Question
Please answer these questions. Its due tonight JakeBox.zip //******************************************************************** // JukeBox.java Author: Lewis/Loftus // // Demonstrates the use of a combo box. //******************************************************************** import javax.swing.*;
Please answer these questions. Its due tonight
JakeBox.zip
//********************************************************************
// JukeBox.java Author: Lewis/Loftus
//
// Demonstrates the use of a combo box.
//********************************************************************
import javax.swing.*;
public class JukeBox
{
//-----------------------------------------------------------------
// Creates and displays the controls for a juke box.
//-----------------------------------------------------------------
public static void main(String[] args)
{
JFrame frame = new JFrame("Java Juke Box");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JukeBoxControls controlPanel = new JukeBoxControls();
frame.getContentPane().add(controlPanel);
frame.pack();
frame.setVisible(true);
}
}
//********************************************************************
// JukeBoxControls.java Author: Lewis and Loftus
//
// Represents the control panel for the juke box.
//********************************************************************
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.applet.AudioClip;
import java.net.URL;
public class JukeBoxControls extends JPanel
{
private JComboBox musicCombo;
private JButton stopButton, playButton;
private AudioClip[] music;
private AudioClip current;
//-----------------------------------------------------------------
// Sets up the GUI for the juke box.
//-----------------------------------------------------------------
public JukeBoxControls()
{
URL url1, url2, url3, url4, url5, url6;
url1 = url2 = url3 = url4 = url5 = url6 = null;
// Obtain and store the audio clips to play
try
{
url1 = new URL("file", "localhost", "westernBeat.wav");
url2 = new URL("file", "localhost", "classical.wav");
url3 = new URL("file", "localhost", "jeopardy.au");
url4 = new URL("file", "localhost", "newAgeRythm.wav");
url5 = new URL("file", "localhost", "eightiesJam.wav");
url6 = new URL("file", "localhost", "hitchcock.wav");
}
catch (Exception exception) {}
music = new AudioClip[7];
music[0] = null; // Corresponds to "Make a Selection..."
music[1] = JApplet.newAudioClip(url1);
music[2] = JApplet.newAudioClip(url2);
music[3] = JApplet.newAudioClip(url3);
music[4] = JApplet.newAudioClip(url4);
music[5] = JApplet.newAudioClip(url5);
music[6] = JApplet.newAudioClip(url6);
JLabel titleLabel = new JLabel("Java Juke Box");
titleLabel.setAlignmentX(Component.CENTER_ALIGNMENT);
// Create the list of strings for the combo box options
String[] musicNames = {"Make A Selection...", "Western Beat",
"Classical Melody", "Jeopardy Theme", "New Age Rythm",
"Eighties Jam", "Alfred Hitchcock's Theme"};
musicCombo = new JComboBox(musicNames);
musicCombo.setAlignmentX(Component.CENTER_ALIGNMENT);
// Set up the buttons
playButton = new JButton("Play", new ImageIcon("play.gif"));
playButton.setBackground(Color.white);
playButton.setMnemonic('p');
stopButton = new JButton("Stop", new ImageIcon("stop.gif"));
stopButton.setBackground(Color.white);
stopButton.setMnemonic('s');
JPanel buttons = new JPanel();
buttons.setLayout(new BoxLayout(buttons, BoxLayout.X_AXIS));
buttons.add(playButton);
buttons.add(Box.createRigidArea(new Dimension(5,0)));
buttons.add(stopButton);
buttons.setBackground(Color.cyan);
// Set up this panel
setPreferredSize(new Dimension(300, 100));
setBackground(Color.cyan);
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
add(Box.createRigidArea(new Dimension(0,5)));
add(titleLabel);
add(Box.createRigidArea(new Dimension(0,5)));
add(musicCombo);
add(Box.createRigidArea(new Dimension(0,5)));
add(buttons);
add(Box.createRigidArea(new Dimension(0,5)));
musicCombo.addActionListener(new ComboListener());
stopButton.addActionListener(new ButtonListener());
playButton.addActionListener(new ButtonListener());
current = null;
}
//*****************************************************************
// Represents the action listener for the combo box.
