Question
PLEASE ANSWER THE QUESTION CORRECTLY AND ACCURATELY. ENSURE YOU SHOW THE CORRECT CODE AND THE OUTPUT THAT THE CODE RUNS ON THE SCREEN. ALL THE
PLEASE ANSWER THE QUESTION CORRECTLY AND ACCURATELY. ENSURE YOU SHOW THE CORRECT CODE AND THE OUTPUT THAT THE CODE RUNS ON THE SCREEN. ALL THE HEADER AND CLASS FILES ARE GIVEN REQUIRED FOR THE PROGRAM.
Background You are tasked to create a program for manipulating records of patients and employees in a hospital. The program would allow hospital administrators to query the data and to obtain some summary information. The data of patients and employees is initialized using the function populateRecords(). The data is stored in an array, and the size of the array is given using the define NUM_RECORDS. The patients and employees data: a. Shared data: First Name first name Family Name family name, Telephone telephone number b. Employee only data: Salary annual salary Years of service number of years at the hospital Position the position at the hospital Department the department that the doctors is working at. c. Patient only data: Department - the department the patient is in Daily cost cost of having the patient in the hospital for one day Number of days number of days that the patient is hospitalized Severity the seriousness of the patient illness. The following information defines the records fields: Common fields First Name 14 characters long (not including the \0 character) Family Name 14 characters long (not including the \0 character) Patient fields use bit fields to minimize the size Department an integer in the range of 1-6 Daily cost an integer number in the range of 1-50 Number of days in hospital an integer number in the range of 0-30 Severity an integer number in the range of 0-3 Employee fields use bit fields to minimize the size Salary a real non negative number Years of service an integer in the range of 0-60 Position an integer in the range of 0-3 Department - range 1-6 (integer) Tasks In this assignment you will write a short program to manipulate person data of a hospital: patients and employees). 1) Suggestions As you code your small helping functions write small test functions to ensure that the code is correct. This will allow you to focus on the logic of your program without worrying about the simple functions. In particular make sure that you have a few functions that check the validity of the input. Create function for each menu option. The function should accept as input the array of person and the number of elements in the array. Tasks 1. Modifying patient, employee, and person structures (15 pts) Grading (this includes some bonus points) 5 points for minimizing the structure sizes 10 pointes for using proper packing order and bit fields. o 3 points for packing the structure in the correct order o 7 points for using bit fields correctly (including data types) Initial design of the data structures determined that three data structures are sufficient: PatientRec - patient specific data, EmployeeRec - employee specific data and, PersonRec person data that is commone to both. // structure contains patient information typedef struct patient { int department; // department in hospital long dailyCost; // cost of hospitalization per day short numDaysInHospital; // number of days in hospital char severity; // severity of illness } PatientRec; // structure contains employee information typedef struct employee { int position; // position of employee in hospital; long yearsService; // years of service unsigned long department; // department in hospital float salary; // annual salary } EmployeeRec; // structure contains person information typedef struct person { char firstName[NAME_SIZE]; char familyName[NAME_SIZE]; char emplyeeOrPatient; union { EmployeeRec emp; PatientRec patient; }; } PersonRec; The type of many of the fields does not correspond to the value that a field must hold. Examples are: Family Name in PersonRec the family name can be at most 14 characters long. Storing the \0 character with the family name means that only 15 characters are required for the field familyName. However, the designer allocated 64 character to the family name (the designer set NAME_SIZE to 64). Here you can reduce the size by setting the NAME_SIZE to 15 In PatientRec the severity field can have values 0-3 which require only 2 bits. However, the initial design set the field severity to an int which is 32 bits. Here you can reduce the size to 2 bits for this field. In this task you need to modify each structure so that: Each field can store all the values in the required range The structure requires as little memory space as possible. In order to achieve it you can: Change the type of each of the fields in the structure Change the order of each of the fields in the structure Use bit fields to create subtypes of the integer family of data types - char, short, int or long (either signed or unsigned). You cannot change the fields names, or the structures names. 2. Populating the array (0 pts) The program creates an array of 20 persons. It does it by invoking the function populateArray() in file populate_array.c (file populate_array.h contains that function prototype). Note that you will have to link the file with your code. 3. Menu (5 pts) Create a menu function that will allow the user to manipulate the lists. 