Question
please create a test plan for this program: Thank you ArrayUnbndQueue.java //--------------------------------------------------------------------------- // ArrayUnbndQueue.java by Dale/Joyce/Weems Chapter 5 // // Implements UnboundedQueueInterface with an array
please create a test plan for this program:
Thank you
ArrayUnbndQueue.java
//--------------------------------------------------------------------------- // ArrayUnbndQueue.java by Dale/Joyce/Weems Chapter 5 // // Implements UnboundedQueueInterface with an array to hold queue elements. // // Two constructors are provided; one that creates a queue of a default // original capacity and one that allows the calling program to specify the // original capacity. // // If an enqueue is attempted when there is no room available in the array, a // new array is created, with capacity incremented by the original capacity. //---------------------------------------------------------------------------
package ch05.queues;
public class ArrayUnbndQueue
public ArrayUnbndQueue() { queue = (T[]) new Object[DEFCAP]; rear = DEFCAP - 1; origCap = DEFCAP; }
public ArrayUnbndQueue(int origCap) { queue = (T[]) new Object[origCap]; rear = origCap - 1; this.origCap = origCap; }
private void enlarge() // Increments the capacity of the queue by an amount // equal to the original capacity. { // create the larger array T[] larger = (T[]) new Object[queue.length + origCap]; // copy the contents from the smaller array into the larger array int currSmaller = front; for (int currLarger = 0; currLarger < numElements; currLarger++) { larger[currLarger] = queue[currSmaller]; currSmaller = (currSmaller + 1) % queue.length; } // update instance variables queue = larger; front = 0; rear = numElements - 1; }
public void enqueue(T element) // Adds element to the rear of this queue. { if (numElements == queue.length) enlarge();
rear = (rear + 1) % queue.length; queue[rear] = element; numElements = numElements + 1; }
public T dequeue() // Throws QueueUnderflowException if this queue is empty; // otherwise, removes front element from this queue and returns it. { if (isEmpty()) throw new QueueUnderflowException("Dequeue attempted on empty queue."); else { T toReturn = queue[front]; queue[front] = null; front = (front + 1) % queue.length; numElements = numElements - 1; return toReturn; } }
public boolean isEmpty() // Returns true if this queue is empty; otherwise, returns false { return (numElements == 0); } }
BoundedQueueInterface.java
//---------------------------------------------------------------------------- // BoundedQueueInterface.java by Dale/Joyce/Weems Chapter 5 // // Interface for a class that implements a queue of T with a bound // on the size of the queue. A queue is a "first in, first out" structure. //----------------------------------------------------------------------------
package ch05.queues;
public interface BoundedQueueInterface
{ void enqueue(T element) throws QueueOverflowException; // Throws QueueOverflowException if this queue is full; // otherwise, adds element to the rear of this queue.
boolean isFull(); // Returns true if this queue is full; otherwise, returns false. }
GlassQueue.java
//--------------------------------------------------------------------------- // GlassQueue.java by Dale/Joyce/Weems Chapter 5 // // Extends ArrayUnbndQueue with operations to determine the size of the queue // and to access the front and rear queue elements without removing them. //---------------------------------------------------------------------------
package ch05.queues;
public class GlassQueue
public GlassQueue() { super(); }
public GlassQueue(int origCap) { super(origCap); }
public int size() // Returns the number of elements in this queue. { return numElements; } public T peekFront() // Returns the object at the front of this queue. // If the queue is empty, returns null. { return queue[front]; } public T peekRear() // Returns the object at the rear of this queue. // If the queue is empty, returns null. { return queue[rear]; } }
QueueInterface.java
//---------------------------------------------------------------------------- // QueueInterface.java by Dale/Joyce/Weems Chapter 5 // // Interface for a class that implements a queue of T. // A queue is a "first in, first out" structure. //----------------------------------------------------------------------------
package ch05.queues;
public interface QueueInterface
{ T dequeue() throws QueueUnderflowException; // Throws QueueUnderflowException if this queue is empty; // otherwise, removes front element from this queue and returns it.
