Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please complete, or thoroughly explain this C++ lab...Thank you! Instructions: In class, we implemented a list using a dynamic array. For lab, you are going
Please complete, or thoroughly explain this C++ lab...Thank you!
Instructions: In class, we implemented a list using a dynamic array. For lab, you are going to expand the functionality of the class by adding a function reverse that, amazingly enough, reverses the order of the items in a list. You may implement this any way you wish, but it must be a member function of the list class.
- The header and implementation files are given to you, you only have to add the new function.
- Your driver file should create a list, give it values, display it, reverse the list, and then display it again
- I do NOT want the function to just display the list in reverse order, I want it to actually reverse the order.
______________________________________________________________
/*-- DList.cpp----------------------------------------------------------- This file implements List member functions. -------------------------------------------------------------------------*/ #include#include using namespace std; #include "DList.h" //--- Definition of class constructor List::List(int maxSize) : mySize(0), myCapacity(maxSize) { myArray = new(nothrow) ElementType[maxSize]; assert(myArray != 0); } //--- Definition of class destructor List::~List() { cout << "In the destructor "; delete [] myArray; } //--- Definition of copy constructor List::List(const List & origList) : mySize(origList.mySize), myCapacity(origList.myCapacity) { //--- Get new array for copy myArray = new(nothrow) ElementType[myCapacity]; if (myArray != 0) // check if memory available //--- Copy origList's elements into this new array for(int i = 0; i < mySize; i++) myArray[i] = origList.myArray[i]; else { cerr << "*** Inadequate memory to allocate storage for list *** "; exit(1); } } //--- Definition of assignment operator const List & List::operator=(const List & rightHandSide) { if (this != &rightHandSide) // check that not self-assignment { //-- Allocate a new array if necessary if (myCapacity != rightHandSide.myCapacity) { delete[] myArray; myCapacity = rightHandSide.myCapacity; myArray = new(nothrow) ElementType[myCapacity]; if (myArray == 0) // check if memory available { cerr << "*Inadequate memory to allocate stack *** "; exit(1); } } //--- Copy rightHandSide's list elements into this new array mySize = rightHandSide.mySize; for(int i = 0; i < mySize; i++) myArray[i] = rightHandSide.myArray[i]; } return *this; } //--- Definition of empty() bool List::empty() const { return mySize == 0; } //--- Definition of display() void List::display(ostream & out) const { for (int i = 0; i < mySize; i++) out << myArray[i] << " "; } //--- Definition of output operator ostream & operator<< (ostream & out, const List & aList) { aList.display(out); return out; } //--- Definition of insert() void List::insert(ElementType item, int pos) { if (pos < 0 || pos > mySize) { cerr << "*** Illegal location to insert -- " << pos << ". List unchanged. *** "; return; } if (mySize == myCapacity) { cout << "Increasing list capacity "; myCapacity += 5; ElementType *newarray = new (nothrow) ElementType[myCapacity]; for (int i = 0; i < mySize; i++) newarray[i] = myArray[i]; delete [] myArray; myArray = newarray; } // First shift array elements right to make room for item for(int i = mySize; i > pos; i--) myArray[i] = myArray[i - 1]; // Now insert item at position pos and increase list size myArray[pos] = item; mySize++; } //--- Definition of erase() void List::erase(int pos) { if (mySize == 0) { cerr << "*** List is empty *** "; return; } if (pos < 0 || pos >= mySize) { cerr << "Illegal location to delete -- " << pos << ". List unchanged. *** "; return; } // Shift array elements left to close the gap for(int i = pos; i < mySize; i++) myArray[i] = myArray[i + 1]; // Decrease list size mySize--; }
__________________________________________________________________
/*-- DList.h -------------------------------------------------------------- This header file defines the data type List for processing lists. Basic operations are: Constructor Destructor Copy constructor Assignment operator empty: Check if list is empty insert: Insert an item erase: Remove an item display: Output the list << : Output operator -------------------------------------------------------------------------*/ #include#ifndef DLIST #define DLIST typedef int ElementType; class List { public: /******** Function Members ********/ /***** Class constructor *****/ List(int maxSize = 1024); /*---------------------------------------------------------------------- Construct a List object. Precondition: maxSize is a positive integer with default value 1024. Postcondition: An empty List object is constructed; myCapacity == maxSize (default value 1024); myArray points to a dynamic array with myCapacity as its capacity; and mySize is 0. -----------------------------------------------------------------------*/ /***** Class destructor *****/ ~List(); /*---------------------------------------------------------------------- Destroys a List object. Precondition: The life of a List object is over. Postcondition: The memory dynamically allocated by the constructor for the array pointed to by myArray has been returned to the heap. -----------------------------------------------------------------------*/ /***** Copy constructor *****/ List(const List & origList); /*---------------------------------------------------------------------- Construct a copy of a List object. Precondition: A copy of origList is needed; origList is a const reference parameter. Postcondition: A copy of origList has been constructed. -----------------------------------------------------------------------*/ /***** Assignment operator *****/ const List & operator=(const List & rightHandSide); /*---------------------------------------------------------------------- Assign a copy of a List object to the current object. Precondition: none Postcondition: A copy of rightHandSide has been assigned to this object. A const reference to this list is returned. -----------------------------------------------------------------------*/ /***** empty operation *****/ bool empty() const; //--- See Figure 6.1 for documentation /***** insert and erase *****/ void insert(ElementType item, int pos); //--- See Figure 6.1 for documentation (replace CAPACITY with myCapacity) void erase(int pos); //--- See Figure 6.1 for documentation /***** output *****/ void display(ostream & out) const; //--- See Figure 6.1 for documentation private: /******** Data Members ********/ int mySize; // current size of list int myCapacity; // capacity of array ElementType * myArray; // pointer to dynamic array }; //--- end of List class //------ Prototype of output operator ostream & operator<< (ostream & out, const List & aList); #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
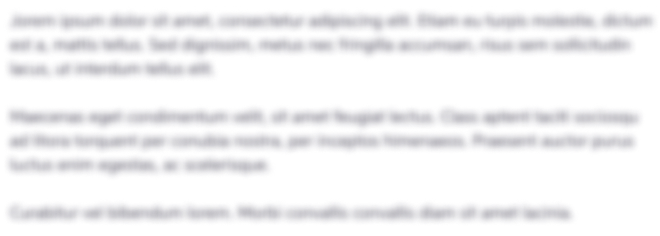
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started