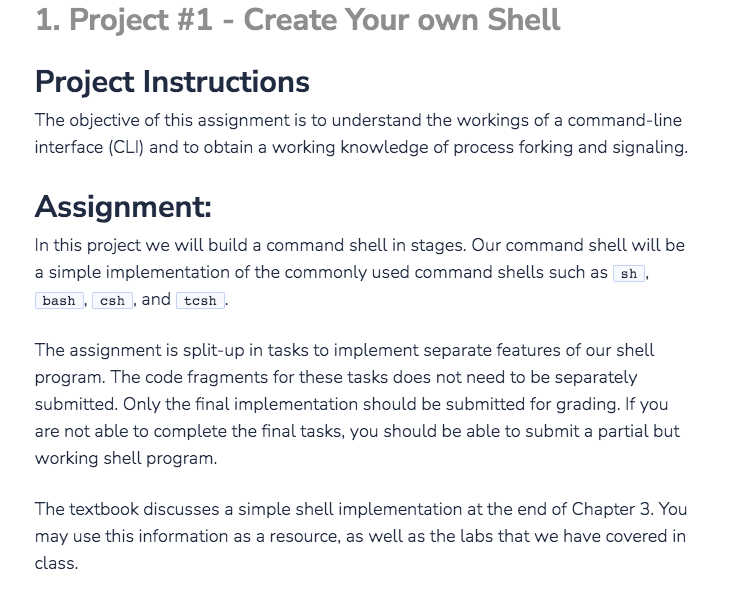
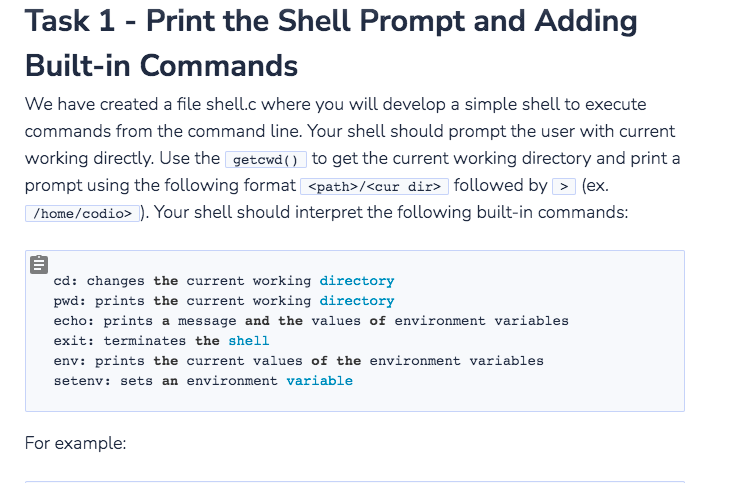
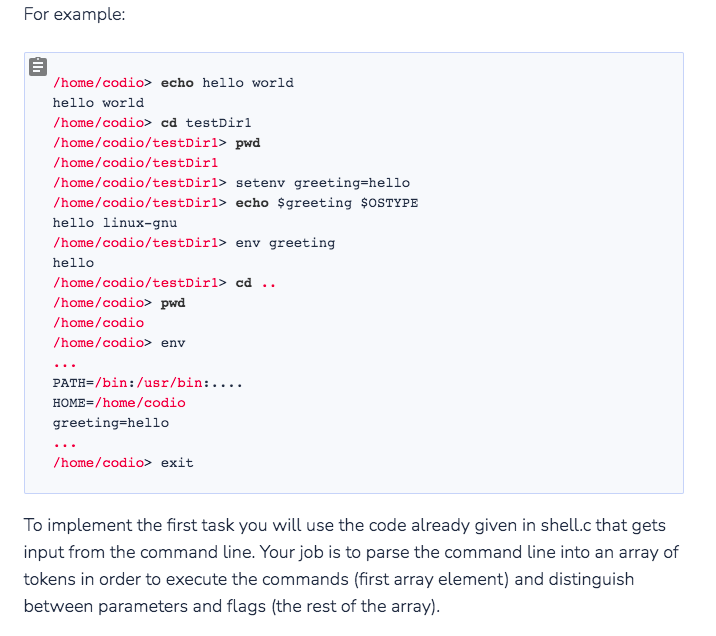
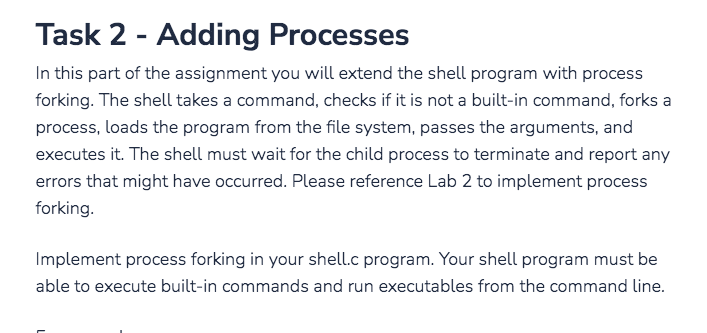
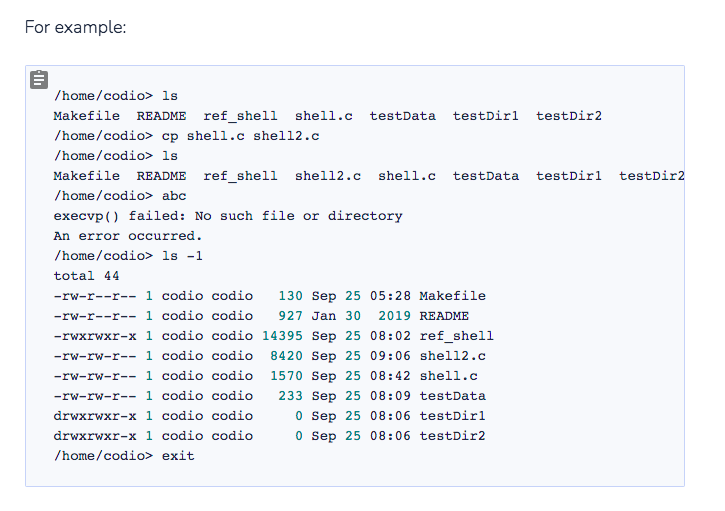
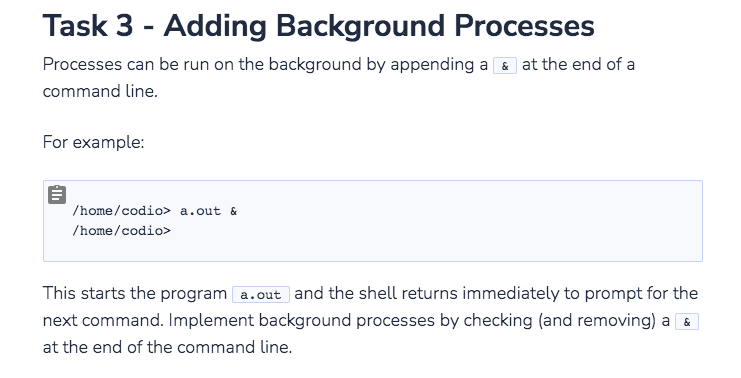
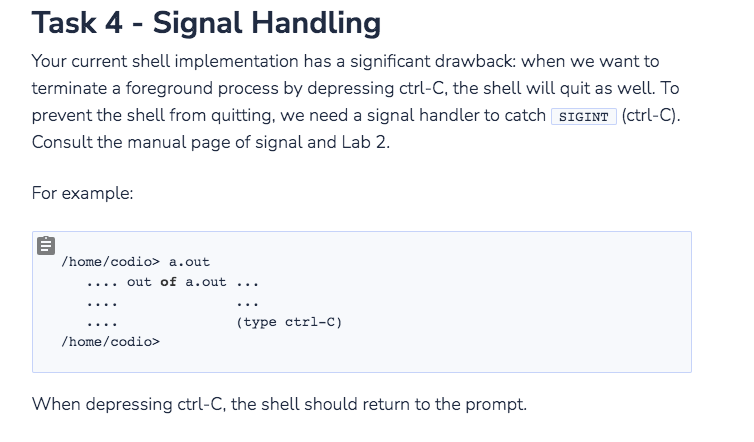
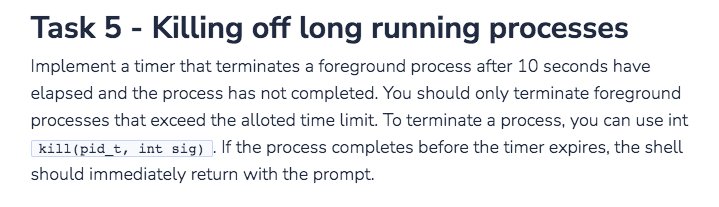
Please code in C
1. Project #1 - Create Your own Shell Project Instructions The objective of this assignment is to understand the workings of a command-line interface (CLI) and to obtain a working knowledge of process forking and signaling. Assignment: In this project we will build a command shell in stages. Our command shell will be a simple implementation of the commonly used command shells such as sh , bash , csh , and tesh. The assignment is split-up in tasks to implement separate features of our shell program. The code fragments for these tasks does not need to be separately submitted. Only the final implementation should be submitted for grading. If you are not able to complete the final tasks, you should be able to submit a partial but working shell program. The textbook discusses a simple shell implementation at the end of Chapter 3. You may use this information as a resource, as well as the labs that we have covered in class. Task 1 - Print the Shell Prompt and Adding Built-in Commands We have created a file shell.c where you will develop a simple shell to execute commands from the command line. Your shell should prompt the user with current working directly. Use the getcwd() to get the current working directory and print a prompt using the following format
/ followed by > (ex. /home/codio> ). Your shell should interpret the following built-in commands: cd: changes the current working directory pwd: prints the current working directory echo: prints a message and the values of environment variables exit: terminates the shell env: prints the current values of the environment variables seteny: sets an environment variable For example: For example: /home/codio> echo hello world hello world /home/codio> cd testDirl /home/codio/testDirl> pwd /home/codio/testDiri /home/codio/testDirl> setenv greeting=hello /home/codio/testDiri> echo $greeting $OSTYPE hello linux-gnu /home/codio/testDirl> env greeting hello /home/codio/testDirl> cd .. /home/codio> pwd /home/codio /home/codio> env PATH=/bin:/usr/bin:.... HOME=/home/codio greeting=hello /home/codio> exit To implement the first task you will use the code already given in shell.c that gets input from the command line. Your job is to parse