3.1. Create a menu function (int menu()) which will return the menu option that the user has selected. 3.2. Display the menu options: 3.2.1. 1. Print all employees 3.2.2. 2. Print all patients 3.2.3. 3. Search patient using Family Name 3.2.4. 4. Summary of Employees Data 3.2.5. 5. Summary of Patients data 3.2.6. 6. Size of structures (PersonRec, PatientRec and EmployheeRec) 3.2.7. 0. Quit 3.3. The program will display the menu to the user and ask the user to enter an option. If the selected option is valid that the system will execute the option. Otherwise, the system will prompt the user that the option is not valid and then redisplay the menu. 4. Menu actions (70pts) 4.1. (5 pts.) Switch Statement Create a switch statement in the main function, which will process the menu item selected by the user. Each case will invoke a corresponding function that will execute one of the actions described in 4.2 4.8. Here you will be responding to the action selected by the user as follows 4.2. Print All Employees (10pts) Print all the employees. Hints a. create a function to print a single employee then call the function in a for loop checking if the record is an employee record; b. use sprintf to print the first name and the last name to a temporary string. Then using the temporary string print the name using the formatting. The xxx in the print format represent the number of characters that the printed value must occupy. For example depts.: is followed by xx. Here the dept: number has to occupy 2 spaces. If the value does not have 2 digits (e.g., 14) but rather one digit (e.g., 1) than a space must be used the 1such as dept: 1 Print format First Name Family Name (33 char) dept:xx salary:xxxxxx.xx position:xx years of service:xxxx salary to-date:xxxxxxxx.xx For example: Don Johnson dept: 5 salary: 35510.00 position: 2 years of service: 17 salary to-date: 603670.00 Printout example Hospital Employees Don Johnson dept: 5 salary: 35510.00 position: 2 years of service: 17 salary to-date: 603670.00 John Ouster dept: 2 salary: 7644.70 position: 3 years of service: 47 salary to-date: 359300.91 Grading: 5 points for printing as required 5 points for printing all employees 4.3. Print all Patients (10pts) Print all the patients. Hints a. create a function to print a patient then use the function is a for loop chcking each record if it is an employee record; b. use sprintf to print the first name and the last name to a temporary string. Then using the temporary string print the name using the formatting. Print format First Name Family Name (33) characters dept:xx days in hospital:xxx severity:xx daily cost:xxx total cost:xxxxx John Johnson dept: 3 days in hospital: 21 severity: 0 daily cost: 32 total cost: 672 Output example Patient List John Johnson dept: 3 days in hospital: 21 severity: 0 daily cost: 32 total cost: 672 David Carp dept: 5 days in hospital: 26 severity: 1 daily cost: 37 total cost: 962 Assignment 3 COMP 2401 Page8 of 11 Grading: 5 points for printing as required 5 points for printing all patients 4.4. Print Patients by Family Name (10 pts.) 4.4.1. Prompt the user to enter a family name 4.4.2. Search the list for all patients with a matching family name and print their record as above (Section 4.3) Grading: 10 points for finding and printing a matched patient 4.5. Summary of Employees Data (20 pts.) here you will provide a summary data of the employees in the hospital The summary will consist of two parts: overall summary and summary by position. 4.5.1. Overall summary Here the program will provide the number of employees in the hospital, the total salary that is paid to the employees and the average salary The output format is Total number of employees:xxx total salary:xxxxxxx.xx average salary:xxxxx.xx For example: Total number of employees: 11 total salary: 248450.75 average salary:22586.43 4.5.2. Summary by position The hospital has four postions 0, 1, 2, and 3. Here you will provide a summary of the employees by positions. For each position you will provide the number of employees in the position, the total salary of the employees in the position and the average salary in the position. The format is: position[0] employees:xx total salary:xxxxxx.xx average salary:xxxxx.xx For example position[0] - employees: 2 total salary: 21997.44 average salary:10998.72 An example of the output is: Employee Summary Total number of employees: 11 total salary: 248450.75 average salary:22586.43 Summary by position position[0] - employees: 2 total salary: 21997.44 average salary:10998.72 position[1] - employees: 3 total salary: 91936.06 average salary:30645.35 position[2] - employees: 3 total salary: 70829.72 average salary:23609.91 position[3] - employees: 3 total salary: 63687.52 average salary:21229.17 Grading: 4 points for correctly printing the employee summary 4 points for formatting the employee summary 12 points for the summary by department o 6 points for correctly following the departmental data formatting o 6 points for correctly computing the summary data: number of employees, total salary and average salary. 4.6. Summary of Patient Data (10 pts.) here you will provide a summary data of the patients in the hospital The summary will consist of two parts: overall summary and summary by department. 