Question
PLEAS SHOW ALL STEPS IN SCREENSHOTS OR COMMENTS DON'T JUST POST THE CODE!!!! EXERCISE 1 SHOULD BE SHOWN IN DETAILS AND SCREENSHOTS ON HOW TO
PLEAS SHOW ALL STEPS IN SCREENSHOTS OR COMMENTS DON'T JUST POST THE CODE!!!!
EXERCISE 1 SHOULD BE SHOWN IN DETAILS AND SCREENSHOTS ON HOW TO DO THEM BECAUSE I HAVE NO BASIC KNOWLEDGE IN CODEING OR JAVA!!
IF YOU CAN'T DO THE ABOVE DON'T SOLVE THIS QUESTION!
Make sure you are using the appropriate indentation and styling conventions
Exercise 1 (20 Points)
- In BlueJ, create a new project called Exec4
- Create a class in that project called TextFiles
- Create a static method named readData that accepts a String parameter named fileName and returns a String output.
- Inside the method, define a String local variable named fileContents. Initially this variable should be assigned an empty string value. This variable is the output of the method and it represents the entire contents of the text file whose name is the passed parameter fileName.
- Create a new object of type File named file. The constructor of the object should accept fileName as an argument. Add the appropriate import statement.
- Create a new Scanner object named scanFile. The constructor of the object should accept file as an argument. Add the appropriate import statement.
- You will notice now that the compiler signals an error. This is because the previous step might cause a checked exception (run-time error) of type FileNotFoundException. Resolve this issue using the throws keyword.
- Create a while loop that iterates as long as the file has more lines in it. Inside the loop, read the contents line by line. Add each line to the fileContentsvariable. Be careful that, the nextLine() method consumes the newline character but doesn't add it to the returned string. We want the file to be read as is including new lines.
- Finally, you should return the entire contents of the file.
Exercise 2 (10 Points)
- Now, you should test your readData method defined in the previous exercise. To do so, create a main method inside the TextFiles class.
- Inside main, define a new Scanner object to read from the user via the keyboard.
- Print a prompt statement to the user to enter the name of the file he wants to read data from. Read the name of the file from the user, then call the readData method and pass to it the value you just read as an argument.
- You will notice that the compiler will signal an error. Remember that the readData method throws a FileNotFoundException. This is a delegation to its caller to take care of the checked exception. The caller is the main method itself! The main method now should decide whether it will handle the exception (using try-catch blocks) or it will just throw it the caller! For now, let the main throw the exception to the caller the same as you did for readData. Can you tell who is the caller of main?!!
- Finally, print the contents of the file to the user. Here is an example on a file called sample.txt:
Enter the file name you want to read: sample.txt File contains: Two roads diverged in a yellow wood, And sorry I could not travel both And be one traveler, long I stood And looked down one as far as I could To where it bent in the undergrowth;
Then took the other, as just as fair, And having perhaps the better claim, Because it was grassy and wanted wear; Though as for that the passing there Had worn them really about the same,
And both that morning equally lay In leaves no step had trodden black. Oh, I kept the first for another day! Yet knowing how way leads on to way, I doubted if I should ever come back.
I shall be telling this with a sigh Somewhere ages and ages hence: Two roads diverged in a wood, and I I took the one less traveled by, And that has made all the difference.
Exercise 3 (25 Points)
- Create a new method called countPattern inside the TextFiles class that accepts two parameters and returns an int output. The first parameter is as String named input and the second is another String named pattern. The purpose of this method is to count the number of times pattern is contained within input. For example, if input is "My name is Mohammed" and pattern is "me", the method returns 2. Before continuing with this exercise, please review the String API (Links to an external site.) and learn about the indexOf, contains, and substring methods.
- Inside the countPattern method, define an int local variable named count and give it an initial value of 0.
- Define a while loop that will iterate as long as the input String contains the pattern. This means that the input String contains the pattern at least once. You will need to use the contains method on the input String and pass pattern String to it as an argument.
- Inside the loop, do the following:
- Increment the variable count by 1.
- Define a local variable named patternIndex. This variable should take the value of the index at which pattern is first found inside the input String. You will need to call the indexOf method on input and pass pattern as an argument.
- Define a local variable named sliceIndex. This variable should be equal to the sum of patternIndex and the length of the pattern String.
- Now, you need to check if sliceIndex is less than the length of the input String. If so, you need to remove a portion of the input String. This portion should be from the starting index of the String till after the index of the last character of the first occurrence of pattern. To do so, you need to call the substring method in the input String and pass to it the sliceIndex variable as an argument. The result of substring should be stored in input. What we did here is literally removing the first occurrence of the pattern String from the input String and count this occurrence.
