Question
Pete has two accounts with XBank, a savings account and a checking account. The fees on checking account are 10% of the balance, while the
Pete has two accounts with XBank, a savings account and a checking account. The fees on checking account are 10% of the balance, while the interest on the savings account is 10%. Pete will be travelling for a while and wont need either of the accounts, but wants to keep a set amount on the checking account at all time for a case of emergency. At the beginning of his trip he has a deposit of $1000 on the checking account, and a deposit of $1000 on the savings account. To balance the accounts, Pete scheduled a regular transfer of $100 from the savings to the checking account. Pete reasons that the 10% interest on the savings account will cover exactly the fee. Upon his return, Pete finds much to his surprise that his accounts fall short of the expected $2000. He calls customer service of XBank. The customer representative tells him that they cant find any problem with their banking software; they tested it a few times with the amounts Pete provided, and each time the final combined balance was $2000. The banking software completed three tasks, each by a separate thread. The first thread withdraws the fee from the checking account, the second adds the interest to the savings account, and the final thread transfers $100 from the savings to checking account. Question 1 Open the project banking, clean and build it, and run it a few times. Can you reproduce Petes problem? Analyze and describe the problem, and how to solve it. Use the debugger if necessary. Question 2 Use synchronization to solve the banking problem. Implement in the program files provided to you.
Java Code:
Account.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package banking;
public class Account { final String accountHolder; final String accountType; double balance=0; public Account(String name, String type,double credit) { this.accountHolder = name; this.accountType = type; this.balance=credit; } public void deposit(double credit) { balance += credit; } public void withdraw(double credit) { balance -= credit; } public void addinterest(double rate) { balance *= (100+rate)/100.0; } }
Banking.java:
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package banking;
public class Banking {
/** * @param args the command line arguments */ public static void main(String[] args) throws InterruptedException { System.out.println("Application started"); Account savings = new Account("Pete","Super Saver",1000); Account checking = new Account("Pete","Free Checking",1000); System.out.println(" Beginning of month"); System.out.println(savings.accountType + ":\t"+ savings.balance); System.out.println(checking.accountType + ":\t"+ checking.balance); System.out.println("Total before \t"+ (checking.balance+savings.balance)); Interest checkInterest = new Interest(checking, -10); Interest saveInterest = new Interest(savings, 10); Transfer transfer = new Transfer(savings,checking,100); checkInterest.start(); saveInterest.start(); transfer.start(); Thread.sleep(520); System.out.println(" End of month"); System.out.println(savings.accountType + ":\t"+ savings.balance); System.out.println(checking.accountType + ":\t"+ checking.balance); System.out.println("Total after \t"+ (checking.balance+savings.balance)); System.out.println("Main thread finished"); } }
Interest.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package banking;
import java.util.logging.Level; import java.util.logging.Logger;
class Interest extends java.lang.Thread { final Account myAccount; double myRate; public Interest(Account account, double rate) { this.myAccount=account; this.myRate = rate; setName("Interest"); } @Override public void run() { System.out.println("Interest this month on "+ myAccount.accountType + ":\t" + myAccount.balance*myRate/100.0); myAccount.addinterest(myRate); //System.out.println(getName() + " to account " + myAccount.accountType + " successfully applied"); } }
Transfer.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package banking;
import java.util.logging.Level; import java.util.logging.Logger;
class Transfer extends java.lang.Thread { final Account myAccount1; Account myAccount2; double myAmount; public Transfer(Account account1,Account account2, double amount) { this.myAccount1=account1; this.myAccount2=account2; this.myAmount = amount; setName("Transfer"); } @Override public void run() { myAccount1.withdraw(myAmount); myAccount2.deposit(myAmount); System.out.println(getName() + " from " + myAccount1.accountType + " to " + myAccount2.accountType + " successfully applied"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
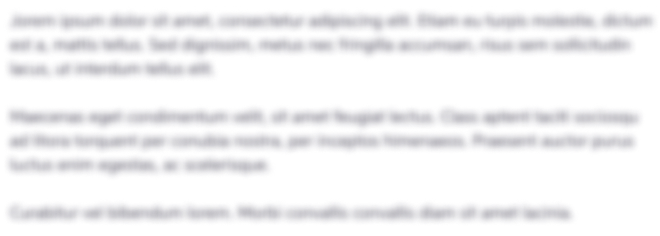
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started