Question
PlayerAnimation.java package Images; import javafx.animation.Interpolator; import javafx.animation.Transition; import javafx.geometry.Rectangle2D; import javafx.scene.image.ImageView; public class PlayerAnimation extends Transition { private final ImageView imageView; private final int count;
PlayerAnimation.java
package Images;
import javafx.animation.Interpolator;
import javafx.animation.Transition;
import javafx.geometry.Rectangle2D;
import javafx.scene.image.ImageView;
public class PlayerAnimation extends Transition {
private final ImageView imageView;
private final int count;
private final int columns;
private final int offsetX;
private final int offsetY;
private final int width;
private final int height;
private int lastIndex;
public PlayerAnimation(ImageView imageView, int count, int columns, int offsetX, int offsetY,
int width, int height) {
this.imageView = imageView;
this.count = count;
this.columns = columns;
this.offsetX = offsetX;
this.offsetY = offsetY;
this.width = width;
this.height = height;
setInterpolator(Interpolator.LINEAR);
}
protected void interpolate(double k) {
final int index = Math.min((int) Math.floor(k * count), count - 1);
if (index != lastIndex) {
final int x = (index % columns) * width + offsetX;
final int y = (index / columns) * height + offsetY;
imageView.setViewport(new Rectangle2D(x, y, width, height));
lastIndex = index;
}
}
}
Game.java
package Images;
import javafx.animation.Animation;
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.geometry.Rectangle2D;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.Pane;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.util.Duration;
public class Game extends Application {
// set constants
static final int CANVAS_WIDTH = 600;
static final int CANVAS_HEIGHT = 600;
// image variables
private Image maze = new Image("images/gamebackground.png");
private Image player = new Image("images/naruto.png");
private Image villain = new Image("images/sasuke_stone.png");
// player variables
private int playerColumns = 6;
private int playerCount = 6;
private int playerOffsetX = 0;
private int playerOffsetY = 0;
private int playerWidth = 32;
private int playerHeight = 31;
private int VillianOffsetX = 0;
private int VillianOffsetY = 0;
private int VillianWidth = 33;
private int VillianHeight = 33;
// wall rectangles
private Rectangle Wall, Wall2, Wall3, Wall4, Wall5, Wall6, Wall7, Wall8, Wall9, Wall10;
private Rectangle Wall11, Wall12, Wall13, Wall14, Wall15, Wall16, Wall17, Wall18, Wall19, Wall20;
@Override
public void start(Stage primaryStage) throws Exception {
// create scene and pane
Pane pane = new Pane();
StackPane stackPane = new StackPane();
Scene scene = new Scene(pane, CANVAS_WIDTH, CANVAS_HEIGHT);
Canvas canvas = new Canvas(CANVAS_WIDTH, CANVAS_HEIGHT);
stackPane.getChildren().add(canvas);
pane.getChildren().add(stackPane);
// text for collision
Text text = new Text();
text.setFill(Color.RED);
text.setFont(Font.font("Arial", FontWeight.BOLD, 12));
// read background image
final ImageView mazeView = new ImageView(maze);
// set the collision walls
Wall = new Rectangle(237, 477, 125, 44);
Wall2 = new Rectangle(0, 357, 43, 204);
Wall3 = new Rectangle(0, 560, 600, 40);
Wall4 = new Rectangle(557, 358, 43, 202);
Wall5 = new Rectangle(117, 398, 44, 82);
Wall6 = new Rectangle(159, 438, 43, 42);
Wall7 = new Rectangle(438, 398, 45, 83);
Wall8 = new Rectangle(397, 437, 43, 45);
Wall9 = new Rectangle(238, 199, 44, 163);
Wall10 = new Rectangle(280, 199, 40, 41);
Wall11 = new Rectangle(318, 199, 44, 164);
Wall12 = new Rectangle(78, 78, 84, 44);
Wall13 = new Rectangle(117, 78, 45, 163);
Wall14 = new Rectangle(238, 78, 124, 43);
Wall15 = new Rectangle(117, 398, 44, 82);
Wall16 = new Rectangle(439, 77, 43, 165);
Wall17 = new Rectangle(439, 77, 82, 44);
Wall18 = new Rectangle(557, 0, 43, 280);
Wall19 = new Rectangle(0, 0, 600, 42);
Wall20 = new Rectangle(0, 0, 42, 281);
// set animation for player
final ImageView playerImageView = new ImageView(player);
playerImageView.setViewport(new Rectangle2D(playerOffsetX, playerOffsetY, playerWidth, playerHeight));
final ImageView villainImageView = new ImageView(villain);
playerImageView.setViewport(new Rectangle2D( VillianOffsetX, VillianOffsetY, VillianWidth, VillianHeight));
// add background image and player
pane.getChildren().add(mazeView);
pane.getChildren().add(playerImageView);
pane.getChildren().add(text);
