Question
PLEASE DONT ACTUALLY USE GUI. WE ARE STIMULATING IT. In this assignment, we will implement a simple user interface (the view model) for the server
PLEASE DONT ACTUALLY USE GUI. WE ARE STIMULATING IT.
In this assignment, we will implement a simple user interface (the view model) for the server that we have developed in the first assignment. The user interface simulates a graphical user interface without the need to construct its graphics assets. Below is a description of the view model:
a. The view model is composed of a menu and a dashboard
b. The menu is composed of a list of menu-items. Each menu-item has a key, an order of appearance, a path (e.g. /services, /employees, /offices ), an icon and optionally a list of sub items.
c. A dashboard is a set of widgets
d. Each widget has a title and holds statistics about a given entity (e.g. employees, offices, etc.). Example of statistics are: (e.g. total number of items of the same entity, a breakdown by a certain field in the entity). For example: the employees widget has the total number of employees, a summary of number of secretaries, number of lawyers, etc. The offices widget has a summary of number of offices per floor.
e. The client can edit the title of a widget.
f. The client can click on a menu-item or sub item.
The implementation consists of two main parts: (1) client side; and (2) server side.
Below is a description of these parts and their interactions:
The client starts by requesting the menu and the dashboard objects from the server. Moreover, the client simulates the user clicking on some menu-items or editing widgets titles. Your design must be flexible so that any integration of a new feature is easily incorporated.
The client must implement two methods: clickMenuItem and editWidgetTitle.
clickMenuItem takes as parameter the menu-item (or sub item) object, and returns the result of a GET to the path assigned to the menu item. E.g. clicking on the /services menu-item is equivalent to requesting GET /services
editWidgetTitle takes as parameters the widget object, and the string to put in place of the old title value.
We finally want to store client interactions as events and give them different priorities. The flushEvents() method sorts the events based on their priorities and runs their assigned actions in the same order.
Next is a snapshot of the required client main code:
public static void main(String[] args) {
Server s = new Server();
Client cl = new Client(s);
Menu menu = cl.getMenu();
Dashboard dashboard = cl.getDashboard();
// add an event with priority 2
addEvent(new Event(2, client -> client.clickMenuItem(menu.getMenuItems().get(0)))); // add an event with priority 1
addEvent(new Event(1, client -> client.editWidgetTitle(dashboard.getWidgets().get(0), "Bob")));
// empty event!
addEvent(new Event(4, client -> {}));
flushEvents();
}
Output:
Event 1: Client edited the title of widget employees with text: bob
Event 2: Client clicked on services menu item
GET /services
< multiplication
< addition
PLEASE DONT ACTUALLY USE GUI. WE ARE STIMULATING IT.
I HAVE ALREADY IMPLEMENTED THE CLASSES REQUIRED TO SOLVE THE FOLLOWING QUESTION SO PLEASE REFER TO THE CLASSES BELOW AND THANK YOU:
/* * This solution is licensed to all the AUB CMPS 212 Course students of year 2017-2018 * Please use the solution wisely. */ package aub.cmps212.assignment1;
/** * * @author tsamman */ public class EntityEmployee { private int ID = 0; private String Name = ""; private String Status = ""; private String Profession = ""; private String Salary = ""; public EntityEmployee () { ID = 0; this.Name = ""; this.Status = ""; this.Profession = ""; this.Salary = ""; } public EntityEmployee (int inputID, String inputName, String inputStatus, String inputProfession, String inputSalary) { this.ID = inputID; this.Name = inputName; this.Status = inputStatus; this.Profession = inputProfession; this.Salary = inputSalary; }
public int getID() { return ID; } public String getName() { return Name; }
public String getStatus() { return Status; }
public String getProfession() { return Profession; }
public String getSalary() { return Salary; }
public void setID(int ID) { this.ID = ID; } public void setName(String Name) { this.Name = Name; }
