Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/ / Pet.cpp / / 2 a - Lab - 0 6 - Pets / / #include #include #include #include #include Pet.h using namespace
Pet.cpp
aLabPets
#include
#include
#include
#include
#include "Pet.h
using namespace std;
This is a way to properly initialize outofline a static variable.
sizet Pet::population ;
Pet::Petstd::string name, long id int numlimbs : namenameididnumlimbsnumlimbs
population;
Pet::~Pet
population;
string Pet::getname const
return name;
long Pet::getid const
return id;
int Pet::getnumlimbs const
return numlimbs;
bool Pet::setnamestring name
ifname.empty
name name;
return true;
return false;
bool Pet::setidlong id
ifid
id id;
return true;
return false;
bool Pet::setnumlimbsint numlimbs
ifnumlimbs
numlimbs numlimbs;
return true;
return false;
string Pet::tostring const
stringstream ss;
ss Name: name ID: id Limb Count: numlimbs ;
return ssstr;
Fill in the supplied pets vector with n pets whose
properties are chosen randomly.
Don't mess with this method more than necessary.
void Pet::getnpetssizet n std::vector& pets, int namelen
pets.resizen;
long previd ;
for sizet i ; i n; i
long id previd rand;
petsisetidid;
petsisetnumlimbsrand; up to arachnids
string name makeanamenamelen;
petsisetnamename;
previd id;
string Pet::makeanameint len
const string vowels "aeiou";
const string consonants bcdfghjklmnpqrstvwxyz;
string name ;
bool isvowel rand;
bool isconsonants rand;
int i ;
while i len
if isvowel
name vowelsrand vowels.length;
else ifisconsonants
name consonantsrand consonants.length;
isvowel isvowel;
i;
return name;
bool operatorconst Pet& pet const Pet& pet
return petgetname petgetname && petgetid petgetid && petgetnumlimbs petgetnumlimbs;
bool operator!const Pet& pet const Pet& pet
return pet pet;
ostream& operatorostream& os const Pet& pet
os pet.tostring;
return os;
#ifndef Peth
#define Peth
#include
#include
#include
#include
using namespace std;
class Tests;
class Pet
public:
string name;
long id;
int numlimbs;
static sizet population;
Petstring name long id int numlimbs ;
~Pet;
string getname const;
long getid const;
int getnumlimbs const;
bool setnamestring name;
bool setidlong id;
bool setnumlimbsint numlimbs;
string tostring const;
static void getnpetssizet n std::vector& pets, int namelength;
static sizet getpopulation return population;
static string makeanameint len;
;
std::ostream& operatorstd::ostream& os const Pet& pet;
bool operatorconst Pet& pet const Pet& pet;
bool operator!const Pet& pet const Pet& pet;
#endif Peth Test Output
Check failed. I called makeaname:
And got But I expected to get: kuxeyos
You think that's it
&
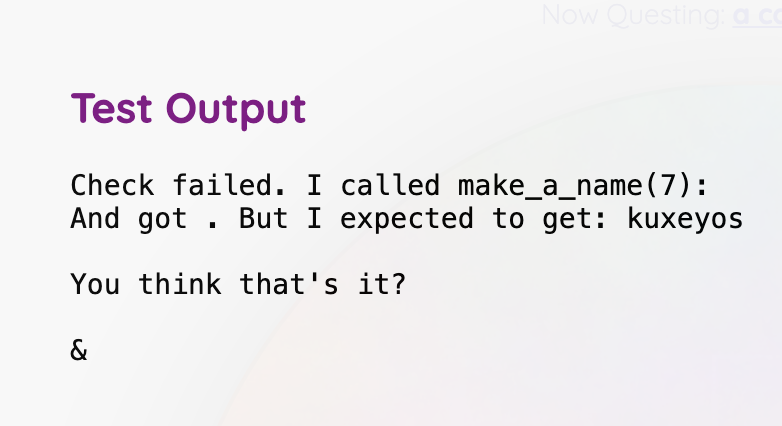
Step by Step Solution
There are 3 Steps involved in it
Step: 1
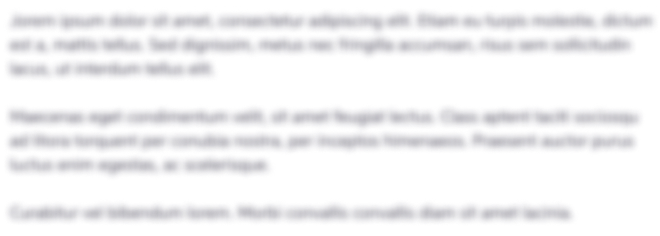
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started