Answered step by step
Verified Expert Solution
Question
1 Approved Answer
PLEASE ANSWER ALL OF THIS, IT IS URGENT, LEAVE COMMENT IF YOU DONT UNDERSTAND SOMETHING // game-loop for Hangman void gameLoop() { int difficulty, tries;
PLEASE ANSWER ALL OF THIS, IT IS URGENT, LEAVE COMMENT IF YOU DONT UNDERSTAND SOMETHING
// game-loop for Hangman void gameLoop() { int difficulty, tries; string word, current; char letter; while (true) { cout > difficulty; while (difficulty 3) { cout _> difficulty; } if (difficulty == 3) { cout > letter; if (!revealLetter(word, letter, current)) { tries--; } if (current == word) { break; } if (tries == 2) { cout
![]()
This is the initial directions and couple codes they gave you that will be part of the code in addition to the following instructions. Add and implement the following functions to your .cpp file:
![]()
#include #include #include #include #include using namespace std;const int g_MAX_WORDS = 1000;int g_word_count = 0;std::string g_words[g_MAX_WORDS];std::string g_definitions[g_MAX_WORDS];std::string g_pos[g_MAX_WORDS];/*@param : The string with the `filename`@post : Reads the words, definitionspos into the global-arraysand set the value of `g_word_count`to the number of words read*/void readWords(string filename) {ifstream fin(filename);if (!fin) {cerrexit(1);}string word;string pos;string definition;string line;string junk;while (fin >> word >> pos >> junk ) {getline(fin, line);definition = line.substr(1);g_words[g_word_count] = word;g_pos[g_word_count] = pos;g_definitions[g_word_count] = definition;g_word_count++;}fin.close();}/*@param : The string with a query word@return : Integer index of the word in`g_words` global-array. Returns-1 if the word is not found@post : Find the index of given `word`in the `g_words` array. Return -1if word is not in the array*/int getIndex(string word) {for (int i = 0; iif (g_words[i] == word) {return i;}}return -1;}/*@param : The string with a query word@return : Return the string definition ofthe word from `g_definitions`global-array. Return "NOT_FOUND" ifword doesn't exist in the dictionary@post : Find the definition of the given `word`Return "NOT_FOUND" otherwise*/string getDefinition(string word) {int index = getIndex(word);if (index != -1) {return g_definitions[index];}else {return "NOT_FOUND";}}/*@param : The string with a query word@return : Return the string part-of-speech(pos)from the `g_pos` global-array. Return"NOT_FOUND" if the word doesn't existin the dictionary.@post : Find the pos of the given `word`Return "NOT_FOUND" otherwise*/string getPOS(string word) {int index = getIndex(word);if (index != -1) {return g_pos[index];}else {return "NOT_FOUND";}}/*@param : The string prefix of a word (the prefixcan be of any length)@return : Integer number of words found that startswith the given `prefix`@post : Count the words that start with the given`prefix`*/int countPrefix(string prefix) {int count = 0;for (int i = 0; iif (g_words[i].find(prefix) == 0) {count++;}}return count;}/*@param word : The string with a new word@param definition : The string with the definition of thenew `word`@param pos : The string with the pos of the new `word`@return : return `true` if the word issuccessfully added to the dictionaryreturn `false` if failed (word alreadyexists or dictionary is full)@post : Add the given `word`, `definition`, `pos`to the end of the respectiveglobal-arrays.The word should not be added to theglobal-arrays if it already existsor if the array reached maximumcapacity(`g_MAX_WORDS`).Update `g_word_count` if the word issuccessfully added*/bool addWord(string word, string definition, string pos) {for (int i = 0; iif (g_words[i] == word) {return false;}}if (g_word_count == g_MAX_WORDS) {return false;}g_words[g_word_count] = word;g_definitions[g_word_count] = definition;g_pos[g_word_count] = pos;g_word_count++;return true;}/*@param word : The string with the word that is tobe edited@param definition : The string with the new definition ofthe `word`@param pos : The string with the new pos of the `word`@return : return `true` if the word is successfullyedited, return `false` if the `word`doesn't exist in the dictionary@post : Replace the given `word`'s definitionand pos with the given `definition` and`pos` (by modifying global-arrays`g_definitions` and `g_pos`).The modification will fail if the worddoesn't exist in the dictionary*/bool editWord(string word, string definition, string pos) {int index = -1;for (int i = 0; iif (g_words[i] == word) {index = i;break;}}if (index == -1) {return