Question
please Code in c++ Create a new Library class. You will need both a header file and a source file for this class. The class
please Code in c++
- Create a new Library class. You will need both a header file and a source file for this class. The class will contain two data members:
- an array of Book objects
- the current number of books in the array Since the book array is moving from the main.cc file, you will also move the constant array size definition (MAX_ARR_SIZE) into the Library header file.
- Write the following functions for the Library class:
- a constructor that initializes the data member(s) that require initialization; think about what these might be
- an addBook(Book&) function that adds the given book parameter to the back of the book array terminology: the back of a collection is its end; the front of a collection is its beginning
- a print() function that prints out all the books in the array to the screen
- Change the program so that the main() function:
- doesnt declare a book array anymore; instead, it will declare a Library object
- uses the Library object and its functions, instead of manipulating the book array directly
- creates temporary Book objects to be added to the library
the Book classs setBook() function should no longer be used and should be removed adds the new book to the library using functions implemented in step #3
- Update the Makefile so that the new Library class gets compiled and linked into the executable, as we saw in the course material section on Makefiles.
- Build and run the program. Check that the book information is correct when the library is printed out at the end of the program.
Book.h
#define BOOK_H
#define BOOK_H
#include
using namespace std;
class Book
{
public:
Book(int=0, string="Unknown", string="Unknown", int=0);
void setBook(int, string, string, int);
void print();
private:
int id;
string title;
string author;
int year;
};
#endif
Book.cc
#include
#include
using namespace std;
#include "Book.h"
Book::Book(int i, string t, string a, int y)
{
id = i;
title = t;
author = a;
year = y;
}
void Book::setBook(int i, string t, string a, int y)
{
id = i;
title = t;
author = a;
year = y;
}
void Book::print()
{
cout << setw(3) << id
<<" Title: " << setw(40) << title
<<"; Author: " << setw(20) << author
<<"; Year: " << year << endl;
}
main.cc
#include
using namespace std;
#include
#include "Book.h"
#define MAX_ARR_SIZE 128
int mainMenu();
void printLibrary(Book arr[MAX_ARR_SIZE], int num);
int main()
{
Book library[MAX_ARR_SIZE];
int numBooks = 0;
string title, author;
int id, year;
int menuSelection;
while (1) {
menuSelection = mainMenu();
if (menuSelection == 0)
break;
else if (menuSelection == 1) {
cout << "id: ";
cin >> id;
cout << "title: ";
cin.ignore();
getline(cin, title);
cout << "author: ";
getline(cin, author);
cout << "year: ";
cin >> year;
library[numBooks].setBook(id, title, author, year);
++numBooks;
}
}
if (numBooks > 0)
printLibrary(library, numBooks);
return 0;
}
int mainMenu()
{
int numOptions = 1;
int selection = -1;
cout << endl;
cout << "(1) Add book" << endl;
cout << "(0) Exit" << endl;
while (selection < 0 || selection > numOptions) {
cout << "Enter your selection: ";
cin >> selection;
}
return selection;
}
void printLibrary(Book arr[MAX_ARR_SIZE], int num)
{
cout << endl << endl << "LIBRARY: " << endl;
for (int i=0; i arr[i].print(); cout << endl; } Makefile OPT = -Wall t01: main.o Book.o g++ $(OPT) -o t01 main.o Book.o main.o: main.cc Book.h g++ $(OPT) -c main.cc Book.o: Book.cc Book.h g++ $(OPT) -c Book.cc clean: rm -f *.o t01 in.txt 1 101 Ender's Game Card, Orson Scott 1985 1 102 Dune Herbert, Frank 1965 1 110 Foundation Asimov, Isaac 1951 1 111 Hitch Hiker's Guide to the Galaxy Adams, Douglas 1979 1 112 1984 Orwell, George 1949 1 113 Stranger in a Strange Land Heinlein, Robert A. 1961 1 114 Farenheit 451 Bradbury, Ray 1954 1 115 2001: A Space Odyssey Clarke, Arthur C. 1968 1 116 I, Robot Asimov, Isaac 1950 1 117 Starship Troopers Heinlein, Robert A. 1959 1 118 Do Androids Dream of Electric Sheep? Dick, Philip K. 1968 1 119 Neuromancer Gibson, William 1984 1 120 Ringworld Niven, Larry 1970 1 121 Rendezvous with Rama Clarke, Arthur C. 1973 1 122 Hyperion Simmons, Dan 1989 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
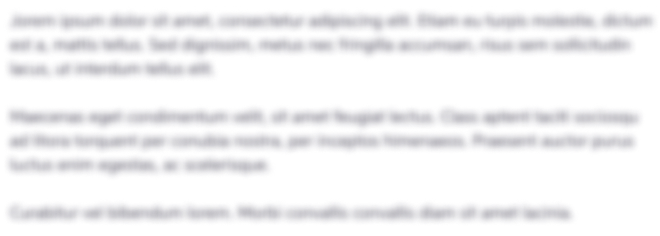
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started