Question
Please Answer the question between /* */ C PROGRAMMING #include #include #include #include altqueue.h #define NAMELEN 100 struct node_s { char name[NAMELEN]; struct node_s *next;
Please Answer the question between /* */ C PROGRAMMING
#include
#include "altqueue.h"
#define NAMELEN 100
struct node_s { char name[NAMELEN]; struct node_s *next; };
/* This is the pointer to the head of the freelist. */
static struct node_s *freelist;
void altqueue_startup(void) { /* TODO: Put your code here to initialize the freelist. */ }
void altqueue_cleanup(void) { /* Put your code here to empty and free all nodes on the freelist. */ }
/****************************************************************** * Initialize a queue (it starts empty) */ void altqueue_init(altqueue_t *q) { q->first = q->last = NULL; }
/****************************************************************** * Add a new name to the queue */ void altqueue_enqueue(altqueue_t *q, char *name) { struct node_s *newnode = NULL;
/* : Put your code here to get a new node. Note that there are * two cases, depending on whether or not a node is available on * the freelist. */ if (newnode == NULL) { fprintf(stderr, "Out of memory! Exiting program. "); exit(1); }
strcpy(newnode->name, name); newnode->next = NULL;
if (q->first == NULL) q->first = newnode; else q->last->next = newnode;
q->last = newnode; }
/****************************************************************** * Return the name at the front of the queue. Returns NULL if the * queue is empty. */ char *altqueue_front(altqueue_t *q) { if (q->first == NULL) { return NULL; } else { return q->first->name; } }
/****************************************************************** * Remove the name at the front of the queue. Calls with an empty * queue will be ignored. */ void altqueue_dequeue(altqueue_t *q) { if (q->first != NULL) { struct node_s *curr = q->first; q->first = q->first->next; if (q->first == NULL) q->last = NULL;
/* Put your code here to place the curr node on the freelist. */ } }
/****************************************************************** * Print out the names in the queue, with commas separating them. If * the queue is empty, it just prints "EMPTY". */ void altqueue_print(altqueue_t *q) { struct node_s *curr = q->first;
if (curr == NULL) { printf("EMPTY "); } while (curr != NULL) { printf("%s", curr->name); curr = curr->next; if (curr != NULL) printf(", "); } printf(" "); }
/****************************************************************** * Destroy a queue - frees up all resources associated with the queue. */ void altqueue_destroy(altqueue_t *q) { while (q->first != NULL) altqueue_dequeue(q); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
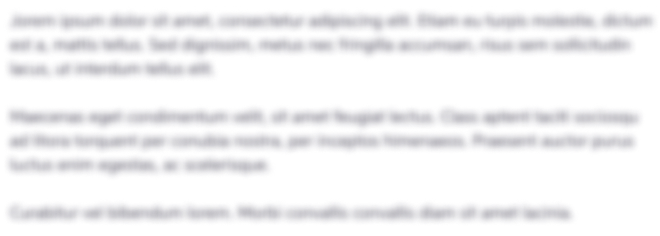
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started