Question
Please do not copy an answer from another chegg expert. Modify the List class of L ist.java to include method search that recursively searches a
Please do not copy an answer from another chegg expert.
Modify the List
The method should return a reference to the value if its found; otherwise, it should return null. Use your method in a test program that creates a list of integers. The program should prompt the user for a value to locate in the list.
==========List.java================
// ListNode and List class declarations. package com.deitel.datastructures;
import java.util.NoSuchElementException;
// class to represent one node in a list class ListNode
// constructor creates a ListNode that refers to object ListNode(E object) {this(object, null);}
// constructor creates ListNode that refers to the specified // object and to the next ListNode ListNode(E object, ListNode
// return reference to data in node E getData() {return data;}
// return reference to next node in list ListNode
// class List definition public class List
// constructor creates empty List with "list" as the name public List() {this("list");}
// constructor creates an empty List with a name public List(String listName) { name = listName; firstNode = lastNode = null; }
// insert item at front of List public void insertAtFront(E insertItem) { if (isEmpty()) { // firstNode and lastNode refer to same object firstNode = lastNode = new ListNode
// insert item at end of List public void insertAtBack(E insertItem) { if (isEmpty()) { // firstNode and lastNode refer to same object firstNode = lastNode = new ListNode
// remove first node from List public E removeFromFront() throws NoSuchElementException { if (isEmpty()) { // throw exception if List is empty throw new NoSuchElementException(name + " is empty"); }
E removedItem = firstNode.data; // retrieve data being removed
// update references firstNode and lastNode if (firstNode == lastNode) { firstNode = lastNode = null; } else { firstNode = firstNode.nextNode; }
return removedItem; // return removed node data }
// remove last node from List public E removeFromBack() throws NoSuchElementException { if (isEmpty()) { // throw exception if List is empty throw new NoSuchElementException(name + " is empty"); }
E removedItem = lastNode.data; // retrieve data being removed
// update references firstNode and lastNode if (firstNode == lastNode) { firstNode = lastNode = null; } else { // locate new last node ListNode
// loop while current node does not refer to lastNode while (current.nextNode != lastNode) { current = current.nextNode; }
lastNode = current; // current is new lastNode current.nextNode = null; }
return removedItem; // return removed node data }
// determine whether list is empty; returns true if so public boolean isEmpty() {return firstNode == null;}
// output list contents public void print() { if (isEmpty()) { System.out.printf("Empty %s%n", name); return; }
System.out.printf("The %s is: ", name); ListNode
// while not at end of list, output current node's data while (current != null) { System.out.printf("%s ", current.data); current = current.nextNode; }
System.out.println(); } }
=========ListTest.java to test the code==========
// Exercise 21.21 Solution: ListTest.java // Program recursively searches a list of numbers. import java.util.Random;
public class ListTest { public static void main(String[] args) { List
// create objects to store in the List for (int i = 0; i <25; i++) { number = randomNumber.nextInt(101); list.insertAtFront(number); }
list.print(); Integer searchResult = list.search(34);
// display result of searching 34 if (searchResult != null) { System.out.println("Value found: 34"); } else { System.out.println("Value not found: 34"); } searchResult = list.search(50);
// display result of searching 50 if (searchResult != null) { System.out.println("Value found: 50"); } else { System.out.println("Value not found: 50"); } searchResult = list.search(72);
// display result of searching 72 if (searchResult != null) { System.out.println("Value found: 72"); } else { System.out.println("Value not found: 72"); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
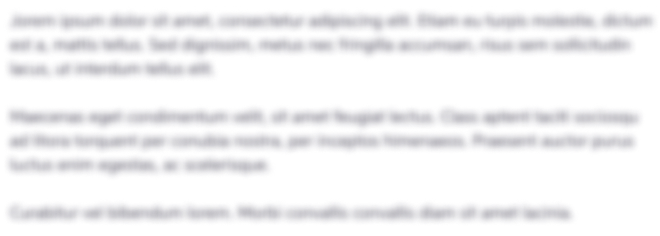
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started