pane.getChildren().add(villainImageView);
// start location of player
playerImageView.setX(280);
playerImageView.setY(400);
villainImageView.setX(200);
villainImageView.setY(300);
// set keyboard for player movement
playerImageView.setOnKeyPressed(e -> {
switch (e.getCode()) {
case UP:
case W:
playerImageView.setX(playerImageView.getX());
playerImageView.setY(playerImageView.getY() - 5);
break;
case DOWN:
case S:
playerImageView.setX(playerImageView.getX());
playerImageView.setY(playerImageView.getY() + 5);
break;
case LEFT:
case A:
playerImageView.setX(playerImageView.getX() - 5);
playerImageView.setY(playerImageView.getY());
break;
case RIGHT:
case D:
playerImageView.setX(playerImageView.getX() + 5);
playerImageView.setY(playerImageView.getY());
break;
default:
break;
}
});
playerImageView.requestFocus();
primaryStage.setScene(scene);
primaryStage.show();
// set game loop
AnimationTimer game = new AnimationTimer() {
@Override
public void handle(long now) {
checkCollision(playerImageView, Wall, Wall2, Wall3, Wall4, Wall5, Wall6, Wall7, Wall8, Wall9, Wall10,
Wall11, Wall12, Wall13, Wall14, Wall15, Wall16, Wall17, Wall18, Wall19, Wall20, pane, text,
playerImageView);
}
};
game.start();
}
// check for collision
private void checkCollision(Node player, Rectangle wall1, Rectangle wall2, Rectangle wall3, Rectangle wall4,
Rectangle wall5, Rectangle wall6, Rectangle wall7, Rectangle wall8, Rectangle wall9, Rectangle wall10,
Rectangle wall11, Rectangle wall12, Rectangle wall13, Rectangle wall14, Rectangle wall15, Rectangle wall16,
Rectangle wall17, Rectangle wall18, Rectangle wall19, Rectangle wall20, Pane pane, Text text,
ImageView playerImageView) {
boolean collisionDetected = false;
// check collision between player and wall.
if ((player.getBoundsInParent().intersects(wall1.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall2.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall3.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall4.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall5.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall6.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall7.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall8.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall9.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall10.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall11.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall12.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall13.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall14.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall15.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall16.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall17.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall18.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall19.getBoundsInParent()))
|| (player.getBoundsInParent().intersects(wall20.getBoundsInParent())))
collisionDetected = true;
if (collisionDetected) {
text.setX(((ImageView) player).getX() - 10);
text.setY(((ImageView) player).getY() - 10);
playerImageView.setX(((ImageView) player).getX() - 10);
playerImageView.setY(((ImageView) player).getY() - 10);
} else {
text.setText("");
}
}
// main method
public static void main(String[] args) {
launch(args);
}
}
1. Make sure it is using JavaFX and not the older Swing or AWT libraries.
2. Create a background maze (can be an image you make in Photoshop)
3. Put a sprite on it and move it with the keyboard keys (either arrow keys or "asdw" keys).
4. Get your sprite to collide with the walls and borders. Meaning when it collides it doesn't go through the wall.
This time:
1. Get your sprite to collect a treasure and increase the score (text) if they collect (touch) the treasure. The treasure (gold or whatever) sprite should go away as soon as your sprite touches it.
2. Add enemy sprites that can run into your character and if that happens the game is over. The enemy sprites also must respect walls and not go through them.
3. For extra credit add multiple mazes. For example if the character goes to the right most edge of the current maze then a new room is displayed and the character is put in the extreme left of that room. Exactly how the original Legend of Zelda works:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
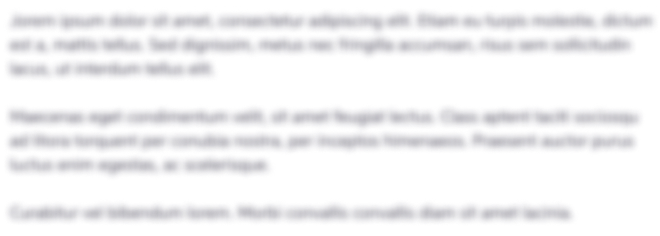
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started