Question
Photo instructions Use Java language and build the classes/GUI from from the follwing code: Main.java import java.io.*; import java.util.*; public class Main { //To store
Photo instructions
Use Java language and build the classes/GUI from from the follwing code:
Main.java
import java.io.*;
import java.util.*;
public class Main {
//To store all bicycle parts.
private static ArrayList bicycleParts;
//flag if changes is made in database or not.
//if yes save it.
private static boolean databaseUpdated = false;
//main database file.
private static File wareHouseFile;
public static void main(String[] args) {
//initialize.
bicycleParts = new ArrayList();
wareHouseFile = new File("wareHouseDb.txt");
//for reading user input.
Scanner inputReader = new Scanner(System.in);
if(wareHouseFile.exists()){
try {
//reading main file.
FileInputStream inputStream = new FileInputStream(wareHouseFile);
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
String line;
while ((line=reader.readLine())!=null){
if(line.isEmpty()){
continue;
}
//get new line from file and add it to bicycle.
bicycleParts.add(BicyclePart.getPartFromString(line));
}
}catch (Exception ignore){
System.out.println("Cant read ware house database.");
}
}
while (true){
System.out.println("Press any key to continue.....");
inputReader.nextLine();
System.out.println("Please select your option from the following menu:");
System.out.println("Read: Read an inventory delivery file");
System.out.println("Enter: Enter a part");
System.out.println("Sell: Sell a part");
System.out.println("Display: Display a part");
System.out.println("SortName: Sort parts by part name");
System.out.println("SortNumber: Sort parts by part number");
System.out.println("Quit:");
System.out.print("Enter your choice: ");
String choice = inputReader.nextLine();
if(choice.equalsIgnoreCase("read")){
readFile(inputReader);
}
else if(choice.equalsIgnoreCase("enter")){
enterPart(inputReader);
}
else if(choice.equalsIgnoreCase("sell")){
sellPart(inputReader);
}
else if(choice.equalsIgnoreCase("display")){
displayPart(inputReader);
}
else if(choice.equalsIgnoreCase("sortName")){
sortByName();
}
else if(choice.equalsIgnoreCase("sortNumber")){
sortByNumber();
}
else if(choice.equalsIgnoreCase("quit")){
saveDatabase();
break;
}
else {
System.out.println("Invalid option chosen.");
}
}
}
//Reading file inventory.txt.
private static void readFile(Scanner inputReader) {
System.out.println("Enter file name to read from: ");
String fileName = inputReader.nextLine();
File file = new File(fileName);
if(!file.exists()){
System.out.println("Error: File not exists.");
return;
}
try {
Scanner fileReader = new Scanner(file);
nextLine:
while (fileReader.hasNextLine()){
String line = fileReader.nextLine();
BicyclePart temp = BicyclePart.getPartFromString(line);
for (BicyclePart bicyclePart:bicycleParts){
if(temp.id==bicyclePart.id){
bicyclePart.listPrice = temp.listPrice;
bicyclePart.salePrice = temp.salePrice;
bicyclePart.onSale = temp.onSale;
bicyclePart.quantity += temp.quantity;
continue nextLine;
}
}
bicycleParts.add(temp);
databaseUpdated=true;
}
}catch (Exception e){
System.out.println("Error: " + e.getMessage());
e.printStackTrace();
}
}
private static void enterPart(Scanner inputReader) {
System.out.print("Enter part name: ");
String name = inputReader.nextLine();
System.out.print("Enter part number: ");
long id = inputReader.nextLong();
inputReader.nextLine();
System.out.print("Enter list price: ");
double listPrice = inputReader.nextDouble();
inputReader.nextLine();
System.out.print("Enter sell price: ");
double salePrice = inputReader.nextDouble();
inputReader.nextLine();
System.out.print("Part is on sale? [true/false]: ");
boolean onSale = inputReader.nextBoolean();
inputReader.nextLine();
System.out.println("Enter quantity: ");
int quantity = inputReader.nextInt();
inputReader.nextLine();
for (BicyclePart bicyclePart: bicycleParts){
if(bicyclePart.id == id){
bicyclePart.listPrice = listPrice;
bicyclePart.salePrice = salePrice;
bicyclePart.onSale = onSale;
bicyclePart.quantity += quantity;
System.out.println("Bicycle part updated.");
databaseUpdated = true;
return;
}
}
BicyclePart bicyclePart = new BicyclePart(id,name,listPrice,salePrice,onSale,quantity);
bicycleParts.add(bicyclePart);
System.out.println("New bicycle part is added.");
databaseUpdated = true;
}
private static void sellPart(Scanner inputReader) {
System.out.println("Enter part number: ");
long partNumber = inputReader.nextLong();
inputReader.nextLine();
for (BicyclePart part: bicycleParts){
if(partNumber == part.id){
sellPart(part);
return;
}
}