the command line into an array of tokens in order to execute the commands (first array element) and distinguish between parameters and flags (the rest of the array). Task 2 - Adding Processes In this part of the assignment you will extend the shell program with process forking. The shell takes a command, checks if it is not a built-in command, forks a process, loads the program from the file system, passes the arguments, and executes it. The shell must wait for the child process to terminate and report any errors that might have occurred. Please reference Lab 2 to implement process forking. Implement process forking in your shell.c program. Your shell program must be able to execute built-in commands and run executables from the command line. For example: /home/codio> ls Makefile README ref_shell shell.c testData testDiri testDir2 /home/codio> cp shell.c shell2.c /home/codio> ls Makefile README ref_shell shel12.c shell.c testData testDiri testDir2 /home/codio> abc execvp() failed: No such file or directory An error occurred. /home/codio> ls -1 total 44 -rw-r--r-- 1 codio codio 130 Sep 25 05:28 Makefile -rw-r--r-- 1 codio codio 927 Jan 30 2019 README -rwxrwxr-x 1 codio codio 14395 Sep 25 08:02 ref_shell -rw-rw-r-- 1 codio codio 8420 Sep 25 09:06 shell2.c -rw-rw-r-- 1 codio codio 1570 Sep 25 08:42 shell.c -rw-rw-r-- 1 codio codio 233 Sep 25 08:09 testData drwxrwxr-x 1 codio codio 0 Sep 25 08:06 testDiri drwxrwxr-x 1 codio codio 0 Sep 25 08:06 testDir2 /home/codio> exit Task 3 - Adding Background Processes Processes can be run on the background by appending a & at the end of a command line. For example: /home/codio> a.out & /home/codio> This starts the program a.out and the shell returns immediately to prompt for the next command. Implement background processes by checking (and removing) a & at the end of the command line. Task 4 - Signal Handling Your current shell implementation has a significant drawback: when we want to terminate a foreground process by depressing ctrl-C, the shell will quit as well. To prevent the shell from quitting, we need a signal handler to catch SIGINT (ctrl-C). Consult the manual page of signal and Lab 2. For example: /home/codio> a.out ... out of a.out (type ctrl-c) /home/codio> When depressing ctrl-C, the shell should return to the prompt. Task 5 - Killing off long running processes Implement a timer that terminates a foreground process after 10 seconds have elapsed and the process has not completed. You should only terminate foreground processes that exceed the alloted time limit. To terminate a process, you can use int kill(pid_t, int sig). If the process completes before the timer expires, the shell should immediately return with the prompt. 