Answered step by step
Verified Expert Solution
Question
1 Approved Answer
please do it using c++ and do as it is given in the pictures 5. Write a class called StudentList, which will be used to
please do it using c++ and do as it is given in the pictures
5. Write a class called StudentList, which will be used to store the list of various students, using a single array of pointers but with flexible size. Note that this array will act as a heterogeneous list, and it will need to be dynamically managed. Each item in the array should be a Base Class pointer, which should point to the appropriate type of object. Your list should only be big enough to store the students currently in it. Your Student List class needs to have these public functions available: a StudentList() -- default constructor. Sets up an empty list b-StudentList() -- destructor. Needs to clean up all dynamically managed data being managed inside a StudentList object, before it is deallocated c bool ImportFile(const char* filename) i This function should add all data from the given file (the parameter) into the internally managed list of students. The file format is specified below in this writeup. (Note that if there is already data in the list from a previous import, this call should add MORE data to the list). Records should be added to the end of the given list in the same order they appear in the input file. If the file does not exist or cannot be opened, return false for failure. Otherwise, after importing the file, return true for success. d bool CreateReportFile(const char* filename) i This function should create a grade report and write it to an output file (filename given by the parameter). Grade report file format is described below in this writeup. If the output file cannot be opened, return false for failure. Otherwise, after writing the report file, return true for success. e void showList() const i This function should print to the screen the current list of students, one student per line. The only information needed in this printout is last name, first name, and course name. Format this output so that it lines up in columns Note that while this class will need to do dynamic memory management, you do not need to create a copy constructor or assignment operator overload (for deep copy) for this class. (Ideally we would, but in this case, we will not be using a Student List object in a context where copies of such a list will be made) Given below is the starting declarations for StudentList class with the appropriate prototypes class StudentList { public: StudentList(); // starts out empty -Student List(); // cleanup (destructor) bool ImportFile(const char* filename); bool CreateReportFile(const char* filename); void ShowList() const; // print basic list data private: ); 6. Write a program that creates a single StudentList object and then implements a menu interface to allow interaction with the object. Your main program should implement the following menu loop (any single letter options should work on both lower and upper case inputs): *** Student List menu *** Import students from a file Show student list (brief) Export a grade report to file Show this Menu Quit Program al 7. The import and export options should start by asking the user for input of a filename you may assume it will be 30 chars or less, no spaces. If the import/export fails due to bad file, print a message in the main() indicating that the job was not successfully completed). The "Show student list" option should print the brief student list to screen. Make sure that no data member of class changes in this function. The "Show this Menu" option should re-display the menu. Quit should end the menu program. 8. File formats Input File -- The first line of the input file will contain the number of students listed in the file. This will tell you how many student records are being imported from this file. After the first lines, every set of two lines constitutes a student entry. The first line of a student entry is the name, in the format lastName, firstName. Note that a name could india spaces -- the comma will delineate last name from first name. The second lite 60/69 contain the subject ("English", "History", or "Math"), followed by a list of grau integers), all separated by spaces. There will be no extra spaces at the ends of lines in the file. The order of the grades for each class type is as follows: English -- Attendance, Project, Midterm, Final Exam History -- Term Paper, Midterm, Final Exam Math -- Quiz 1. Quiz 2, Quiz 3, Test 1, Test 2, Final Exam 1. Output File -- The output file that you print should list each student's name (firstName lastName- no extra punctuation between), Final Exam grade, final average (printed to 2 decimal places), and letter grade (based on 10 point scale, i.e. 90100 A, 80-89 B, etc). Output should be separated by subject, with an appropriate heading before each section, and each student's information listed on a separate line, in an organized fashion. (See example below). The order of the students within any given category should be the same as they appear in the student list. Data must line up appropriately in columns when multiple lines are printed in the file. At the bottom of the output file, print a grade distribution of ALL students -- how many As, Bs, Cs, etc. 8. General Requirements No global variables, other than constants! All member data of your classes must be private or protected You may use any of the standard 1/0 libraries that have been discussed in class (iostream, iomanip, fstream, as well as C libraries, like cstring, cctype, etc). You may also use the string class library You may not use any of the other STL (Standard Template Libraries) besides string . 