your previous lab (Student or Faculty), populate the fields with the values of the GUI and reflect the contents of the displayNamel) and toString() methods in separate text boxes within the GUI. While technically you could update the contents of the display name and toString boxes without actually creating a Student/Faculty, the purpose of this lab is to move towards using objects in a real-world fashionStep by Step Solution
There are 3 Steps involved in it
Step: 1
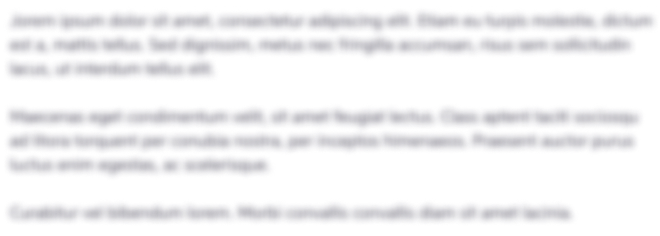
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started