Question
Please answer all parts of this question: given code: #include #include struct address_tag { int houseNum; char street[30]; char city[30]; char state[3]; }; typedef struct
Please answer all parts of this question:
given code:
#include
#include
struct address_tag {
int houseNum;
char street[30];
char city[30];
char state[3];
};
typedef struct address_tag Address;
struct person_tag {
char firstName[20];
char lastName[20];
Address addr;
};
typedef struct person_tag Person;
void printAddress(Address addr);
void printPerson(Person per);
void displayPeople(Person ppl[], int pplCount);
void addPerson(Person newPerson, Person ppl[], int *pplCount);
void moveToTacoma(Person *per);
void moveAllToTacoma(Person ppl[], int pplCount);
int main(void) {
Person people[10] = {
{ "Joseph", "Miller", { 10, "Downing", "London", "WA" } },
{ "Anne", "Simpson", { 1600, "Pennsylvania Ave", "Columbia", "WA" } },
{ "Peter", "Price", { 832, "Main St.", "Dallas", "TX" } }
};
int peopleCount = 3;
displayPeople(people, peopleCount);
Person historicFigure = { "Thea", "Foss", { 17, "Prospect St.", "Tacoma", "WA" } };
addPerson(historicFigure, people, &peopleCount);
displayPeople(people, peopleCount);
moveAllToTacoma(people, peopleCount);
printf("After massive relocation "); // Now this works!
displayPeople(people, peopleCount);
return 0;
}
void printAddress(Address addr) {
printf("%d %s ",addr.houseNum, addr.street);
printf("%s, %s ",addr.city, addr.state);
}
void printPerson(Person per){
printf("%s, %s ",per.lastName, per.firstName);
printAddress(per.addr);
}
void displayPeople(Person ppl[], int pplCount) {
int i;
for (i = 0; i
printPerson(ppl[i]);
printf(" ");
}
}
void addPerson(Person newPerson, Person ppl[], int *pplCount) {
ppl[*pplCount] = newPerson;
(*pplCount)++;
}
void moveToTacoma(Person *per) {
strcpy(per->addr.city, "Tacoma");
strcpy(per->addr.state, "WA");
}
void moveAllToTacoma(Person ppl[], int pplCount) {
int i = 0;
for (i = 0; i
moveToTacoma(&ppl[i]);
}
}
1. Download structinstruct3.c that we analyzed in class into your own lab03 directory. Rename the file lab03structs.c. 2. Open emacs by opening the terminal and typing emacs 3. Open lab03structs.c in emacs and look over the code. 4. Create another structure definition called comm_tag that contains two strings of size 30 each: email and phone 5. Typedef this structure definition to name it Com and add Com type variable to the person_tag structure as the last field (in the manner similar to how the address variable appears in the person_tag) 6. Update the code in main so that these 2 new fields are given some values when creating an array of people. 7. Add a function printComms that takes a variable of Com type and prints the email and the phone number. 8. Update function printPerson so that it calls printComms (in a manner similar to how it calls printAddress). 9. Compile and run in Emacs by opening the terminal in the 2nd emacs frame (as explained above). 10. Add a function changeEmail that takes one Person variable and a new e-mail address for that person. Change the existing e-mail to this new e-mail. Assume that the new e-mail is of appropriate length (no error checking required). Test in main by passing one of the array records to this function along with a new e-mail. 11. Compile and run in Emacs. Take a couple of screenshots that show you edited and ran the code in Emacs. Do it for each lab partner since we are working remotely this quarter. 12. Compile and run in Emacs. 13. Add comments to the following function prototypes to indicate the logical data flow of parameters (in, out, inout for each parameter): addPerson, printComms, changeEmail
Step by Step Solution
There are 3 Steps involved in it
Step: 1
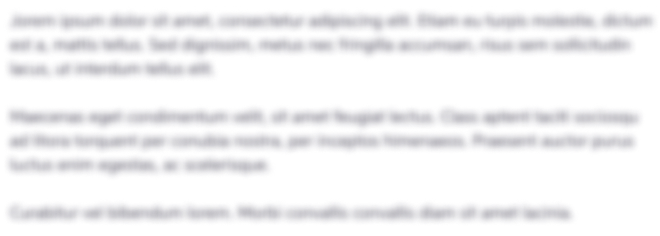
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started