Question
Please ask the user to input a low range and a high range and then print the range between them. Add printRang method to BST.java
Please ask the user to input a low range and a high range and then print the range between them.
Add printRang method to BST.java that, given a low key value, and high key value, print all records in a sorted order whose values fall between the two given keys from the inventory.txt file. (Both low key and high key do not have to be a key on the list).
****Please seperate the information in text file by product id, car_name, details, Price, etc
inventory.txt
GT12C1068A,YUKON XL,07-14 GMC YUKON XL RT Front fender brace Lower Bracket Hinge,24.00 NI27E1251B,ALTIMA SDN,13-15 NISSAN ALTIMA SDN RT Front fender liner From 10-12 (CAPA),63.00 NI23H1297A,ALTIMA SDN,13-15 NISSAN ALTIMA SDN LT Front fender liner From 10-12 (CAPA),48.00 CV15F1067A,SILVERADO 1500 (NEW),07-13 CHEVY SILVERADO 1500 LT Front fender brace Lower Bracket Hinge,23.00 HY07E1288A,SONATA,15-16 HYUNDAI SONATA Front bumper cover 2.4L Std Type w/o Park Assist prime (CAPA),326.00 CV20B1225B,SILVERADO 1500 HYBRID,09-13 CHEVY SILVERADO 1500 HYBRID LT Front fender brace Lower Bracket Hinge,23.00 CV39A1251A,AVALANCHE,07-13 CHEVY AVALANCHE RT Front fender brace Lower Bracket Hinge,24.00 CV39A1250A,SUBURBAN,07-14 CHEVY SUBURBAN RT Front fender brace Lower Bracket Hinge,24.00 AC12C1250AQ,MDX,07-13 ACURA MDX LT Front fender liner (CAPA),68.00 AC12C1251AQ,MDX,07-13 ACURA MDX RT Front fender liner (CAPA),68.00
BST.java
import java.lang.Comparable;
/** Binary Search Tree implementation for Dictionary ADT */ class BST
/** Constructor */ BST() { root = null; nodecount = 0; }
/** Reinitialize tree */ public void clear() { root = null; nodecount = 0; }
/** Insert a record into the tree. @param k Key value of the record. @param e The record to insert. */ public void insert(Key k, E e) { root = inserthelp(root, k, e); nodecount++; }
// Return root
public BSTNode getRoot() { return root; }
/** Remove a record from the tree. @param k Key value of record to remove. @return The record removed, null if there is none. */ public E remove(Key k) { E temp = findhelp(root, k); // First find it if (temp != null) { root = removehelp(root, k); // Now remove it nodecount--; } return temp; }
/** Remove and return the root node from the dictionary. @return The record removed, null if tree is empty. */ public E removeAny() { if (root == null) return null; E temp = root.element(); root = removehelp(root, root.key()); nodecount--; return temp; }
/** @return Record with key value k, null if none exist. @param k The key value to find. */ public E find(Key k) { return findhelp(root, k); }
/** @return The number of records in the dictionary. */ public int size() { return nodecount; } private E findhelp(BSTNode
private BSTNode
private BSTNode
private StringBuffer out;
public String toString() { out = new StringBuffer(400); printhelp(root); return out.toString(); } private void printVisit(E it) { out.append(it + " "); }
}
BSTNode.java
class BSTNode
/** Constructors */ public BSTNode() {left = right = null; } public BSTNode(Key k, E val) { left = right = null; key = k; element = val; } public BSTNode(Key k, E val, BSTNode
/** Get and set the key value */ public Key key() { return key; } public void setKey(Key k) { key = k; }
/** Get and set the element value */ public E element() { return element; } public void setElement(E v) { element = v; }
/** Get and set the left child */ public BSTNode
/** Get and set the right child */ public BSTNode
/** @return True if a leaf node, false otherwise */ public boolean isLeaf() { return (left == null) && (right == null); } }
BinNode.java
/** ADT for binary tree nodes */ public interface BinNode
/** @return The left child */ public BinNode
/** @return The right child */ public BinNode
/** @return True if a leaf node, false otherwise */ public boolean isLeaf(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
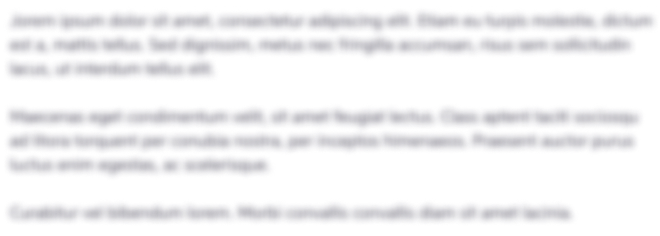
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started