4.6.1. Overall summary (10 pts.) Here the program will provide the number of patients in the hospital, the total cost to date of caring for the patients and the average cost per patient. The total cost to date is the sum of caring for all patients (daily cost per patient x number of days in hostpital) The output format is Total number of patients:xxx total cost to-date:xxxxxxx.xx average cost per patient to-date:xxxxx.xx For example: Total number of patients: 9 total cost to-date: 6052.00 average cost per patient to-date: 672.44 4.6.2. Summary by Department (BONUS section 15 points) The hospital has six departments 1, 2, 3, 4, 5, 6. Here you will provide a summary of the patients by department. For each department you will provide the number of patients, the total cost of caring for the patients in the department to date, the daily cost of caring and the average daily cost per patient. The cost to-date is the sum of the caring for each patient (number of days in the hospital x daily cost). The daily cost is the sum of daily cost for all patients in the department. The format is: Department [1] - patients:xxx cost to-date:xxxx.xx daily cost:xxxx.xx average daily cost per patient:xxxx.xx For example Department [1] - patients: 1 cost to-date: 234.00 daily cost: 26.00 average daily cost per patient: 26.00 An example of the output is: Patient Summary Total number of patients: 9 total cost to-date: 6052.00 average cost per patient to-date: 672.44 Summary by Department Department [1] - patients: 1 cost to-date: 234.00 daily cost: 26.00 average daily cost per patient: 26.00 Department [2] - patients: 1 cost to-date:1029.00 daily cost: 49.00 average daily cost per patient: 49.00 Department [3] - patients: 2 cost to-date:1730.00 daily cost: 78.00 average daily cost per patient: 39.00 Department [4] - patients: 0 cost to-date: 0.00 daily cost: 0.00 average daily cost per patient: 0 Department [5] - patients: 3 cost to-date:1487.00 daily cost: 63.00 average daily cost per patient: 21.00 Department [6] - patients: 2 cost to-date:1572.00 daily cost: 87.00 average daily cost per patient: 43.50 Grading: 5 points for correctly printing the patient summary 5 points for formatting the patient summary 15 points for the summary by department o 6 points for correctly following the departmental data formatting o 9 points for correctly computing the summary data: number of patients, cost to-date, daily cost, average daily cost per patient. 4.7. Size of structures (PersonRec, PatientRec and EmployheeRec) (5 pts) Print the size of the three structures Format to follow: Size of structures Size of PersonRrec = 148 Size of EmployeeRrec = 16 Size of PatientRrec = 12 Note that the above example is for the provided structures. They do not reflect the minimization of the structures. 4.8. Quit (5 pts.) 4.8.1. Warn the user that he/she is about to quit and ask whether to proceed (y/n) Grading: 5 points for correctly warning the user and quitting
STRUCT.H
// file is struct.h #ifndef STRUCT_HEADER #define STRUCT_HEADER
/* The file contains the structure declarations required by the program
Revisions: February 10, 2018 Doron Nussbaum, initial version
*/
/***************************************************************/
//DEFINES
#define NAME_SIZE 64 // size of array for patient/employee name (maximum name length is NAME_SIZE - 1)
//Patient related defines #define PATIENT_TYPE 1 // determine that the record is a patient record #define NUM_SEVERITIES 4 // number of different states of a patient #define LIGHT 0 #define STABLE 1 #define ACCUTE 2 #define IMMEDIATE_SURGERY 3
//Employee related defines #define EMPLOYEE_TYPE 0 // determines that the record is an employee record #define MAX_POSITIONS 4 // number of different positions #define DOCTOR 0 #define NURSE 1 #define SURGEON 2 #define ADMIN 3
// Department defines #define MAX_DEPARTMENTS 6
// structure contains patient information typedef struct patient { int department; // department in hospital long dailyCost; // cost of hospitalization per day short numDaysInHospital; // number of days in hospital char severity; // severity of illness } PatientRec;
// structure contains employee information typedef struct employee { int position; // position of employee in hospital; long yearsService; // years of service unsigned long department; // department in hospital float salary; // annual salary } EmployeeRec;
// structure contains person information typedef struct person { char firstName[NAME_SIZE]; char familyName[NAME_SIZE]; char emplyeeOrPatient; union { EmployeeRec emp; PatientRec patient;
}; } PersonRec;
#endif
POPULATERECORDS.H
// File populateRecords.h /* Purpose: populate the records of the patient and the employee
The user can populate the reocrds of an array of tyupe person.
Revisions: 15/2/2018 - Doron Nussbaum file created
*/
#ifndef POPULATE_ARRAY_HEADER #define POPULATE_ARRAY_HEADER
#include "struct.h"
void populateRecords(PersonRec *person, int numRecords); // parameter: person and array of person records, nuMRecords the array size
#endif
POPULATERECORDS.C
/ file is populateRecords.c /* Purpose: populate the records of the patient and the employee
The file consists of several functions for ppopulating a person array and thus populating patient records and employee records in the array.
The records are populated using random number generator. No special seed point is given (using the default seed of 1).