public void setStatus(String Status) { this.Status = Status; }
public void setProfession(String Profession) { this.Profession = Profession; }
public void setSalary(String Salary) { this.Salary = Salary; } @Override public String toString () { String output = "Name: " + Name + " " + "Status: " + Status + " " + "Profession: " + Profession + " " + "Salary: " + Salary + " "; return output; } }
/* * This solution is licensed to all the AUB CMPS 212 Course students of year 2017-2018 * Please use the solution wisely. */ package aub.cmps212.assignment1;
/** * * @author tsamman */ public class EntityOffice { private int OfficeID = 0; private String Location = ""; private String Surface = ""; public EntityOffice () { this.OfficeID = 0; this.Location = ""; this.Surface = ""; } public EntityOffice (int inputOfficeID, String inputLocation, String inputSurface) { this.OfficeID = inputOfficeID; this.Location = inputLocation; this.Surface = inputSurface; }
public int getOfficeID() { return OfficeID; }
public String getLocation() { return Location; }
public String getSurface() { return Surface; }
public void setOfficeID(int OfficeID) { this.OfficeID = OfficeID; }
public void setLocation(String Location) { this.Location = Location; }
public void setSurface(String Surface) { this.Surface = Surface; } @Override public String toString () { String output = "Office ID: " + OfficeID + " " + "Location: " + Location + " " + "Surface: " + Surface + " "; return output; } }
/* * This solution is licensed to all the AUB CMPS 212 Course students of year 2017-2018 * Please use the solution wisely. */ package aub.cmps212.assignment1;
import java.io.FileNotFoundException; import java.util.ArrayList;
/** * * @author tsamman */
// This Class represents the parent class for all the resources // It is an abstract class that has the function definitions public abstract class Resource { private String ResourceName = ""; public Resource () { this.ResourceName = ""; } public Resource (String name) { this.ResourceName = name; } public void setResourceName (String name) { this.ResourceName = name; } public String getResourceName () { return this.ResourceName; } // The String... represents a variable input parameter, meaning if we don't know the number of parameters we want to use, we use this way // then params can be used as an input array public abstract String GET () throws FileNotFoundException; public abstract String GET (String... params); public abstract String POST (); public abstract String POST (String... params); public abstract String PUT (); public abstract String PUT (String... params); public abstract String PATCH (); public abstract String PATCH (String... params); public abstract String DELETE () throws FileNotFoundException; public abstract String DELETE (String... params); }
/* * This solution is licensed to all the AUB CMPS 212 Course students of year 2017-2018 * Please use the solution wisely. */ package aub.cmps212.assignment1;
import java.util.ArrayList;
/** * * @author tsamman */ public class ResourceEntities extends Resource { int employeeCount = 1; int officeCount = 1; ArrayList
return output; } } else if (params[0].equalsIgnoreCase("Offices") == true) { if (index != -1) { output = "Office with ID: " + offices.get(index).getOfficeID() + " updated.. "; offices.get(index).setLocation(params[2]); offices.get(index).setSurface(params[3]);
return output; } } return "Entity update at requested ID failed, please check.. "; } @Override public String PATCH () { return ""; } public String PATCH (String... params) { String output = ""; int index = Integer.parseInt(params[1]) - 1; if (params[0].equalsIgnoreCase("Employees") == true) { if (index != -1) { output = "Employee with ID: " + employees.get(index).getID() + " patched.. "; if (params[2].equalsIgnoreCase("Name") == true) { employees.get(index).setName(params[3]); } else if (params[2].equalsIgnoreCase("Status") == true) { employees.get(index).setStatus(params[3]); } else if (params[2].equalsIgnoreCase("Profession") == true) { employees.get(index).setProfession(params[3]); } else if (params[2].equalsIgnoreCase("Salary") == true) { employees.get(index).setSalary(params[3]); }
