Question
PLEASE DONT COMPLICATE THIS PROGRAM. USE FILE OPERATIONS C++ ONLY. * Programming Assignment Details You have been asked to write a program to help a
PLEASE DONT COMPLICATE THIS PROGRAM. USE FILE OPERATIONS C++ ONLY.
* Programming Assignment Details
You have been asked to write a program to help a friend choose among schools in Ohio. Your friend has input the data in a file with specific format to help you.
The input file contains the names, locations, current enrollments, and current tuitions of schools in Ohio, respectively. In particular, all information for one school is listed together. For example, in the input file, OH-in.dat, the first four lines indicate the name of the school (i.e., AntiochCollege), location of the school (i.e., YellowSprings), current enrollment of the school (i.e., 330), and current tuition of the school (i.e.,27800). Then, the same information for other schools is followed in the file. (Note that you must use OH-in.dat file as the input file for the program.) Also note that there are no spaces in the names of the schools, so you can safely use the >> operator to process input from the file using string type variables. The input file is in the lib directory under the class directory. Use cp command to copy the input file from the lib directory to your work directory.
- How to copy OH-in.dat to your work directory.
o Go to your work directory (i.e., Prog02).
o Type pwd command to get the full path to the work directory. This path will be used in the cp command later.
(Full path should be similar to /home/xx/yourID/cs202jl/Prog02.) o Copy the full path to the work directory.
o Then, go to the lib directory under the class directory.
o Type ls lt command to list the files in the lib directory.
(The input file should be listed if you are in the correct directory.) o Use cp command to copy the input file.
cp OH-in.dat fullPathToWorkDirecory
- The number of schools should be passed to the main( ) function via command line arguments.
o Use int main (int argc, char *argv[ ]) in your program.
o argc (argument count) is the number of strings pointed to by argv. It is 1 plus the number of arguments.
o argv (argument vector) holds the arguments passed to the main function.
o For example, when executing your program using ./runprog02 48 argc will be 2 and argv[0] will be ./runprog02 argv[1] will be 48
o The number of schools should be no more than 48.
(I may test your program with 48 and a smaller number.)
- The school information should be read and stored in an array of structure.
o struct name: schoolInfo
o struct members: schoolName, city, enrollment, tuition
Complete the following tasks in the order listed.
1. Using a function called, find_school( ), list the schools and the tuition of the schools in Cincinnati with tuition below 20000.
Before calling find_school( ) function, call another function called count_school( ) function to count how many school in Cincinnati with tuition below 20000. This function should accept the array of struct (for the school info), a string variable which was initialized with Cincinnati, and an int variable which holds the total number of schools. count_school( ) function should return an int variable which holds the count of the schools that meet the criteria. Use the int variable value to dynamically-declare an array of struct for the array of struct returned by find_school( ) function.
The find_school( ) function should accept the array of struct (for the school info), a string variable which was initialized with Cincinnati, an int variable which holds the total number of schools, and an int variable which holds the count of the schools that meet the criteria determined from the count_school( ) function. The find_school( ) function should return an array of struct for the school info that meets the criteria (name the struct as returnedSchools)
Using the returned array of struct, in the main function, display the number of schools that meet these criteria and the school names with their tuition.
2. Using a function called, avg_tuition( ), calculate and display the average tuition of schools with more than 10000 enrolled students.
The function should accept the array of struct (for the school info) and an int variable which holds the total number of schools.
All calculation and displaying the result should be done in this function for this task.
3. Using a function called, find_expensive_schools( ), give the name and tuition of the three most expensive schools. You can assume that the top three schools have different tuitions than all others (there are no "ties").
The function should accept the array of struct (for the school info) and an int variable which holds the total number of schools.
The function should return an array of struct for the school info that meets the criteria (name the struct asexpensiveSchools). (Note that you know the size of this array of struct.)
Using the returned array of struct, in the main function, display the school names with their tuition.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
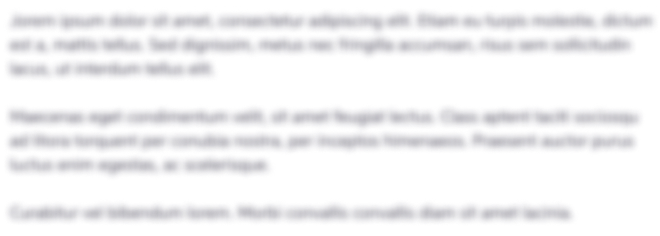
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started