Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please add code for the function printGraph() so that the result looks like this. Please ONLY respond in C++. Additional helper functions is fine This
Please add code for the function printGraph() so that the result looks like this. Please ONLY respond in C++. Additional helper functions is fine
This is the ArrayMaxHeap.cpp file:
// Created by Frank M. Carrano and Tim Henry. // Copyright (c) 2013 __Pearson Education__. All rights reserved. /** Array-based implementation of the ADT heap. @file ArrayMaxHeap.cpp */ #include // for log2 #include // #include
This is the ArrayMeaxHeap.h file:
// Created by Frank M. Carrano and Tim Henry. // Copyright (c) 2016 __Pearson Education__. All rights reserved. /** Array-based implementation of the ADT heap. Listing 17-2. @file ArrayMaxHeap.h */ #ifndef ARRAY_MAX_HEAP_ #define ARRAY_MAX_HEAP_ #include "HeapInterface.h" #include "PrecondViolatedExcep.h" #include #include #include using namespace std; template class ArrayMaxHeap : public HeapInterface { private: static const int ROOT_INDEX = 0; // Helps with readability static const int DEFAULT_CAPACITY = 21; // Small capacity to test for a full heap std::unique_ptr items; // Array of heap items int itemCount; // Current count of heap items int maxItems; // Maximum capacity of the heap // --------------------------------------------------------------------- // Most of the private utility methods use an array index as a parameter // and in calculations. This should be safe, even though the array is an // implementation detail, since the methods are private. // --------------------------------------------------------------------- // Returns the array index of the left child (if it exists). int getLeftChildIndex(const int nodeIndex) const; // Returns the array index of the right child (if it exists). int getRightChildIndex(int nodeIndex) const; // Returns the array index of the parent node. int getParentIndex(int nodeIndex) const; // Tests whether this node is a leaf. bool isLeaf(int nodeIndex) const; // Converts a semiheap to a heap. void heapRebuild(int subTreeRootIndex); // Creates a heap from an unordered array. void heapCreate(); public: ArrayMaxHeap(); ArrayMaxHeap(const ItemType someArray[], const int arraySize); virtual ~ArrayMaxHeap(); // HeapInterface Public Methods: bool isEmpty() const; int getNumberOfNodes() const; void collectNodeInOrder (int i, vector& inorder ) const ; void printGraph() const; int getHeight() const; ItemType peekTop() const; bool add(const ItemType& newData); bool remove(); void clear(); }; // end ArrayMaxHeap // #include "ArrayMaxHeap.cpp" #endif
This is the main.cpp file (Disregard files for PrecondViolatedExcep.cpp and .h):
#include "ArrayMaxHeap.h" #include "ArrayMaxHeap.cpp" #include #include using namespace std; void display(std::string& anItem) { std::cout arr ) { ArrayMaxHeap<:string>* heap1Ptr = new ArrayMaxHeap<:string>(); for (string x: arr ) { heap1Ptr->add( x ); } heap1Ptr->printGraph (); int main() { vector arr { "51", "10", "17", "20", "30", "40", "50", "60", "70", "80", }; testHeap ( arr ); vector arr2 {"50","33", "30", "70", "20", "60", "40", "10", "80", }; testHeap ( arr2 ); return 0; } // end main
Heap level-order traversal: 80705051601740103020 (same as the sequence in th e array) Heap in-order traversal: 10513070206080175040 level 2: +51++601740 level 3: 1030 Heap level-order traversal: 807060502030401033 (same as the sequence in the array) Heap in-order traversal: 105033702080306040 level 0:+++ level 3:1033 Step by Step Solution
There are 3 Steps involved in it
Step: 1
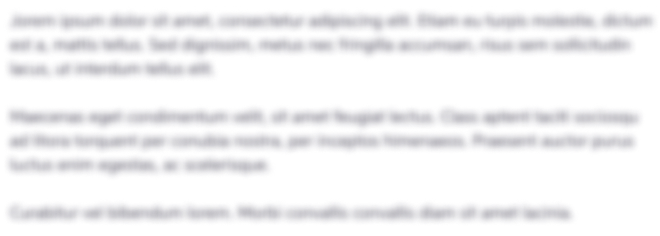
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started