boolean isEmpty(); // Returns true if this queue is empty; otherwise, returns false. }
QueueOverflowException.java
package ch05.queues;
public class QueueOverflowException extends RuntimeException { public QueueOverflowException() { super(); }
public QueueOverflowException(String message) { super(message); } }
QueueUnderFlowException.java
package ch05.queues;
public class QueueUnderflowException extends RuntimeException { public QueueUnderflowException() { super(); }
public QueueUnderflowException(String message) { super(message); } }
UnboundedQueueInterface.java
//---------------------------------------------------------------------------- // UnboundedQueueInterface.java by Dale/Joyce/Weems Chapter 5 // // Interface for a class that implements a queue of T with no bound // on the size of the queue. A queue is a "first in, first out" structure. //----------------------------------------------------------------------------
package ch05.queues;
public interface UnboundedQueueInterface
{ void enqueue(T element); // Adds element to the rear of this queue. }
Simulation.java
package main; //--------------------------------------------------------------------- // Simulation.java by Dale/Joyce/Weems Chapter 5 // // Models a sequence of customers being serviced // by a number of queues. //---------------------------------------------------------------------
import support.*; // Customer, CustomerGenerator import ch05.queues.*;
public class Simulation { final int MAXTIME = Integer.MAX_VALUE; CustomerGenerator custGen; // a customer generator //CustomerGenerator2 custGen; // fixed distribution of IATs and STs float avgWaitTime = 0.0f; // average wait time for most recent simulation int maxCustomersOnLine; int maxOnQ; public Simulation(int minIAT, int maxIAT, int minST, int maxST) { custGen = new CustomerGenerator(minIAT, maxIAT, minST, maxST); } public float getAvgWaitTime() { return avgWaitTime; }
public int getMaxCustomersOnLine() { return maxCustomersOnLine; }
public int getMaxOnQueue() { return maxOnQ; }
public void simulate(int numQueues, int numCustomers, boolean useShortestQueue) // Preconditions: numQueues > 0 // numCustomers > 0 // No time generated during simulation is > MAXTIME // // Simulates numCustomers customers entering and leaving the // a queuing system with numQueues queues { // the queues GlassQueue
Customer nextCust; // next customer from generator Customer cust; // holds customer for temporary use int customersOnLine;
int totWaitTime = 0; // total wait time int custInCount = 0; // count of customers started so far int custOutCount = 0; // count of customers finished so far int nextArrTime; // next arrival time int nextDepTime; // next departure time int nextQueue; // index of queue for next departure int assignedQueue; int shortest; // int shortestSize; // int quickest; // int shortestWait; // Customer rearCust; // customer at rear of shortest queue
int finishTime; // calculated finish time for customer being enqueued
// instantiate the queues for (int i = 0; i < numQueues; i++) queues[i] = new GlassQueue
if (nextArrTime < nextDepTime) // handle customer arriving { assignedQueue = 0; if (numQueues > 1) { // determine assigned queue shortest = 0; quickest = 0; if (queues[0].size() > 0) { shortestSize = queues[0].size(); shortestWait = queues[0].peekRear().getFinishTime(); for (int i = 1; i < numQueues; i++) { if (queues[i].size() > 0) { if (queues[i].size() < shortestSize) { shortest = i; shortestSize = queues[i].size(); } if (queues[i].peekRear().getFinishTime() < shortestWait) { quickest = i; shortestWait = queues[i].peekRear().getFinishTime(); } } else { shortest = i; quickest = i; break; } } } if (useShortestQueue) assignedQueue = shortest; else assignedQueue = quickest; }
// determine the finish time if (queues[assignedQueue].size() == 0) finishTime = nextCust.getArrivalTime() + nextCust.getServiceTime(); else { finishTime = queues[assignedQueue].peekRear().getFinishTime() + nextCust.getServiceTime(); } // set finish time and enqueue customer nextCust.setFinishTime(finishTime); queues[assignedQueue].enqueue(nextCust); if (queues[assignedQueue].size() > maxOnQ) maxOnQ = queues[assignedQueue].size();
custInCount = custInCount + 1; // if needed, get next customer to enqueue if (custInCount < numCustomers) nextCust = custGen.nextCustomer(); } else // handle customer leaving { cust = queues[nextQueue].dequeue(); totWaitTime = totWaitTime + cust.getWaitTime(); custOutCount = custOutCount + 1; } customersOnLine = 0; for (int i = 0; i < numQueues; i++) { customersOnLine += queues[i].size(); } if (customersOnLine > maxCustomersOnLine) maxCustomersOnLine = customersOnLine; //System.out.println(custInCount + " - " + custOutCount + " - " + customersOnLine);
} // end while avgWaitTime = totWaitTime/(float)numCustomers; } }
SimulationApp.java
package main; //--------------------------------------------------------------------- // SimulationApp.java by Dale/Joyce/Weems Chapter 5 // // Simulates customers waiting in queues. Customers always enter // the shortest queue. // // Input consists of customer information: // Minimum and maximum customer inter-arrival time. // Minimum and maximum customer service time. // Followed by a sequence of simulation instance information: // Number of queues and customers. // // Output includes, for each simulation instance: // The average waiting time for a customer. //----------------------------------------------------------------------
