Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please explain the following methods in details public class Task1 { public static void main (String[] args) { double [] nums1d = { 1 ,
Please explain the following methods in details
public class Task1 { public static void main(String[] args) { double[] nums1d = {1, 3}; double[][] nums1 = {{1, 2}, {3, 4, 5}, {6}}; double[][] nums2 = {{4, 6}, {12, 4, 5}, {6}}; String str = "abc"; String[] str2 = {"Hello", "World"}; String[][] str3 = {{"Hello", "World"}, {"Welcome", "to", "Java"}}; } /** * This method adds a number to each element of a 2d array * * @param nums1 {{1,2},{3,4,5},{6}} * @param value {5} * @return added value(5) to nums1 [[6.0, 7.0], [8.0, 9.0, 10.0], [11.0]] */ public static double[][] addValueToTwoDArray(double[][] nums1, double value) { for (double[] nums : nums1) { for (int i = 0; i < nums.length; i++) { nums[i] += value; } } return nums1; } /** * This method adds two 2d double arrays * * @param nums1 {6.0, 7.0}, {8.0, 9.0, 10.0},{11.0} * @param nums2 {{4,6},{12,4,5},{6}} * @return new array(nums1+nums2) [[10.0, 13.0], [20.0, 13.0, 15.0], [17.0]] */ public static double[][] addArrays(double[][] nums1, double[][] nums2) { double[][] newArray = new double[nums1.length][]; for (int i = 0; i < newArray.length; i++) { double[] array1row = nums1[i]; double[] array2row = nums2[i]; double[] newrow = new double[array1row.length]; for (int j = 0; j < newrow.length; j++) { newrow[j] = array1row[j] + array2row[j]; } newArray[i] = newrow; } return newArray; } /** * This method appends a 1d double array to a 2d double array as a new row * * @param nums1 {6.0, 7.0}, {8.0, 9.0, 10.0},{11.0} * @param nums1d {1,3} * @return appends 1d and 2d arrays [[6.0, 7.0], [8.0, 9.0, 10.0],[11.0] * [1.0, 3.0]] */ public static double[][] app1DArray(double[][] nums1, double[] nums1d) { double[][] result = Arrays.copyOf(nums1, nums1.length + 1); result[nums1.length] = nums1d; return result; } /** * This method appends a 2d double array under another 2d double array * * @param nums1 [[6.0, 7.0], [8.0, 9.0, 10.0], [11.0]] * @param nums2 {{4,6},{12,4,5},{6}} * @return new array [[6.0, 7.0], [8.0, 9.0, 10.0], [11.0], [4.0, 6.0] * [12.0, 4.0, 5.0], [6.0]] */ public static double[][] app2DArray(double[][] nums1, double[][] nums2) { double[][] app = new double[nums1.length + nums2.length][]; System.arraycopy(nums1, 0, app, 0, nums1.length); System.arraycopy(nums2, 0, app, nums1.length, nums2.length); return app; } /** * This method appends a 2d double array at the right of another 2d array * * @param nums1 [[6.0, 7.0], [8.0, 9.0, 10.0], [11.0]] * @param nums2 {{4,6},{12,4,5},{6}} * @return new array [[6.0, 7.0, 4.0, 6.0], [8.0, 9.0, 10.0, 12.0, 4.0, 5.0] * [11.0, 6.0]] */ public static double[][] appendRight(double[][] nums1, double[][] nums2) { // defining new array by increasing length by size of two arrays double[][] newArray = new double[nums1.length][]; // adding first array to the new array for (int i = 0; i < newArray.length; i++) { double[] array1row = nums1[i]; double[] array2row = nums2[i]; double[] newRow = new double[array1row.length + array2row.length]; for (int j = 0; j < array1row.length; j++) { newRow[j] = array1row[j]; } for (int j = 0; j < array2row.length; j++) { newRow[array1row.length + j] = array2row[j]; } newArray[i] = newRow; } return newArray; } /** * This method removes a row from a 2d array * * @param nums1 [[6.0, 7.0], [8.0, 9.0, 10.0], [11.0]] * @param index {0} * @return newArray [8.0, 9.0, 10.0], [11.0]] */ public static double[][] removeRow(double[][] nums1, int index) { double[][] newArray; if (index < 0 || index >= nums1.length) { newArray = new double[nums1.length][]; } else { newArray = new double[nums1.length - 1][]; } int newIndex = 0; for (int i = 0; i < nums1.length; i++) { // if if the idx is invalid, returns the orignal array if (i != index) { newArray[newIndex] = nums1[i]; newIndex++; } } return newArray; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
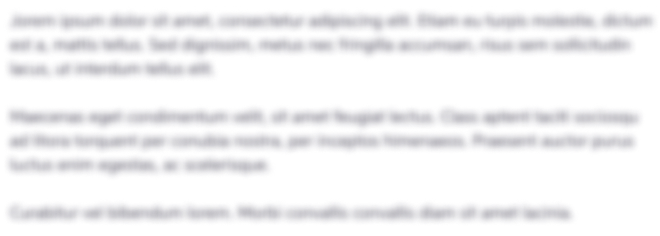
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started