Question
Please complete the last two methods. /** * This class allows singly-linked lists to be constructed and manipulated */ public class LinkedIntList { private ListNode
Please complete the last two methods.
/** * This class allows singly-linked lists to be constructed and manipulated */ public class LinkedIntList { private ListNodefront; private int size; //O(1) /** * Constructs a LinkedIntList with no nodes, front is set to null */ public LinkedIntList() { front = null; size = 0; } /** * Constructs a LinkedIntList and sets the front to the head ListNode * passed to it. Note: head may already be connected to other ListNodes * * @param head ListNode to become the front of the linked list */ public LinkedIntList(ListNode head) { front = head; size = size2(); } /** * Constructs a singly linked list with one ListNode storing an integer * value * * @param value data value to store in the ListNode */ public LinkedIntList(int value) { front = new ListNode(value, null); size = 1; } /** * Adds a new list node to the front of the singly linked list * * @param value Note: You need to change the front instance field reference * to point at this new node */ public void addFront(int value) { if (front == null) { front = new ListNode(value, null); } else { front = new ListNode(value, front); } size++; } /** * Add a list node to the end of the singly linked list * * @param value integer value to add */ public void addEnd(int value) { if (front == null) { addFront(value); } else { ListNode curr = front; while (curr.next != null) { curr = curr.next; } curr.next = new ListNode(value); size++; } } /** * Removes the first node in the list and returns the data value * stored in the removed node * * @return integer data value that was removed * * Note: if front is null, throw an IllegalStateException("No node to remove"); */ public int removeFront() { if (front == null) { throw new IllegalStateException("no node to remove."); } else { int removed = front.data; front = front.next; size--; return removed; } } /** * Traverses to the NEXT to last node and removes the last list node * in the singly linked list by setting current.next to null. Make sure you * save the removed value before setting .next to null, so you can return it. * * @return integer data value that was removed * * Note: if front is null, throw an IllegalStateException("No node to remove"); */ public int removeEnd() { if (front == null) { throw new IllegalStateException("no node to remove"); } int removed = front.data; if (front.next == null) { front = null; } else { ListNode
curr = front; while (curr.next.next != null) { curr = curr.next; } removed = curr.next.data; curr.next = null; } size--; return removed; } /** * Determines if the value is in a list node in the singly linked list * @param value integer data value to search for * @return true if value is found, false otherwise */ public boolean contains(int value) { ListNode current = front; while (current != null) { if (current.data == value) { return true; } current = current.next; } return false; } /** * This size2 method has an O(n) time complexity since it traverses the entire * list to count the number of nodes * @return the number of list nodes */ public int size2() { int count = 0; ListNode curr = front; while (curr != null) { curr = curr.next; count++; } return count; } /** * Returns the number of nodes in the list. This is an O(1) implementation as the * retrieval occurs in constant time * @return number of nodes */ public int size() { return size; } @Override public String toString() { if (front == null) { return "[]"; } StringBuilder result = new StringBuilder("[" + front.data); ListNode curr = front.next; while (curr != null) { result.append(",").append(curr.data); curr = curr.next; } result.append("]"); return result.toString(); } /** * Adds a node at the given index * @param index integer in range 0 to size * @param value integer to add to the list * * */ public void add(int index, int value) { if (size == 0){ throw new IndexOutOfBoundsException("index out of bounds for size == 0"); } if (index > size || index curr = front; for (int i = 0; i (value, curr.next); size++; } } /** * Returns the data value for a node at a given index * @param index indicates position in list from 0 to size - 1 * @return data value for node */ public int get(int index) { if (size == 0){ throw new IndexOutOfBoundsException("index out of bounds for size == 0"); } if (index >= size || index curr = front; for (int i = 0; i On your own (or with a partner), complete: Change the data at a particular "virtual" index in the LinkedList to the value passed to this method. - Make sure that the index is valid for the size of the list - Traverse to the node in the LinkedList that you are looking for - Overwrite the data at that node to be the given new value Be careful not to lose the front of the list! Remove the node at the given "virtual" index, conceptually "shifting" over all other elements as would happen in an ArrayList. - Make sure that the index does not exceed the size of the list (this includes an empty list) - Handle index 0 by calling removeFront - Handle index = size 1 by calling removeEnd - Otherwise: - Traverse to the node in the LinkedList that comes before the place you need to insert the node - Point the previous node to the node after the node that you will remove Be careful not to lose the front of the list
Step by Step Solution
There are 3 Steps involved in it
Step: 1
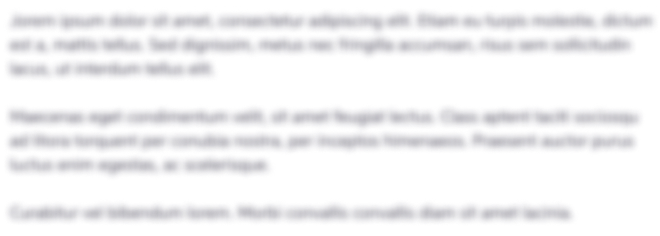
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started