import java.util.Scanner;
public class SimulationApp { public static void main(String[] args) { Scanner conIn = new Scanner(System.in);
int minIAT; // minimum inter-arrival time int maxIAT; // maximum inter-arrival time int minST; // minimum service time int maxST; // maximum service time int numQueues; // number of queues int numCust; // number of customers boolean useShortestQueue;
String skip; // skip end of line after reading an integer String more = null; // used to stop or continue processing
// Get customer information System.out.print("Enter minimum inter-arrival time: "); minIAT = conIn.nextInt(); System.out.print("Enter maximum inter-arrival time: "); maxIAT = conIn.nextInt(); System.out.print("Enter minimum service time: "); minST = conIn.nextInt(); System.out.print("Enter maximum service time: "); maxST = conIn.nextInt();
// create object to perform simulation Simulation sim = new Simulation(minIAT, maxIAT, minST, maxST);
do { // Get next simulation instance to be processed. System.out.print("Enter number of queues: "); numQueues = conIn.nextInt(); System.out.print("Enter number of customers: "); numCust = conIn.nextInt(); skip = conIn.nextLine(); // skip end of line useShortestQueue = true; if (numQueues > 1) { System.out.print("Please hit just Enter to assign customers to shortest queue; type anything, then Enter for shortest finish time assignment."); skip = conIn.nextLine(); if (skip.length() > 0) useShortestQueue = false; } // run simulation and output average waiting time sim.simulate(numQueues, numCust, skip.length() == 0); if (numQueues > 1) { if (useShortestQueue) System.out.println(">>> New customers are assigned to shortest queue." ); else System.out.println(">>> New customers are assigned to queue with shortet finish time." ); } System.out.println("Average waiting time is " + sim.getAvgWaitTime()); System.out.println("Max customers on line: " + sim.getMaxCustomersOnLine()); System.out.println("Max on a single queue: " + sim.getMaxOnQueue());
// Determine if there is another simulation instance to process System.out.println(); System.out.print("Evaluate another simulation instance? (Y=Yes): "); more = conIn.nextLine(); System.out.println(); } while (more.equalsIgnoreCase("y"));
System.out.println("Program completed."); } }
Customer.java
//---------------------------------------------------------------------- // Customer.java by Dale/Joyce/Weems Chapter 5 // // Supports customer objects having arrival, service, and finish time // attributes. Responsible for computing and returning wait time. //----------------------------------------------------------------------
package support;
public class Customer { protected int arrivalTime; protected int serviceTime; protected int finishTime;
public Customer(int arrivalTime, int serviceTime) { this.arrivalTime = arrivalTime; this.serviceTime = serviceTime; }
public int getArrivalTime() { return arrivalTime; } public int getServiceTime() { return serviceTime; }
public void setFinishTime(int time) { finishTime = time; }
public int getFinishTime() { return finishTime; }
public int getWaitTime() { return (finishTime - arrivalTime - serviceTime); }
}
CustomerGenerator.java
//---------------------------------------------------------------------- // CustomerGenerator.java by Dale/Joyce/Weems Chapter 5 // // Generates a sequence of random Customer objects based on the // constructor arguments for min and max interarrival and service times. // Assumes a flat distribution of both interarrival and service times. // Assumes time starts at 0. //----------------------------------------------------------------------
package support;
import java.util.Random;
public class CustomerGenerator { protected int minIAT; // minimum inter-arrival time protected int maxIAT; // maximum inter-arrival time protected int minST; // minimum service time protected int maxST; // maximum service time protected int currTime = 0; // current time Random rand = new Random(); // to generate random numbers
public CustomerGenerator (int minIAT, int maxIAT, int minST, int maxST) // Preconditions: all arguments >= 0 // minIAT <= maxIAT // minST <= maxST { this.minIAT = minIAT; this.maxIAT = maxIAT; this.minST = minST; this.maxST = maxST; }
public void reset() { currTime = 0; }
public Customer nextCustomer() // Creates and returns the next random customer. { int IAT; // next inter-arrival time int ST; // next service time IAT = minIAT + rand.nextInt(maxIAT - minIAT + 1); ST = minST + rand.nextInt(maxST - minST + 1); currTime = currTime + IAT; // updates current time to the arrival // time of next customer Customer next = new Customer(currTime, ST); return next; } }
LLNode.java
//---------------------------------------------------------------------------- // LLNode.java by Dale/Joyce/Weems Chapter 3 // // Implements
package support;
public class LLNode
public T getInfo() // Returns info of this LLONode. { return info; } public void setLink(LLNode
public LLNode
Step by Step Solution
There are 3 Steps involved in it
Step: 1
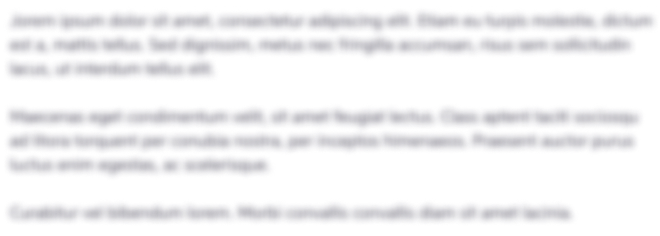
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started