false;}g_definitions[index] = definition;g_pos[index] = pos;return true;}/*@param word : The string with the word to be removed@return : Return true if the word is successfullyremoved from the dictionary, false otherwise@post : Remove the given `word`, along with itscorresponding `definition` and `pos` fromthe respective global-arrays. Shift theelements of the arrays to fill the gap.Update `g_word_count` if the word issuccessfully removed*/bool removeWord(string word) {int index = getIndex(word);if (index == -1) {return false;}else {for (int i = index; ig_words[i] = g_words[i+1];g_definitions[i] = g_definitions[i+1];g_pos[i] = g_pos[i+1];}g_words[g_word_count-1] = "";g_definitions[g_word_count-1] = "";g_pos[g_word_count-1] = "";g_word_count--;return true;}}
Do the following well, please follow all directions, send correct codes and make sure you send the output as well so i know it runs, please follow everything well and complete this task: I have asked this type of question various times now but no good response, all answers wrong, make sure this runs on gradedescope and follow all directions well. You have to do this task, and i will send a code that i did for the first 3 tasks so you would have to add your code for the fourth tasks on to my initial code and make sure all of it combines runs. Now we are ready to make Hangman! Oh and here is a dictionary you can use: dictionary.txt The game-loop is a sequence of processes that run continuously as long as the game is running. The three main processes that occur in the game-loop are input, update, and render. Lucky for you, we have provided the game-loop. Make sure to include the following function in your program: The game-loop uses some helper functions that are not implemented yet. You will implement most of them. One of the helper function is getRandomWord( ) . In the game-loop, we are going to choose a random word from the dictionary. Use this function to get a random word from your dictionary: / / MAKE SURE YOU INCLUDE THIS LIBRARY! \#include string getRandomWord( ) \{ srand((unsigned) time(NULL)); int index =rand() \% g_word_count; return g_words[index]; \} *) int getTries(int difficulty); /* For example : calling "printattemps (2,1) " would print "ooxxxxx" . Based on given "difficulty", we know the total tries is 7 (from "getTries(1)"). Also, the player has 2 "tries" remaining based on the given parameter. Therefore, the function prints two " 0 "s to indicate the remaining tries and 5 " x "s to indicate the tries that have been used (72=5) */ void printAttempts(int tries, int difficulty); /* For example : Let's say we have the following main function: int main( ) string w="g cout revealLetter ("good", 'o", "g_.") endl; cout w w end; \} The first "cout' will print 1 because the letter ' 0 ' exists in "good". Thus, the function returned "true". The second "cout" will print "goo_". The variable "w has been modified by the function to reveal all the "o"s in "good" resulting in "goo_" / bool revealLetter(string word, char letter, string \¤t) lement and test each function before moving on to the next. Once you have all the functions Implement and test each function before moving on to the next. Once you have all the functions implemented correctly, try out your game by running gameLoop() from main() function. Submit only one file to gradescope without the main() function. Every project starts somewhere. While the hangman game is functional, it can be improved significantly. For example: we can prevent users from entering letters that have been entered already we can add a GUI to make the experience more user-friendly and the list goes on. You can add your own rules to the game to make something unique that you can put in your Portfolio to impress your friends and potential recruiters. These are all the instructions, please do the code well, make your functions and add the codes they gave (you have to find out whether the code they gives come before or after your functions) You have to add this code you make to my code that i have done already and runs on gradesescope, so make sure your code combined works with this, and send whole code This is my code: Please do all of it went, list the functions as it said, and also add the headers that they have as well. PLEASE FOLLOW ALL DIRECTIONS, MANY TIMES I MAKE INSTRUCTIONS CLEAR BUT THEY DONNT. UNDERSTAND
Step by Step Solution
There are 3 Steps involved in it
Step: 1
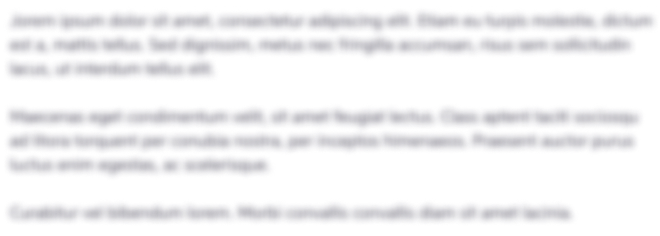
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started