Question
Please code in C# Source Code: customer.cs- using System; using System.Collections.Generic; using System.IO; using System.Threading.Tasks; namespace CustomerMaintenance { public class Customer { public Customer() {
Please code in C#
Source Code:
customer.cs-
using System; using System.Collections.Generic; using System.IO; using System.Threading.Tasks;
namespace CustomerMaintenance { public class Customer { public Customer() { }
public Customer(string firstName, string lastName, string email) => (this.FirstName, this.LastName, this.Email) = (firstName, lastName, email);
public string FirstName { get; set; }
public string LastName { get; set; }
public string Email { get; set; }
public string GetDisplayText() => FirstName + " " + LastName + ", " + Email; } }
Exercise 13-1 Create a Customer Maintenance application that uses classes In this exercise, you'll create a Customer Maintenance application that uses classes with the features presented in this chapter. To make this application easier to develop, we'll give you the starting forms and classes. Open the project and add validation code to the Customer class 1. Open the application in the C:\C#\Chapter 13\CustomerMaintenance direc- tory. 2. Open the Customer class and note how the constructor for this class sets the properties to the appropriate parameters. Then, add code to the set accessors for the FirstName, LastName, and Email properties that throws an exception if the value is longer than 30 characters. 3. Test the class by trying to add a new customer with an email address that's longer than 30 characters. When you do, an exception should be thrown. Then, end the application. 4. Set the MaxLength properties of the First Name, Last Name, and Email text boxes to 30. Then, run the application again and try to add a new customer with an email address that's longer than 30 characters. This shows one way to avoid the exceptions, but your classes should still throw them in case they are used by other applications that don't avoid them. Add a Cust 5. Add a class named CustomerList to the project, and declare a private variable that can store a list of Customer objects. 6. Add the following members to the CustomerList class, coding Count as expression-bodied property and Fill() and Save() as expression-bodied an methods: Constructor Indexer [index] Property Count Description Creates a new list of Customer objects. Description Provides access to the Customer at the specified position. Description An integer that indicates how many Customer objects are in the list. Description Adds the specified Customer object to the list. Removes the specified Customer object from the list. Fills the list with customer data from a file using the GetCustomers() method of the Customer DB class. Saves the customers to a file using the SaveCustomers method of the CustomerDB class. Method Add (customer) Remove (customer) Fill() Save() 7. Modify the Customer Maintenance form to use this class. To do that, you'll need to use the Fill() and Save() methods of the CustomerList object instead of the methods of the CustomerDB class. In addition, you'll need to use a for loop instead of a foreach loop when you fill the list box. 8. Run the application and test it to be sure it works properly. Exercise 13-2 Use overloaded operators In this exercise, you'll enhance the Customer Maintenance application from exercise 13-1 so it uses overloaded operators to add and remove customers from the customer list. 1. If it isn't open already, open the application in the C:\C#\Chapter 13\Customer Maintenance directory 2. Add overloaded + and - operators to the Customer List class that add and remove a customer from the customer list. 3. Modify the Customer Maintenance form to use these operators instead of the Add() and Remove() methods. 4. Run and test the application to make sure it works correctly a Exercise 13-3 Use delegates and events In this exercise, you'll enhance the Customer Maintenance application from exercise 13-2 so it uses a delegate and an event to handle changes to the cus. tomer list. 1. If it's not open already, open the application in the C:\C#\Chapter 13\CustomerMaintenance directory. 2. Add a delegate named ChangeHandler to the CustomerList class. This delegate should specify a method with a void return type and a Customer List parameter as described in figure 13-6. 3. Add an event named Changed to the Customer List class. This event should use the ChangeHandler delegate and should be raised any time the customer list changes as described in figure 13-7. 4. Modify the Customer Maintenance form to use the Changed event to save the customers and refresh the list box any time the list changes. To do that, you'll need to code an event handler that has the signature specified by the delegate, you'll need to wire the event handler to the event, and you'll need to remove any unnecessary code from the event handlers for the Add and Delete buttons. 5. Run and test the application. 6. Modify the statement that wires an event handler to the event so it uses a lambda expression as shown in figure 13-8. The lambda expression should execute the same two statements as the named method that you created for the event handler in step 4. 7. Run and test the application one more time. If this works, delete the named method that was used previously for the event handler
Step by Step Solution
There are 3 Steps involved in it
Step: 1
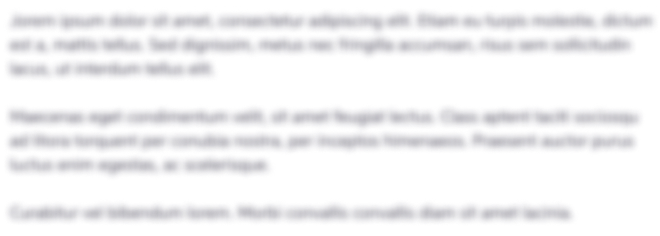
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started