Question
Please finish everything marked TODO AVL.java import java.util.ArrayList; import java.util.Collection; import java.util.List; public class AVL implements Tree { private int height; private int size; private
Please finish everything marked TODO
AVL.java
import java.util.ArrayList; import java.util.Collection; import java.util.List;
public class AVL
private int height; private int size; private BinaryNode
public AVL(){ this.root = null; this.height = 0; this.size = 0; this.RRotations = 0; this.LRotations = 0; }
public AVL(BinaryNode
// Access fields public int getRRotations(){ return this.RRotations; } public int getLRotations(){ return this.LRotations; } public BinaryNode
// TODO: updateHeight - same as BST public void updateHeight() {
}
// Traversals that return lists // TODO: Preorder traversal public List
// TODO: Inorder traversal public List
// TODO: Postorder traversal public List
/* TODO: rotateRight * x y * / \ / \ * y C ===> A x * / \ / \ * A B B C * You should never rotateRight if the left subtree is empty. * Make sure you increment the RRotations. */ public void rotateRight(BinaryNode
/* TODO: rotateLeft * x y * / \ / \ * y C <== A x * / \ / \ * A B B C * You should never rotateLeft if the right subtree is empty. * Make sure you increment the LRotations. * Symmetrical to above. */ public void rotateLeft(BinaryNode
/* TODO: possibleRotateRight * If the current node is unbalanced with the right tree height being smaller * than the left subtree height, rotate right. Otherwise, don't do anything. */ public void possibleRotateRight(BinaryNode
/* TODO: possibleRotateLeft * If the current node is unbalanced with the left tree height being smaller * than the right subtree height, rotate left. Otherwise, don't do anything. */ public void possibleRotateLeft(BinaryNode
/* TODO: mkBalanced * Given a node, balance it if the heights are unbalanced. * Hint: rotations!!! */ public void mkBalanced(BinaryNode
// Helpers for BST/AVL methods // TODO: extractRightMost - identical to BST public BinaryNode
// AVL & BST Search & insert same // TODO: search - identical to BST public BinaryNode
/* TODO: insert - slightly different from BST but similar logic * Hint: mkBalanced will be your best friend here. */ public void insert(E elem) { }
/* TODO: delete - slightly different from BST but similar logic * Hint: mkBalanced will be your best friend here. */ public BinaryNode
// Stuff to help you debug if you want // Can ignore or use to see if it works. static
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
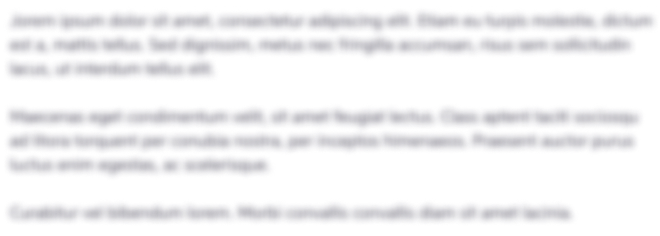
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started