System.out.println("Error: Bicycle part not exists.");
}
private static void sellPart(BicyclePart part){
if(part.quantity
System.out.println("Error: No quantity left.");
return;
}
System.out.println("Part Name: " + part.name);
System.out.println("Part Cost: " + (part.onSale?part.salePrice:part.listPrice));
if(part.onSale){
System.out.println("Part is on sale.");
}
System.out.println("Sold Time: " + new Date().toString());
part.quantity--;
databaseUpdated=true;
}
private static void displayPart(Scanner inputReader) {
System.out.print("Enter part name: ");
String partName = inputReader.nextLine();
for (BicyclePart part: bicycleParts){
if(partName.equalsIgnoreCase(part.name)){
displayPart(part);
return;
}
}
System.out.println("Error: Bicycle part not exists.");
}
private static void displayPart(BicyclePart part) {
System.out.println("Part Name: " + part.name);
System.out.println("Part Cost: " + (part.onSale?part.salePrice:part.listPrice));
}
private static void sortByName() {
bicycleParts.sort(new Comparator() {
@Override
public int compare(BicyclePart o1, BicyclePart o2) {
return o1.name.compareToIgnoreCase(o2.name);
}
});
System.out.println("Sorted part names: ");
for (BicyclePart bicyclePart: bicycleParts){
System.out.println(bicyclePart.name);
}
}
private static void sortByNumber() {
bicycleParts.sort(new Comparator() {
@Override
public int compare(BicyclePart o1, BicyclePart o2) {
return Long.compare(o1.id,o2.id);
}
});
System.out.println("Sorted part names: ");
for (BicyclePart bicyclePart: bicycleParts){
System.out.println(bicyclePart.name);
}
}
private static void saveDatabase() {
if(!databaseUpdated)return;
try {
FileOutputStream outputStream = new FileOutputStream("temp.txt");
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(outputStream));
for (BicyclePart bicyclePart:bicycleParts){
writer.write(bicyclePart.toString() + " ");
}
writer.close();
outputStream.close();
if(wareHouseFile.exists())
wareHouseFile.delete();
File file = new File("temp.txt");
file.renameTo(wareHouseFile);
}catch (Exception ignore){
ignore.printStackTrace();
}
}
}
BicyclePart.java
//Class for bicycle parts, its attributes.
public class BicyclePart {
public long id;
public String name;
public double listPrice;
public double salePrice;
public boolean onSale;
public int quantity;
//Constructor.
public BicyclePart(long id, String name, double listPrice, double sellPrice, boolean onSale,int quantity) {
this.id = id;
this.name = name;
this.listPrice = listPrice;
this.salePrice = sellPrice;
this.onSale = onSale;
this.quantity = quantity;
}
//It will take string line like: akjs,1234567890,12.2,52.3,false,10 and
//convert it to bicycle object by splitting it with comma
public static BicyclePart getPartFromString(String string){
String[] parts = string.split(",");
return new BicyclePart(Long.parseLong(parts[1]), parts[0],
Double.parseDouble(parts[2]),Double.parseDouble(parts[3]),
Boolean.parseBoolean(parts[4]),Integer.parseInt(parts[5]));
}
public String toString(){
return name + "," + id + "," + listPrice + "," + salePrice + "," + onSale;
}
}
You are a programmer working for Bicycle Parts Distributorship. BPD has a warehouse full bicycle parts. Each bicycle part has the following attributes . Part name - example names are spoke, saddle, brake pads, and tires . Part number - a part number is a 10-digit number List price this is what the part costs normally . Sale price - this is what the part costs when on sale . On-sale - When this is false, the list price applies, otherwise the sale price applies In addition to the bicycle parts attributes, the warehouse has an attribute (for each bike part) indicating the number of parts in its inventory Quantity this is the number of parts that Bicycle Parts Distributorship has in its warehouse You shall write a program that maintains an inventory of bicycle parts and allows sales associates to sell bicycle parts Warehouse Inventory Update When inventory is delivered to the warehouse, it is accompanied by a text file describing part the delivery. The database of parts in the warehouse is created and updated by reading the inventory delivery file. The file consists of a sequence of comma-separated lines. Each line describes the attributes of a specific auto part. The attributes on each line of the file match th provided in the Introduction section in the following order The quantity indicates the number of parts in the delivery. The following is an example nventory update fileStep by Step Solution
There are 3 Steps involved in it
Step: 1
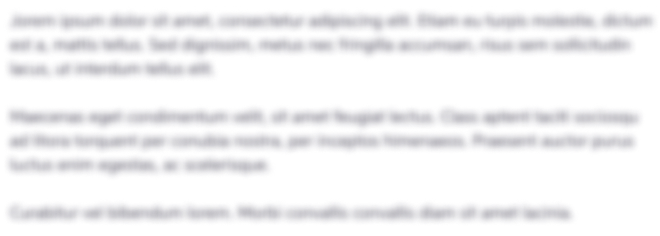
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started