- Outside of the while loop, you should now return the variable count. This will now represent the total number of occurrences of pattern contained inside input.
Exercise 4 (10 Points)
- Now, you should test your countPattern method defined in the previous exercise. Inside main, prompt the user to enter the pattern he wants to search for. Use a Scanner object to read it from the keyboard.
- Call the countPattern method passing to it the contents of the file you read in exercise 2 (using the readData method) and the pattern you just read from the user in this exercise.
- Then, you should print how many times the pattern is contained inside the file. Here are some examples on the sample.txt file used before. Enter the pattern you want to search for: es The pattern "es" is contained in the file named sample.txt 4 times Enter the pattern you want to search for: one The pattern "one" is contained in the file named sample.txt 3 times Enter the pattern you want to search for: ed The pattern "ed" is contained in the file named sample.txt 6 times
Exercise 5 (20 Points)
- Create a new method named charRectangle that accepts three parameters and returns nothing. The first parameter is a char named ch representing a character to be printed to a file. The second parameter is an int named rows representing the number of times the character should be printed in rows. The third parameter is an int named cols representing the number of times the character should be printed in columns. The main purpose of this method is to print a rectangle of characters to an output file. If the third parameter is passed as 0, then the method should print a triangle of characters to the output file.
- Inside the method, define a new PrintWriter object named out and pass a String to its constructor with value "out.txt". This represents the name of the output file that will receive the characters. Be careful to add the throws keyword to the method's header and to add the appropriate import statement.
- Create an outer for loop that iterates from 1 to rows inclusive.
- Check if the value of cols is not 0. If so, you need to create an inner (nested) loop that iterates from 1 to cols inclusive (For a rectangle). Otherwise, you need to create a different inner loop that will print the characters as a triangle!
- Make sure to print the characters and new lines as appropriate inside the loops, and don't forget to close the out object after finishing. Otherwise, your data will not be saved.
Exercise 6 (15 Points)
- Now, you should test the charRectangle method defined in the previous exercise. Inside main, prompt the user to enter the character, number of rows and number of columns respectively. Use a Scanner object to read all if them from the keyboard. To read the character, you will use the next() method of the scanner object. This will read the character as a string, then use the charAt() method to get the first character. Then use the nextInt() method to read the other two parameters. Be careful about the behavior of next() and nextInt() described previously in class!
- xamples (with contents of the out.txt file displayed): Enter the character you want to use in building the rectangle: * Enter the number of rows: 10 Enter the number of columns: 20
******************** ******************** ******************** ******************** ******************** ******************** ******************** ******************** ******************** ********************
Enter the charachter you want to use in building the rectangle: & Enter the number of rows: 5 Enter the number of columns: 30
&&&&&&&&&&&&&&&&&&&&&&&&&&&&&& &&&&&&&&&&&&&&&&&&&&&&&&&&&&&& &&&&&&&&&&&&&&&&&&&&&&&&&&&&&& &&&&&&&&&&&&&&&&&&&&&&&&&&&&&& &&&&&&&&&&&&&&&&&&&&&&&&&&&&&&
Enter the character you want to use in building the rectangle: % Enter the number of rows: 20 Enter the number of columns: 0
% %% %%% %%%% %%%%% %%%%%% %%%%%%% %%%%%%%% %%%%%%%%% %%%%%%%%%% %%%%%%%%%%% %%%%%%%%%%%% %%%%%%%%%%%%% %%%%%%%%%%%%%% %%%%%%%%%%%%%%% %%%%%%%%%%%%%%%% %%%%%%%%%%%%%%%%% %%%%%%%%%%%%%%%%%% %%%%%%%%%%%%%%%%%%% %%%%%%%%%%%%%%%%%%%%
Step by Step Solution
There are 3 Steps involved in it
Step: 1
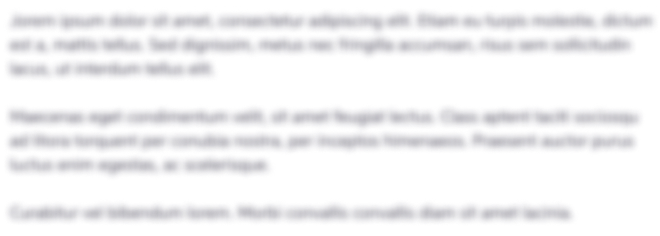
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started