Revisions: 15/2/2018 - Doron Nussbaum file created */
/***********************************************************/ //INCLUDES
#include "stdio.h" #include "stdlib.h" #include "string.h" #include "populateRecords.h"
/*********************************************************/ //FUNCTION PROTOTYPES
void populateEmployee(EmployeeRec *employee); void populatePatient(PatientRec *patient);
/**************************************************************/ /* populates an employees
input
emp - an employee record
output emp - populated employee
*/
/*****************************************************************/ // DEFINES
#define MAX_YEARS_SERVICE 63 #define MAX_SALARY 300000
/*****************************************************************/
void populateEmployee(EmployeeRec *emp) {
emp->salary = (rand() % MAX_SALARY) * 1.34f ; emp->yearsService = rand() % MAX_YEARS_SERVICE+1; emp->department = rand() % MAX_DEPARTMENTS + 1; emp->position = rand() % MAX_POSITIONS;
}
/**************************************************************/ /* populate a patient record
input patient - a student record
output patient - a student record
*/
#define MAX_DAILY_COST 50 #define MAX_DAYS_IN_HOSPITAL 30
void populatePatient(PatientRec *p) {
p->department = rand() % MAX_DEPARTMENTS + 1; p->dailyCost = rand() % MAX_DAILY_COST+5; p->severity = rand() % NUM_SEVERITIES; p->numDaysInHospital = rand() % MAX_DAYS_IN_HOSPITAL+1;
}
/**************************************************************/ /* populate an employee record
input person - an array of persons numPersons - number of person in the array
output emp - array of employees
assumption: numPersons is <= the size of the array person */ #define NUM_NAMES 7 void populateRecords(PersonRec *person, int numPersons) { int i = 0; int j; static int initRandom = 0;
char *fn[NUM_NAMES] = { "John", "Jane", "David", "Dina", "Justin","Jennifer", "Don" }; char *sn[NUM_NAMES] = { "Smith", "Johnson", "Mart", "Carp", "Farmer","Ouster","Door" };
if (!initRandom) { initRandom++;
}
while (i < numPersons) { j = rand() % NUM_NAMES; person->firstName[NAME_SIZE - 1] = '\0'; strncpy(person->firstName, fn[j], NAME_SIZE - 1); j = rand() % NUM_NAMES; person->familyName[NAME_SIZE - 1] = '\0'; strncpy(person->familyName, sn[j], sizeof(person->familyName) - 1); if (rand() % 2) { person->emplyeeOrPatient = PATIENT_TYPE; populatePatient(&person->patient); } else { populateEmployee(&person->emp); person->emplyeeOrPatient = EMPLOYEE_TYPE; } person++; i++; } }
PATIENT.H
// file is patient.h
// include files #include "struct.h"
/******************************************************/ // function prototypes
void printPatients(PersonRec *person, int numRecords); void printPatientSummary(PersonRec *person, int numRecords); void searchPatient(PersonRec *person, int numRecords); void printPatientSummary(PersonRec *person, int numRecords);
PATIENT.C
#include "string.h" #include "stdio.h" #include "patient.h"
/********************************************************************/ void printPatient(PersonRec person)
{
// add code
}
/********************************************************************/ void printPatients(PersonRec *person, int numRecords) {
// add code
}
/********************************************************************/ void printPatientSummary(PersonRec *person, int numRecords) {
// add code
}
/********************************************************************/ void searchPatient(PersonRec *person, int numRecords)
{
// add code
}
HOSPITAL.C
#include "stdio.h" #include "stdlib.h" #include "struct.h" #include "string.h" #include "populateRecords.h" #include "patient.h" #include "employee.h"
#define NUM_RECORDS 20
int main() { struct person person[NUM_RECORDS]; char rc = 0;
// populating the array person with data of patients and employees populateRecords(person, NUM_RECORDS);
// add code here //
return 0; }
EMPLOYEE.H
// file is emoloyee.h
// include files #include "struct.h"
/******************************************************/ // function prototypes
void printEmployees(PersonRec *person, int numRecords); void printEmployeesSummary(PersonRec *person, int numRecords);
EMPLOYEE.C
#include "employee.h" #include "stdio.h"
void printEmployee(PersonRec person)
{
// add code
}
void printEmployees(PersonRec *person, int numRecords) {
// add code
}
void printEmployeesSummary(PersonRec *person, int numRecords) {
// add code
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
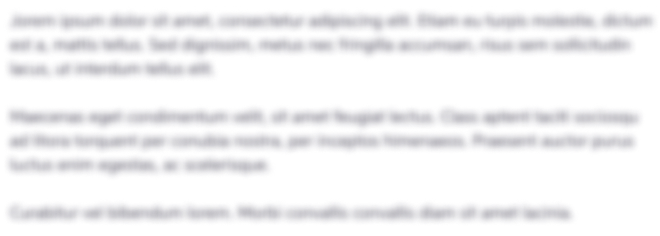
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started