Question
Please debug this code so I can run it with code:blocks and Linux terminal. If you don't mind, please write a makefile for this code
Please debug this code so I can run it with code:blocks and Linux terminal. If you don't mind, please write a makefile for this code as well. Please comment on what you did to fix it. Also, please take screenshots of the working code. Thank you
#include
using namespace std;
int main() {
bool keep_playing = true; bool win = false; int time; string solution; string proposed_solution; char c; char solved = '!'; char quit_game = '0';
cout << "Please enter a word or phrase to guess: "; getline(cin, solution); cout << endl; cout << "How many guesses will the player have?: "; cin >> time;
Puzzle my_puzzle(solution); Fuze my_fuse(time);
/* clear the screen */ cout << string(50, ' ');
cout << "========================"<< endl; cout << " BOOM!" << endl; cout << "========================"<< endl;
cout << "Enter a lower case letter to guess: " << endl; cout << "Enter ! to guess the whole word/phrase." << endl; cout << "Enter 0 to quit." << endl;
while (keep_playing == true) {
cout << my_fuse.to_string() << endl; cout << my_puzzle.to_string() << endl; cout << "Enter a letter that you would like to guess: "; cin.ignore(); cin >> c;
if (c == solved) { cout << "Oh you think you got it? " << endl; cout << "Well what is it?: "; cin.ignore(); getline(cin, proposed_solution); if (my_puzzle.solve(proposed_solution) == true) { cout << "Wow, you actually got it. You win!"<< endl; keep_playing = false; win = true;}
else { cout << "Not quite.. Try again." << endl;} }
else if (c == quit_game) { keep_playing = false; }
else { if (my_puzzle.guess(c) == true) { cout << "Nice job." << endl;} else { if (!my_fuse.burn()) { keep_playing = false;}
} } if (my_puzzle.to_string() == solution) { win = true; keep_playing = false;}
}
if(win) { cout << "****** YOU WIN! ******" << endl; cout << my_puzzle.get_solution() << " was the right answer!" << endl; } else { cout << "BBBBBBBOOOOOOOOOOOOOOOOOOOOOMMMM!!!!!!!!!!!!!!" << endl; cout << endl; cout << "The correct answer was: " << my_puzzle.get_solution() << endl; }
return 0; } ----------------------------------------------------------- Fuze.cpp ------------------------------- #include "fuse.h"
bool Fuse::burn() { if (_time > 0) { --_time; return true;}
else {return false;} } string Fuse::to_string() { string fuse_str; fuse_str +=",____________________, "; fuse_str +="| | "; fuse_str +=" "; for(int i=0; i < _time; ++i) { fuse_str += "~"; } fuse_str += "* "; fuse_str +="| | "; fuse_str +=",____________________, ";
return (fuse_str);} -------------------------------------------------------------- Fuze.h ------------------------------ #ifndef FUSE_H #define FUSE_H #include
class Fuse {
private: int _time;
public: /*Constructor for the fuse, initializing time */ Fuse(int time) : _time{time} { }
/*Decrements the fuse and returns time_left true/false*/ bool burn();
/* ASCII representation of the firecracker */ string to_string();
};
#endif ------------------------------------------------------------------ Puzzle.cpp -------------------------------------- #include "puzzle.h" #include
Puzzle::Puzzle(string solution) {
char answer; _solution = solution; while (1) { cout << "Is this correct?: " << _solution << " [y/n]: "; cin.ignore(); cin >> answer; if (answer == 'y') { break; }
else if (answer == 'n') { cout << "Try again." << endl;}
else {
cout << "Invalid Input. Try again." << endl;}
}
display = solution; display[solution.length()]; for(int i =0; i < solution.length(); ++i) { if (solution[i] != ' ') { display[i] = '_';} else { display[i] = ' ';} } }
bool Puzzle::guess(char c) {
int to_val = c; if ((to_val >= 97) && (to_val <= 122) ) { _guesses[to_val] = true;
for (int i=0; i < _solution.length(); ++i) { if (c == _solution[i]) { display[i] = c; } } return false;}
else { cout << "Invalid Input. Please insert a character from the alphabet." << endl; return true;}
}
bool Puzzle::solve(string proposed_solution) {
if (proposed_solution == _solution) { return true; }
else { cout << "Not quite.. Try again." << endl; return false;} }
string Puzzle::to_string() {
return display;}
string Puzzle::get_solution() {
return _solution;} ---------------------------------------------------------------------------- Puzzle.h --------------------------------------- #ifndef PUZZLE_H #define PUZZLE_H #include
class Puzzle {
private:
string _solution; bool _guesses[255] = {false}; string display;
public:
Puzzle(string solution);
bool guess(char c);
bool solve(string proposed_solution);
string to_string();
string get_solution();
}; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
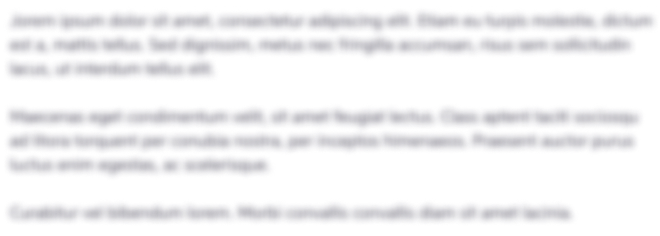
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started