Question
Please either post all 3 different codes for RetailItem Class, CashRegister Class and Main Function, or complete the two sets of code down below. Thanks
Please either post all 3 different codes for RetailItem Class, CashRegister Class and Main Function, or complete the two sets of code down below. Thanks
1. Create a class named RetailItem that holds data about an item in a retail store. The class should store the following data attributes: item description, units in inventory, and price.
Please define the 3 data attributes as private attributes and name them as description, inventory, and price.
Please define the initialization method __init__
Please define the corresponding accessor and mutator methods.
Please override the __str()__ method, which summarizes all data fields of the class.
Please save this class as a separate python module.
2. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class has a private data attribute named as items, which is a list object that is used to internally keep a list of RetailItem objects.
The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list.
A method named get_total that returns the sum of price of all the RetailItem objects stored in the CashRegister objects internal list.
A method named show_items that displays data about the RetailItem objects stored in the CashRegister objects internal list.
A method named clear that should clear the CashRegister objects internal list.
3. Demonstrate the CashRegister class in a hw4.py program that allows the user to select several items for purchase. When the user is ready to check out, the program should display a list of all the items he or she has selected for purchase, as well as the total price.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Here is the code for HW4Starter
# define and run the main method to demonstrate the CashRegister class
# first, you need to import the other two modules
# define Constants to hold the options of purchase items
PANTS = 1
SHIRT = 2
DRESS = 3
SOCKS = 4
SWEATER = 5
# main method
def main():
# Create sale items. For example:
# if the RetailItem class is in the retail module, enter the following
# pants = retail.RetailItem('Pants', 10, 19.99)
# shirt = retail.RetailItem('Shirt', 15, 12.50)
# dress = retail.RetailItem('Dress', 3, 79.00)
# socks = retail.RetailItem('Socks', 50, 1.00)
# sweater = retail.RetailItem('Sweater', 5, 49.99)
# complete the code to define the 5 sale items here using sample data in the example above
pants =
shirt =
dress =
socks =
sweater =
# Create dictionary of sale items.
sale_items = {PANTS:pants, SHIRT:shirt,DRESS:dress, SOCKS:socks,
SWEATER:sweater}
# Create a cash register object
# complete the code here
# Initialize loop test.
checkout = 'N'
# keep looping if user wants to purchase more items:
while checkout == 'N':
# call the get_user_choice() function to let user pick a sale item
# complete the code here:
# use the choice selected by the user to locate the sale item from the
# sale_items dictionary and assign it to a variable called item
# complete the code here:
item = sale_items[]
if item.get_inventory() == 0:
print('The item is out of stock.')
else:
# add the item to the cash register object created before the while loop
# complete the code here:
# update item by reducing the inventory number by 1 and assign it to variable new_item
# for example: if the RetailItem class is in the retail module, the code will be:
# new_item = retail.RetailItem(item.get_description(), \
# item.get_inventory()-1, \
# item.get_price())
# complete the code here:
new_item =
# assign this new_item as the new value to the selected key of the sale_items dictionary
# complete the code here
sale_items[] = new_item
# ask user to checkout or continue
checkout = input('Are you ready to check out (Y/N)? ')
# here is outside the while loop
# print out the total price of the cash register
# complete the code here:
print('Your purchase total is: ',)
# display items in the cashregister by calling the proper method
# complete the code here:
# now you can clear the items of the cashregister by calling the proper method
# complete the code here:
def get_user_choice():
print('Menu')
print('---------------------------------')
print('1. Pants')
print('2. Shirt')
print('3. Dress')
print('4. Socks')
print('5. Sweater')
print()
while True:
try:
choice = int(input('Enter the menu number of the item ' + \
'you would like to purchase: '))
while choice > SWEATER or choice < PANTS: # what you're testing here?
choice = int(input('Please enter a valid item number: '))
print()
return choice
except ValueError as err:
print(err)
print('Please enter a number from the menu!')
# Call the main function.
main()
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Here is the code for cashRegisterStarter
#define the CashRegister class here
#hint: first, you need to import the module that contains the RetailItem class
class CashRegister:
# Initialize an empty list called items to hold purchased items.
def __init__(self):
self.__items = []
# Method that clears the contents of the cash register.
def clear(self):
#complete this method
# Method that simulates adding an item to the cash register.
# It receives a RetailItem object as an argument.
def purchase_item(self, retail_item):
#hint: call the list class' append method to
#add the retail_item to the end of the list
#Complete the method
print('The item was added to the cash register.')
# Method returning the total cost of items in the list.
def get_total(self):
total = 0.0
#hint: use a for loop to loop through the list and
#add the each item's price to total
#Complete the method
return total
# Method that prints all the items in the list.
def show_items(self):
print('The items in the cash register are:')
#hint: use a for loop to loop through the list and
#print one item in each iteration
#Complete the method
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Step by Step Solution
There are 3 Steps involved in it
Step: 1
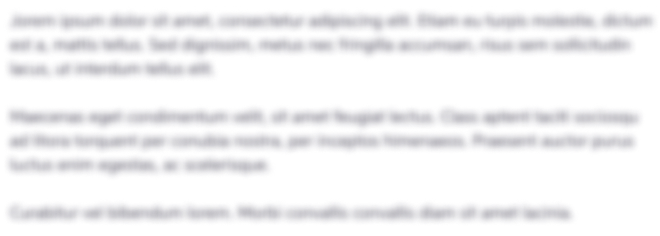
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started