return output; } } else if (params[0].equalsIgnoreCase("Offices") == true) { if (index != -1) { output = "Office with ID: " + offices.get(index).getOfficeID() + " patched.. "; if (params[2].equalsIgnoreCase("Location") == true) { offices.get(index).setLocation(params[3]); } else if (params[2].equalsIgnoreCase("Surface") == true) { offices.get(index).setSurface(params[3]); }
return output; } } return "Entity update at requested ID failed, please check.. "; } @Override public String DELETE () { return ""; } @Override public String DELETE (String... params) { String output = ""; int index = Integer.parseInt(params[1]) - 1; if (params[0].equalsIgnoreCase("Employees") == true) { if (index != -1) { output = "Employee with ID: " + employees.get(index).getID() + " deleted.. "; employees.remove(index);
return output; } } else if (params[0].equalsIgnoreCase("Offices") == true) { if (index != -1) { output = "Office with ID: " + offices.get(index).getOfficeID() + " deleted.. "; offices.remove(index);
return output; } } return "Entity deletion at requested ID failed, please check.. "; } }
/* * This solution is licensed to all the AUB CMPS 212 Course students of year 2017-2018 * Please use the solution wisely. */ package aub.cmps212.assignment1;
import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.io.PrintWriter; import java.util.Scanner;
/** * * @author tsamman */ public class ResourceFile extends Resource { File currentFile = null; public ResourceFile () { super(); currentFile = null; } public ResourceFile (String fileURI) { super(fileURI); currentFile = new File(this.getResourceName()); } @Override public String GET () throws FileNotFoundException { String output = ""; Scanner readFile = new Scanner(currentFile); while (readFile.hasNextLine() == true) { output += readFile.nextLine() + " "; } return output; } @Override public String GET (String... params) { return ""; } @Override public String POST () { return ""; } @Override public String POST (String... params) { String fileName = params[0]; String fileContent = params[1]; try { PrintWriter writer = new PrintWriter(fileName, "UTF-8"); writer.println(fileContent); writer.close(); } catch (IOException e) { return "File Creation Failed.. "; } return "File Creation Successful.. "; } @Override public String PUT () { return "Please note, Files do not implement the PUT protocol.. "; } @Override public String PUT (String... params) { return ""; } @Override public String PATCH () { return "Please note, Files do not implement the PUT protocol.. "; } public String PATCH (String... params) { return ""; } @Override public String DELETE () throws FileNotFoundException { if (this.currentFile.delete() == true) return "File Delete Successful.. "; return "File Delete Failed.. "; } @Override public String DELETE (String... params) { return ""; } }
/* */ package aub.cmps212.assignment1;
import java.util.ArrayList;
/** * * @author tsamman */ public class ResourceServices extends Resource { ArrayList
/* * This solution is licensed to all the AUB CMPS 212 Course students of year 2017-2018 * Please use the solution wisely. */ package aub.cmps212.assignment1;
import java.io.FileNotFoundException; import java.util.Scanner; /** * * @author tsamman */ public class Server { // Scanner variable used for the System input (Keyboard) private Scanner scan = new Scanner(System.in); // The run method, used to run the server simulator public void run() throws FileNotFoundException { // Two resources, entities (employees, offices) and services (addition, substration, multiplication, division) ResourceEntities entities = new ResourceEntities(); ResourceServices services = new ResourceServices(); System.out.print("> "); // While there is still input while (scan.hasNextLine()) { // Read the next line String line = scan.nextLine(); if (line.length() == 0) { continue; } // Split the read line on the spaces String[] parts = line.split(" "); if (parts[0].equals("GET")) { // Split again on the slash "/" String[] getParts = parts[1].split("/"); if (getParts[1].equalsIgnoreCase("services") == true) { if (getParts.length == 2) { System.out.println(services.GET()); } else if (getParts.length == 3) { System.out.println(services.GET(getParts[2])); } } else if (getParts[1].equalsIgnoreCase("files") == true) { if (getParts.length == 3) { ResourceFile newFile = new