Question
Please create a Java program that will simulate a clean digital square wave signal and then add Gaussian noise to the signal as a percentage
Please create a Java program that will simulate a clean digital square wave signal and then add Gaussian noise to the signal as a percentage of the maximum signal strength. The square wave signal has two values of 0s and 1s . Assume the duration of zeros or ones is equal to one millisecond (for simplicity you may assume every 10 zeroes or 10 ones generated by program is one millisecond). The total simulation time is 10 milliseconds. The program should prompt the user to enter the noise percentage and then it adds the noise to the signal. The Gaussian noise could be generated using the function NextGuassian() in java. The NextGuassain() generates random normal numbers between 0 and 1 (note that you need to have the library import java.util.Random; in the program). Since the noise could have both positive and negative values, this function needs to be scaled to a new range of positive and negative noise percentage (e.g.; -10% to +10%). Thus, the following equation should be used in the program: Noise_amount = Noise_percent*( -1+2*NextGuassain() ) The noisy data in the program is obtained as follows: Noisy_data = Clean_data + Noise_amount Next, the program simulates the function of a hub/switch to filter out the noise from the signal. This could be easily accomplished by an "If" statement. For example, if the value of the noise data is greater than one or greater than 0.51 then the filtered data is set to 1; otherwise, the value is set to 0. The program should output the clean data, noisy data, and the filtered data into a text file in form of 3 columns for a given noise percentage. You need to graph these data using excel and submit your computer program. The computer program must be well documented. The program for this project should include the following 5 distinct sections as shown below. Note that the program gets its input data from the user interactively. Program Header: (Programmer's Name, Class, Date, Program's Name, Program's Description)this section is mainly documentation Declaration of Variables: (e.g.; integers, floats, strings, classes, objects, etc.) Program Inputs: List of input data (e.g.; noise_percentage, output file name) Processes: Clean data using a counting loop, Noisy data is the summation of clean data and Gaussian noise, Filtered data is generated from the noisy data using an If statement Program Outputs: Noise percentage, clean data, noisy data, and filtered data in a data file in a formatted manner (see the attachment) Run the program for 5 cases of noise levels, namely; 10%, 20% 30%, 40%, and 50% A running example of the program is shown below: Program inputs that are entered interactively: Enter percentage of noise: 10 Enter name of output file: DigitalData.txt Do you want to continue (Yes/No): Yes Program Outputs: The prorgam outputs are generated in the file DigitalData.txt The assignment should have a brief project description, Algorithm for the program, a well documented project source code in Java, Printed output files, For each noise level, graphs of clean and noisy data in one chart and the graph of filtered data in a seperate chart, and analysis of the obtained results.. Sample Output Data file Noise Percentage: 10% Clean data Noisy Data Filtered Data 0 0.025170022 0 0 -0.092634112 0 0 0.093261424 0 0 0.026753381 0 0 -0.011235332 0 0 0.037359408 0 0 -0.078366917 0 0 1.78342E-05 0 0 0.052983874 0 0 0.082890804 0 1 0.956852102 1 1 1.092310582 1 1 1.010471249 1 1 0.912517327 1 1 0.945451824 1 1 1.096408286 1 1 1.052747855 1 1 1.066120388 1 1 0.917508309 1 1 0.924961827 1 0 -0.000139453 0 0 -0.077387348 0 0 -0.029915433 0 0 -0.087696933 0 0 0.024252159 0 0 0.062054077 0 0 0.063790965 0 0 0.038026812 0 0 0.005230972 0 0 0.018041356 0 1 0.912261794 1 1 0.919291923 1 1 0.951687696 1 1 1.02681557 1 1 0.97000462 1 1 1.070994369 1 1 0.959570194 1 1 1.033450441 1 1 1.035072427 1 1 1.092906998 1 0 0.025040218 0 0 -0.011892461 0 0 0.045966135 0 0 -0.015745845 0 0 0.096973094 0 0 0.039116721 0 0 0.054758146 0 0 -0.007886116 0 0 0.006005197 0 0 -0.034683362 0 Sample of a Well Documented Program import java.io.*; import java.util.Scanner; /**************************************************************************** * This program is developed to add two numbers. * * The AddNum program implements an application that * * simply adds two integer numbers input interactively by the user and * * print the output on the screen. * * * * Author: John Doe * * Version: 1.0 * * Date: 03-12-2018 * * Operating system: Windows 7 * ****************************************************************************/ public class AddNum { /*********************************************************************** * This method is used to add two integers. This is * * a the simplest form of a class method, just to * * show the usage of various javadoc Tags. * * @param numA This is the first parameter to addNum method * * @param numB This is the second parameter to addNum method * * @return int This returns sum of numA and numB. * ***********************************************************************/ public int addNum(int numA, int numB) { return numA + numB; } /****************************************************************************** * This is the main method which makes use of addNum method. * * @param args Unused. * * @return Nothing. * * @exception IOException On input error. * * @see IOException * ******************************************************************************/ public static void main(String args[]) throws IOException { /****************************************************************************** * D E C L A R A T I O N O F V A R I A B L E S * * Num1: An integer number interactively input by the user to the program * * Num2: An integer number interactively input by the user to the program * * Sum: Is the output of the program and holds the summation Num1 and Num2 * ******************************************************************************/ int Num1, Num2, sum; Scanner input = new Scanner(System.in); /****************************************************************************** * I N P U T S E C T I O N * * The program inputs two numbers from the user interactively by prompting * * the user to enter integer values for variables Num1 and Num2 * ******************************************************************************/ System.out.print("Please enter the first integer number: "); Num1 = input.nextInt(); System.out.print("Please enter the second integer number: "); Num2 = input.nextInt(); /****************************************************************************** * P R O C E S S I N G S E C T I O N * * An object called "obj" is created from the user defined class AddNum * * The obj is fed with the Num1 and Num2 and the result is stored in Sum * *****************************************************************************/ AddNum obj = new AddNum(); Sum = obj.addNum(Num1, Num2); /****************************************************************************** * O U T P U T S E C T I O N * * The program outputs variable Sum on the computer screen * ******************************************************************************/ System.out.println("Sum of Num1 and Num2 is :" + Sum); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
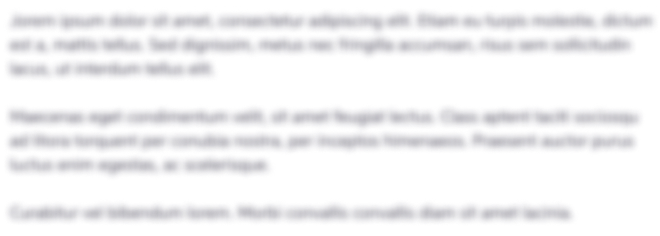
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started