//*****************************************************************
private class ComboListener implements ActionListener
{
//--------------------------------------------------------------
// Stops playing the current selection (if any) and resets
// the current selection to the one chosen.
//--------------------------------------------------------------
public void actionPerformed(ActionEvent event)
{
if (current != null)
current.stop();
current = music[musicCombo.getSelectedIndex()];
}
}
//*****************************************************************
// Represents the action listener for both control buttons.
//*****************************************************************
private class ButtonListener implements ActionListener
{
//--------------------------------------------------------------
// Stops the current selection (if any) in either case. If
// the play button was pressed, start playing it again.
//--------------------------------------------------------------
public void actionPerformed(ActionEvent event)
{
if (current != null)
current.stop();
if (event.getSource() == playButton)
if (current != null)
current.play();
}
}
}
Next go to the PowerPoint slides for chapter 11 Chap11.ppt and find the Java FX version (slide # 84 on) of the "Juke Box" program. Modify it so that it can play only two selections as in the previous problem where the swing and awt was used and modified by you to play only two selections.
import java.io.File;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ChoiceBox;
import javafx.scene.control.Label;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.media.AudioClip;
import javafx.stage.Stage;
//************************************************************************
// JukeBox.java Author: Lewis/Loftus
//
// Demonstrates the use of a combo box and audio clips.
//************************************************************************
public class JukeBox extends Application
{
private ChoiceBox
private AudioClip[] tunes;
private AudioClip current;
private Button playButton, stopButton;
continue
//--------------------------------------------------------------------
// Presents an interface that allows the user to select and play
// a tune from a drop down box.
//--------------------------------------------------------------------
public void start(Stage primaryStage)
{
String[] names = {"Western Beat", "Classical Melody",
"Jeopardy Theme", "Eighties Jam", "New Age Rythm",
"Lullaby", "Alfred Hitchcock's Theme"};
File[] audioFiles = {new File("westernBeat.wav"),
new File("classical.wav"), new File("jeopardy.mp3"),
new File("eightiesJam.wav"), new File("newAgeRythm.wav"),
new File("lullaby.mp3"), new File("hitchcock.wav")};
tunes = new AudioClip[audioFiles.length];
for (int i = 0; i
tunes[i] = new AudioClip(audioFiles[i].toURI().toString());
current = tunes[0];
Label label = new Label("Select a tune:");
continue
continue
choice = new ChoiceBox
choice.getItems().addAll(names);
choice.getSelectionModel().selectFirst();
choice.setOnAction(this::processChoice);
playButton = new Button("Play");
stopButton = new Button("Stop");
HBox buttons = new HBox(playButton, stopButton);
buttons.setSpacing(10);
buttons.setPadding(new Insets(15, 0, 0, 0));
buttons.setAlignment(Pos.CENTER);
playButton.setOnAction(this::processButtonPush);
stopButton.setOnAction(this::processButtonPush);
VBox root = new VBox(label, choice, buttons);
root.setPadding(new Insets(15, 15, 15, 25));
root.setSpacing(10);
root.setStyle("-fx-background-color: skyblue");
Scene scene = new Scene(root, 300, 150);
primaryStage.setTitle("Java Juke Box");
primaryStage.setScene(scene);
primaryStage.show();
}
continue
continue
//--------------------------------------------------------------------
// When a choice box selection is made, stops the current clip (if
// one was playing) and sets the current tune.
//--------------------------------------------------------------------
public void processChoice(ActionEvent event)
{
current.stop();
current = tunes[choice.getSelectionModel().getSelectedIndex()];
}
//--------------------------------------------------------------------
// Handles the play and stop buttons. Stops the current clip in
// either case. If the play button was pressed, (re)starts the
// current clip.
//--------------------------------------------------------------------
public void processButtonPush(ActionEvent event)
{
current.stop();
if (event.getSource() == playButton)
current.play();
}
}
continue
//--------------------------------------------------------------------
// When a choice box selection is made, stops the current clip (if
// one was playing) and sets the current tune.
