Question
please complete the following code : from cImage import * import cluster import sys Your task for this assignment is to complete the application
please complete the following code :
from cImage import * import cluster import sys
""" Your task for this assignment is to complete the application that takes an original image and creates a new k-coloured image and saves it.
NOTE - Do not modify cluster.py or cImage.py """
def create_centroids(k, image, image_window): # This function asks the user to select k pixels with distinct RGB # values from the image and store them as centroids. Note that the # way you store these centroids is completely up to you. You can store # the pixel objects, store their (R,G,B) tuple values, etc. # Let the user click on k points of the image, store them as # centroids, and print x and y coordinates of them in k lines. If a # selected point has the same RGB values as one of the previous ones, # don't accept it and wait for another one. You can display a message # and ask the user to input another pixel. user = input("select 4 centroids by clicking on the image") centroids = input ....
def create_data_file(image): # This function creates a dictionary with image pixels as the values # and returns the dataFile dictionary. Note that the data structure # you use to store points is up to you (I used dictionary) dic = {} datafiledic = create_centroids(image) return datafiledic
def create_new_image(clusters, centroids, width, height): # This function creates a new image (You can check Chapter 6, page 192 # of your textbook to get a hint) where each pixel has the colour # value of its centroid (use the round() function for each # RGB value) and returns the new image. # Hint: The size of the new image should be the same as the old one. # So you can use width and height arguments to create such an image # with the same size. pass
def compute_distance(clusters, centroids, image): # This function computes the total distance and returns its value # which has been rounded to 2 digits beyond the decimal point in form # of a string. # The total distance is defined as the sum of all distances between # points and their centroids. # NOTE: you should round the total result to two digits of precision # beyond the decimal point. total_distance = msmb return total_distance,"%f.2"
def display_image(imagename): # This function opens the image whose name is imagename and # displays it in a window which is the same size as the image # It also returns the image object as well as the window object. #img = None #win = None img = open(imagename) win = ImageWin(img) return img, win
def readCommandLineArgs(): # Process the command line arguments. These arguments are the name # of the original image and the number of clusters (k). Consider 6 # as the default value of k. The user will enter them after the name # of your program file in command line k = 6 iterations = 5 inputFile = sys.argv[1]
return inputFile, k, iterations
def main(): # TODO read and Process the command line arguments. (mandrill.gif, 6, 5) = readCommandLineArgs()
# TODO Read in the original image and display it. Note that when you # displayed the image in display_image function, you should return the # image object as well as the window object you created in # display_image function. imagename = "mandrill.gif" image = FileImage(imagename) image_window = ImageWin('img',image.getWidth(),image.getHeight()) #image, image_window = display_image(.....) image, image_window = display_image(imagename) # TODO Get width and height of the original image. width = image.getWidth() height = image.getHeight()
# TODO Let the user click on k points of the image, store them as # centroids, and print x and y coordinates of them in k lines. If a # selected point has the same RGB values as one of the previous ones, # don't accept it and wait for another one. # image_centroids = create_centroids( ... ) image_centroids = create_centroids(k,image,image_window) # TODO Create data_file which is a dictionary with pixel indexes and also # the the pixels as the values of the dictionary. # data_file = create_data_file( ... ) data_file = create_data_file(image) new_image = None counter = 0 while counter < iterations: # TODO Partition the color values in the image. That is, each pixel is # grouped in the cluster whose centroid it is closest to. # Hint: use cluster.py
# clusters = ... clusters = assignPointsToClusters.cluster(image) # TODO Create a new image where each pixel has the colour value of its # centroid. # new_image = create_new_image( ... ) new_image = create_new_image(clusters,centroids,width,height) # TODO Display the new image. # new_image.draw(image_window) new_image.draw(image_window)
# TODO Print the total distance rounded to two digits of precision # beyond the decimal point. Also NOTE that we are passing the # original image to computeDistance as the argument (NOT the # new_image we created previously)
#distance = compute_distance( ... ) distance = compute_distance(image) print(distance, '%f.2') #print( ... )
# TODO Compute new centroids based on the clusters. # Hint: use cluster.py #image_centroids = ... image_centroids = updateCentroids.cluster counter += 1 #TODO save image to new file k_colours.gif new_image = "k_colours.giff"
if __name__ == "__main__": main()
#####
o reiterate, the basic process of computing a new k-coloured image is:
Process the command line arguments. These arguments are the name of the original image, the number of clusters (k), and the number of iterations the algorithm will run. Consider 6 as the default value of k and 5 for the number of iterations. The user will enter them after the name of your program file in command line.
Read in the original image and display it.
Let the user click on k points of the image, store them as centroids, and print x and y coordinates of them in k lines, followed by a message like: "Starting the clustering process" on a new line. If a selected point has the same RGB values as one of the previous ones, don't accept it and wait for another one.
Partition the color values in the image. That is, each pixel is grouped in the cluster whose centroid it is closest to.
Create a new image where each pixel has the colour value of its centroid (use the round() function for each RGB value).
Display the new image.
Print the total distance rounded to two digits of precision beyond the decimal point.
Compute new centroids based on the clusters.
Repeat steps 4 - 8 for the number of iterations from part 1.
Save new image to file named k_colours.gif
Your Task
Your task for this assignment is to complete the application that takes an original image and creates a new k-coloured image. You can recycle any code from previous class examples, labs, and assignments to complete the assignment. We have provided a skeleton file which you can use to write your code. Note that using the skeleton files is optional and you can implement your own code. How you implement this assignment is up to you, but you will need to add the following functionality:
Write a program that does K-Means clustering. You can use the cluster.py module but do not modify it. You can also use any other code from the textbook/class as a starting point.
Use the cImage.py module to implement any image-specific functionality required to complete the assignment (i.e., read from file, display, get a pixel's RGB values, write new pixels, etc.). Do not edit cImage.py.
Glue together the pieces to complete the process outlined above.
You can get the skeleton files by downloading the Assignment3.zip file.
Some Important Details
1. When selecting the centroid closest to a given point, ties may arise. When they do, you should break ties by choosing the first centroid in the data structure that you keep your centroids, with the minimum distance to the given point.
2. Instead of running your program from Wing IDE, which may cause an error, run it from the command line.
3. You need to create only one window in the first pass and display the resulting image of each pass in it. Do not create five separate windows.
4. Do not modify cluster.py or cImage.py.
5. You don't need to handle cases where invalid image file names are passed from the command line (ie, files that don't exist, files that are not images)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
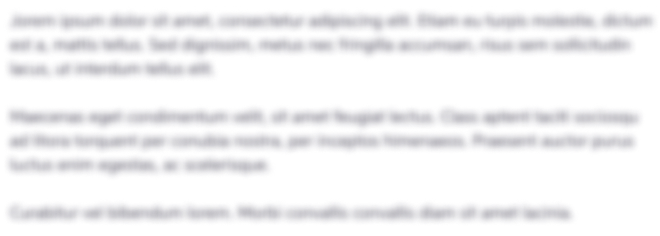
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started