1. Project #1 - Create Your own Shell Project Instructions The objective of this assignment is to understand the workings of a command-line interface (CLI) and to obtain a working knowledge of process forking and signaling. Assignment: In this project we will build a command shell in stages. Our command shell will be a simple implementation of the commonly used command shells such as sh , bash , csh , and tesh. The assignment is split-up in tasks to implement separate features of our shell program. The code fragments for these tasks does not need to be separately submitted. Only the final implementation should be submitted for grading. If you are not able to complete the final tasks, you should be able to submit a partial but working shell program. The textbook discusses a simple shell implementation at the end of Chapter 3. You may use this information as a resource, as well as the labs that we have covered in class. Task 1 - Print the Shell Prompt and Adding Built-in Commands We have created a file shell.c where you will develop a simple shell to execute commands from the command line. Your shell should prompt the user with current working directly. Use the getcwd() to get the current working directory and print a prompt using the following format / followed by > (ex. /home/codio> ). Your shell should interpret the following built-in commands: cd: changes the current working directory pwd: prints the current working directory echo: prints a message and the values of environment variables exit: terminates the shell env: prints the current values of the environment variables seteny: sets an environment variable For example: For example: /home/codio> echo hello world hello world /home/codio> cd testDirl /home/codio/testDirl> pwd /home/codio/testDiri /home/codio/testDirl> setenv greeting=hello /home/codio/testDiri> echo $greeting $OSTYPE hello linux-gnu /home/codio/testDirl> env greeting hello /home/codio/testDirl> cd .. /home/codio> pwd /home/codio /home/codio> env PATH=/bin:/usr/bin:.... HOME=/home/codio greeting=hello /home/codio> exit To implement the first task you will use the code already given in shell.c that gets input from the command line. Your job is to parse the command line into an array of tokens in order to execute the commands (first array element) and distinguish between parameters and flags (the rest of the array). Task 