Question
Please answer in C++ and only edit the searcher h Implement the Searcher class's BinarySearch() template function in the Searcher.h file. Access Searcher.h by clicking
Please answer in C++ and only edit the searcher h
Implement the Searcher class's BinarySearch() template function in the Searcher.h file. Access Searcher.h by clicking on the orange arrow next to main.cpp at the top of the coding window. The function performs a binary search on the sorted array (first parameter) for the key (third parameter). BinarySearch() returns the key's index if found, -1 if not found.
Compare an array element to the key using the Compare() member function of the comparer object passed as BinarySearch()'s last parameter. comparer.Compare(a, b) returns an integer:
greater than 0 if a > b
less than 0 if a < b
equal to 0 if a == b
A few test cases exist in main() to test BinarySearch() with both string searches and integer searches. Clicking "Run program" will display test case results, each starting with "PASS" or "FAIL". Ensure that all tests are passing before submitting code.
Each test in main() only checks that BinarySearch() returns the correct result, but does not check the number of comparisons performed. The unit tests in the submit mode check both BinarySearch()'s return value and the number of comparisons performed.
Searcher h
#ifndef SEARCHER_H #define SEARCHER_H
#include "Comparer.h"
template
#ifndef COMPARER_H #define COMPARER_H
// Comparer is an abstract base that can compare two items with the Compare() // function. The Compare() function compares items a and b and returns an // integer: // - greater than 0 if a > b, // - less than 0 if a < b, or // - equal to 0 if a == b template
#endif
main cpp
#include
// Implementation of PrintSearches is below main() template
int main(int argc, char *argv[]) { // Perform sample searches with strings string sortedFruits[] = { "Apple", "Apricot", "Banana", "Blueberry", "Cherry", "Grape", "Grapefruit", "Guava", "Lemon", "Lime", "Orange", "Peach", "Pear", "Pineapple", "Raspberry", "Strawberry" }; int sortedFruitsSize = sizeof(sortedFruits) / sizeof(sortedFruits[0]); string fruitSearches[] = { "Nectarine", "Mango", "Guava", "Strawberry", "Kiwi", "Apple", "Raspberry", "Carrot", "Lemon", "Bread" }; int fruitSearchesSize = sizeof(fruitSearches) / sizeof(fruitSearches[0]); int expectedFruitSearchResults[] = { -1, -1, 7, 15, -1, 0, 14, -1, 8, -1 }; StringComparer stringComparer; PrintSearches(sortedFruits, sortedFruitsSize, fruitSearches, fruitSearchesSize, stringComparer, expectedFruitSearchResults, true); // Perform sample searches with integers int integers[] = { 11, 21, 27, 34, 42, 58, 66, 71, 72, 85, 88, 91, 98 }; int integerSearches[] = { 42, 23, 11, 19, 87, 98, 54, 66, 92, 1, 14, 21, 66, 87, 83 }; int expectedIntegerSearchResults[] = { 4, -1, 0, -1, -1, 12, -1, 6, -1, -1, -1, 1, 6, -1, -1 }; IntComparer intComparer; PrintSearches(integers, sizeof(integers) / sizeof(integers[0]), integerSearches, sizeof(integerSearches) / sizeof(integerSearches[0]), intComparer, expectedIntegerSearchResults, false); return 0; }
template
#ifndef STRINGCOMPARER_H #define STRINGCOMPARER_H
#include
// StringComparer inherits from Comparer
#endif
#ifndef INTCOMPARER_H #define INTCOMPARER_H
#include "Comparer.h"
// IntComparer inherits from Comparer
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
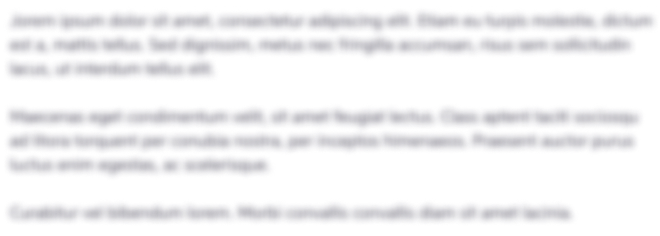
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started