//--------------------------------------------------------------------
public void processChoice(ActionEvent event)
{
current.stop();
current = tunes[choice.getSelectionModel().getSelectedIndex()];
}
//--------------------------------------------------------------------
// Handles the play and stop buttons. Stops the current clip in
// either case. If the play button was pressed, (re)starts the
// current clip.
//--------------------------------------------------------------------
public void processButtonPush(ActionEvent event)
{
current.stop();
if (event.getSource() == playButton)
current.play();
}
}
1. This programming challenge is also easier than CH_11A. Here you will modify the JukeBox program (Listing 11.11) JukeBox.zip so that the user can select one or the other of two.wav files to play. Both files are from operas and both are music associated with the arrival of guests. Specification: No more than 2 selections are to be available You can find the files in the folder CH11CProgChalleng-1.zip The first "entry of the guests music" is from Offenbach's 'Les contes d'Hoffmann' (English translation?????). It is played when guests arrive at the home of the scientist Spalanzani to see a demonstration of his latest invention, a robot. The robot Spalanzani has created is a human-like, life-size mechanical doll (Olympia). The other music is actually called "Entrance of the Guests" or "Entry of the Guests" and is from the opera???? by Richard Wagner. Here the "guests" arrive in the great Hall of the Warburg (a castle in Eisenach, Germany) for a singing contest. When you create the combo box (see p. 565) you should think about how event processing in a Java GUI relates to polymorphism (chapter 10, p. 521) and see if there is an opportunity for the use of polymorphism, since both pieces of music are associated with the arrival of guests and could use methods or interfaces that have the same name. For 20 extra points name the 2 operas (the ???? above) and the city and year where each had their premier. Put this information in the header annotation of your program. 2. Next go to the PowerPoint slides for chapter 11 Chap 11.ppta and find the Java FX version (slide # 84 on) of the uke Box" program. Modify it so that it can play only two selections as in the previous problem where the swing and awt was used and modified by vou to play only two selections 1. This programming challenge is also easier than CH_11A. Here you will modify the JukeBox program (Listing 11.11) JukeBox.zip so that the user can select one or the other of two.wav files to play. Both files are from operas and both are music associated with the arrival of guests. Specification: No more than 2 selections are to be available You can find the files in the folder CH11CProgChalleng-1.zip The first "entry of the guests music" is from Offenbach's 'Les contes d'Hoffmann' (English translation?????). It is played when guests arrive at the home of the scientist Spalanzani to see a demonstration of his latest invention, a robot. The robot Spalanzani has created is a human-like, life-size mechanical doll (Olympia). The other music is actually called "Entrance of the Guests" or "Entry of the Guests" and is from the opera???? by Richard Wagner. Here the "guests" arrive in the great Hall of the Warburg (a castle in Eisenach, Germany) for a singing contest. When you create the combo box (see p. 565) you should think about how event processing in a Java GUI relates to polymorphism (chapter 10, p. 521) and see if there is an opportunity for the use of polymorphism, since both pieces of music are associated with the arrival of guests and could use methods or interfaces that have the same name. For 20 extra points name the 2 operas (the ???? above) and the city and year where each had their premier. Put this information in the header annotation of your program. 2. Next go to the PowerPoint slides for chapter 11 Chap 11.ppta and find the Java FX version (slide # 84 on) of the uke Box" program. Modify it so that it can play only two selections as in the previous problem where the swing and awt was used and modified by vou to play only two selections
Step by Step Solution
There are 3 Steps involved in it
Step: 1
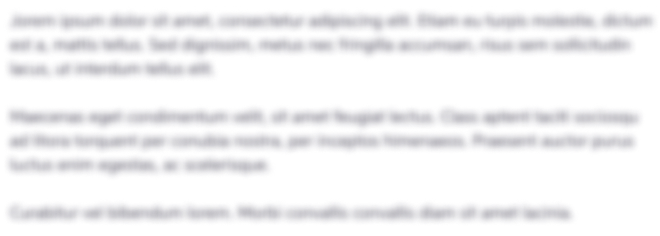
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started