ResourceFile(getParts[2]); System.out.println(newFile.GET()); } } else { if (getParts[1].equalsIgnoreCase("employees") == true) { if (getParts.length == 2) { System.out.println(entities.GET("employees")); } else if (getParts.length == 3) { System.out.println(entities.GET("employees", getParts[2])); } } else if (getParts[1].equalsIgnoreCase("offices") == true) { if (getParts.length == 2) { System.out.println(entities.GET("offices")); } else if (getParts.length == 3) { System.out.println(entities.GET("offices", getParts[2])); } } } } else if (parts[0].equals("POST")) { // Split again on the slash "/" String[] getParts = parts[1].split("/"); if (getParts[1].equalsIgnoreCase("services") == true) { if (getParts.length == 3) { String values = ""; for(int i = 2; i < parts.length; i++) { values += parts[i] + " "; }
String[] getDetails = values.split(" "); System.out.println(services.POST(getParts[2], getDetails[0], getDetails[1])); } } else if (getParts[1].equalsIgnoreCase("files") == true) { if (getParts.length == 2) { String values = ""; for(int i = 3; i < parts.length; i++) { values += parts[i] + " "; } ResourceFile newFile = new ResourceFile(parts[2]); System.out.println(newFile.POST(parts[2], values)); } } else { if (getParts[1].equalsIgnoreCase("employees") == true) { if (getParts.length == 2) { String values = ""; for(int i = 2; i < parts.length; i++) { values += parts[i] + " "; }
String[] getDetails = values.split(","); System.out.println(entities.POST("employees", getDetails[0], getDetails[1], getDetails[2], getDetails[3])); } } else if (getParts[1].equalsIgnoreCase("offices") == true) { if (getParts.length == 2) { String values = ""; for(int i = 2; i < parts.length; i++) { values += parts[i] + " "; }
String[] getDetails = values.split(","); System.out.println(entities.GET("offices", getDetails[0], getDetails[1], getDetails[2])); } } } } else if (parts[0].equals("PUT")) { if (parts.length > 2) { // Split again on the slash "/" String[] getParts = parts[1].split("/"); String values = ""; for(int i = 2; i < parts.length; i++) { values += parts[i] + " "; } String[] getDetails = values.split(","); if (getParts[1].equalsIgnoreCase("employees") == true) { System.out.println(entities.PUT("employees", getParts[2], getDetails[0], getDetails[1], getDetails[2], getDetails[3])); } else if (getParts[1].equalsIgnoreCase("offices") == true) { System.out.println(entities.PUT("offices", getParts[2], getDetails[0], getDetails[1], getDetails[2])); } } } else if (parts[0].equals("PATCH")) { if (parts.length > 2) { // Split again on the slash "/" String[] getParts = parts[1].split("/"); String values = ""; for(int i = 2; i < parts.length; i++) { values += parts[i] + " "; } String[] getDetails = values.split("="); if (getParts[1].equalsIgnoreCase("employees") == true) { System.out.println(entities.PATCH("employees", getParts[2], getDetails[0], getDetails[1])); } else if (getParts[1].equalsIgnoreCase("offices") == true) { System.out.println(entities.PATCH("offices", getParts[2], getDetails[0], getDetails[1])); } } } else if (parts[0].equals("DELETE")) { // Split again on the slash "/" String[] getParts = parts[1].split("/"); if (getParts[1].equalsIgnoreCase("services") == true) { if (getParts.length == 3) { System.out.println(services.DELETE(getParts[2])); } } else if (getParts[1].equalsIgnoreCase("files") == true) { if (getParts.length == 3) { ResourceFile newFile = new ResourceFile(getParts[2]); System.out.println(newFile.DELETE()); } } else { if (getParts[1].equalsIgnoreCase("employees") == true) { if (getParts.length == 3) { System.out.println(entities.DELETE("employees", getParts[2])); } } else if (getParts[1].equalsIgnoreCase("offices") == true) { if (getParts.length == 3) { System.out.println(entities.DELETE("offices", getParts[2])); } } } } // Added this Exit funtion just for ease of use else if (parts[0].equalsIgnoreCase("EXIT")) { break; } else { System.err.println("Method Not Supported"); } System.out.print("> "); } } /** * @param args the command line arguments */ public static void main(String[] args) throws FileNotFoundException { (new Server()).run(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
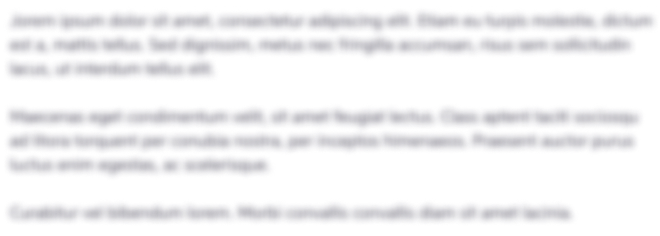
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started