5. Write a class called StudentList, which will be used to store the list of various students, using a single array of pointers but with flexible size. Note that this array will act as a heterogeneous list, and it will need to be dynamically managed. Each item in the array should be a Base Class pointer, which should point to the appropriate type of object. Your list should only be big enough to store the students currently in it. Your Student List class needs to have these public functions available: a StudentList() -- default constructor. Sets up an empty list b-StudentList() -- destructor. Needs to clean up all dynamically managed data being managed inside a StudentList object, before it is deallocated c bool ImportFile(const char* filename) i This function should add all data from the given file (the parameter) into the internally managed list of students. The file format is specified below in this writeup. (Note that if there is already data in the list from a previous import, this call should add MORE data to the list). Records should be added to the end of the given list in the same order they appear in the input file. If the file does not exist or cannot be opened, return false for failure. Otherwise, after importing the file, return true for success. d bool CreateReportFile(const char* filename) i This function should create a grade report and write it to an output file (filename given by the parameter). Grade report file format is described below in this writeup. If the output file cannot be opened, return false for failure. Otherwise, after writing the report file, return true for success. e void showList() const i This function should print to the screen the current list of students, one student per line. The only information needed in this printout is last name, first name, and course name. Format this output so that it lines up in columns Note that while this class will need to do dynamic memory management, you do not need to create a copy constructor or assignment operator overload (for deep copy) for this class. (Ideally we would, but in this case, we will not be using a Student List object in a context where copies of such a list will be made) Given below is the starting declarations for StudentList class with the appropriate prototypes class StudentList { public: StudentList(); // starts out empty -Student List(); // cleanup (destructor) bool ImportFile(const char* filename); bool CreateReportFile(const char* filename); void ShowList() const; // print basic list data private: ); 6. Write a program that creates a single StudentList object and then implements a menu interface to allow interaction with the object. Your main program should implement the following menu loop (any single letter options should work on both lower and upper case inputs): *** Student List menu *** Import students from a file Show student list (brief) Export a grade report to file Show this Menu Quit Program al 7. The import and export options should start by asking the user for input of a filename you may assume it will be 30 chars or less, no spaces. If the import/export fails due to bad file, print a message in the main() indicating that the job was not successfully completed). The "Show student list" option should print the brief student list to screen. Make sure that no data member of class changes in this function. The "Show this Menu" option should re-display the menu. Quit should end the menu program. 8. File formats Input File -- The first line of the input file will contain the number of students listed in the file. This will tell you how many student records are being imported from this file. After the first lines, every set of two lines constitutes a student entry. The first line of a student entry is the name, in the format lastName, firstName. Note that a name could india spaces -- the comma will delineate last name from first name. The second lite 60/69 contain the subject ("English", "History", or "Math"), followed by a list of grau integers), all separated by spaces. There will be no extra spaces at the ends of lines in the file. The order of the grades for each class type is as follows: English -- Attendance, Project, Midterm, Final Exam History -- Term Paper, Midterm, Final Exam Math -- Quiz 1. Quiz 2, Quiz 3, Test 1, Test 2, Final Exam 1. Output File -- The output file that you print should list each student's name (firstName lastName- no extra punctuation between), Final Exam grade, final average (printed to 2 decimal places), and letter grade (based on 10 point scale, i.e. 90100 A, 80-89 B, etc). Output should be separated by subject, with an appropriate heading before each section, and each student's information listed on a separate line, in an organized fashion. (See example below). The order of the students within any given category should be the same as they appear in the student list. Data must line up appropriately in columns when multiple lines are printed in the file. At the bottom of the output file, print a grade distribution of ALL students -- how many As, Bs, Cs, etc. 8. General Requirements No global variables, other than constants! All member data of your classes must be private or protected You may use any of the standard 1/0 libraries that have been discussed in class (iostream, iomanip, fstream, as well as C libraries, like cstring, cctype, etc). You may also use the string class library You may not use any of the other STL (Standard Template Libraries) besides stringStep by Step Solution
There are 3 Steps involved in it
Step: 1
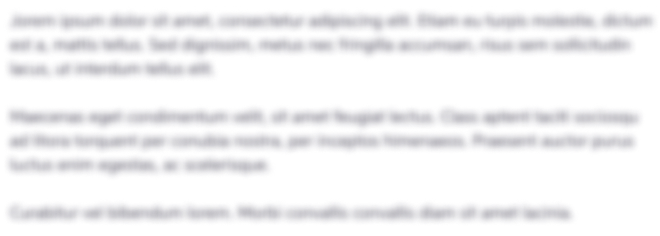
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started