2 - Adding Processes In this part of the assignment you will extend the shell program with process forking. The shell takes a command, checks if it is not a built-in command, forks a process, loads the program from the file system, passes the arguments, and executes it. The shell must wait for the child process to terminate and report any errors that might have occurred. Please reference Lab 2 to implement process forking. Implement process forking in your shell.c program. Your shell program must be able to execute built-in commands and run executables from the command line. For example: /home/codio> ls Makefile README ref_shell shell.c testData testDiri testDir2 /home/codio> cp shell.c shell2.c /home/codio> ls Makefile README ref_shell shel12.c shell.c testData testDiri testDir2 /home/codio> abc execvp() failed: No such file or directory An error occurred. /home/codio> ls -1 total 44 -rw-r--r-- 1 codio codio 130 Sep 25 05:28 Makefile -rw-r--r-- 1 codio codio 927 Jan 30 2019 README -rwxrwxr-x 1 codio codio 14395 Sep 25 08:02 ref_shell -rw-rw-r-- 1 codio codio 8420 Sep 25 09:06 shell2.c -rw-rw-r-- 1 codio codio 1570 Sep 25 08:42 shell.c -rw-rw-r-- 1 codio codio 233 Sep 25 08:09 testData drwxrwxr-x 1 codio codio 0 Sep 25 08:06 testDiri drwxrwxr-x 1 codio codio 0 Sep 25 08:06 testDir2 /home/codio> exit Task 3 - Adding Background Processes Processes can be run on the background by appending a & at the end of a command line. For example: /home/codio> a.out & /home/codio> This starts the program a.out and the shell returns immediately to prompt for the next command. Implement background processes by checking (and removing) a & at the end of the command line. Task 4 - Signal Handling Your current shell implementation has a significant drawback: when we want to terminate a foreground process by depressing ctrl-C, the shell will quit as well. To prevent the shell from quitting, we need a signal handler to catch SIGINT (ctrl-C). Consult the manual page of signal and Lab 2. For example: /home/codio> a.out ... out of a.out (type ctrl-c) /home/codio> When depressing ctrl-C, the shell should return to the prompt. Task 5 - Killing off long running processes Implement a timer that terminates a foreground process after 10 seconds have elapsed and the process has not completed. You should only terminate foreground processes that exceed the alloted time limit. To terminate a process, you can use int kill(pid_t, int sig). If the process completes before the timer expires, the shell should immediately return with the prompt