Question
Please fix the following code and make sure that it compiles! (Make sure to have a copy constructor and a copy assignment declarations to the
Please fix the following code and make sure that it compiles!
(Make sure to have a copy constructor and a copy assignment declarations to the public area of your Time.h file. Implement the copy constructor and a copy assignment in your Time.cpp and add code to your main() to test your copy constructor and a copy assignment implementation.)
/** * Copy constructor */ Time(const Time & t);
/** * Copy assignment */ Time& operator =( const Time& rhs );
---------------------------------------------------------------------------------------------------------------------------------------------------
/* Main.cpp */
#include
#include
#include "Time.h"
#include
#include
using namespace std;
int main()
{
/* Default constructor test */
cout << "Object test: ";
Time test{};
if (test.is24Hour())
cout << "test is 24 hour formatted";
else
cout << "test is not 24 hour formatted";
cout << endl < /* Overloaded test: asLong() test */ cout << "Object raw: "; Time raw{14,02,50,600}; raw.set24Hour(true); if (raw.is24Hour()) cout << "raw is 24 hour formatted "; else cout << "raw is not 24 hour formatted "; cout << raw.toString() << " Time in ms: " << raw.asLong() << endl; /* Overloaded test: 24 hour */ cout << "Object now: "; Time now{13, 20, 04, 600}; now.set24Hour(true); if (now.is24Hour()) cout << "now is 24 hour formatted"; else cout << "now is not 24 hour formatted"; cout << endl < /* Overloaded test: 12 hour */ cout << "Object t_money: "; Time t_money {15, 55, 43, 900}; if (t_money.is24Hour()) cout << "t_money is 24 hour formatted"; else cout << "t_money is not 24 hour formatted"; cout << endl < /* Overloaded test: max time, min time [ALL WORKING, MIDNIGHT CASE HANDLED]*/ cout << "Object min: "; Time min {0,0,0,0}; cout << min.toString() << " "; min.set24Hour(true); cout << min.toString() << " "; cout << "Object max: "; Time max {23, 59, 59, 999}; cout << max.toString() << " "; max.set24Hour(true); cout << max.toString() << " "; /* Overloaded test: bad input */ cout << "Object bad1: "; Time bad1 {-30, 80, 109, 1010}; //Negative, out of bound values cout << bad1.toString() << " "; cout << "Object bad2: "; Time bad2 {'T', 'a', 6, 509}; //non-integer values cout << bad2.toString() << " "; cout << "Object bad3: "; Time bad3 {14, 43, 50, 1010}; //Out of bound only cout << bad3.toString() << " "; cout << "Object bad4: "; Time bad4 {15, 45, 20, 50}; cout << bad4.toString() << " "; /* Operator override test */ /* Output */ cout << "Override test: << "; Time over1{15, 45, 39, 120}; cout << "toString() output: " << over1.toString() << " "; cout << "Override output: " << over1 << " "; /* Less than: Tested <, >, and == */ cout << "Override test: < "; Time x{5, 45, 39, 120}; Time y{15, 45, 39, 120}; cout << "x == " << x.asLong() << ", y == " << y.asLong() << " "; if (x < y) cout << "x is < y "; else cout << "x is not < y "; /* Greater than: Tested >, <, and == */ cout << "Override test: > "; Time a{2,0,0,0}; Time b{3,0,0,0}; cout << "a == " << a.asLong() << ", b == " << b.asLong() << " "; if (b > a) cout << "b is > a "; else cout << "b is not > a "; /* Equals: both equality and inequality work */ cout << "Equality test: == "; Time c{2,30,30,50}; Time d{2,30,30,50}; Time e{5, 6, 7, 8}; Time f{10, 10, 10, 10}; cout << "c == " << c.asLong() << ", d == " << d.asLong() << ", e == " << e.asLong() << ", f == " << f.asLong() << " "; if (c==d) cout << "c==d "; else cout << "c!=d "; if (e==f) cout << "e==f "; else cout << "e!=f "; /* Plus: */ cout << "Addition test: + "; Time g{10,0,0,0}; Time h{15,45,30,0}; Time i{}; cout << "g == " << g << ", h == " << h << " "; i = g + h; cout << "g + h == " << i << " "; g = {12,0,0,0}; h = {13,0,0,0}; cout << "g == " << g << ", h == " << h << " "; i = g + h; cout << "g + h == " << i << " "; cout << "g == " << g << ", h == " << h << " "; cout << "g + h == " << i << " "; Time ms1 {21*60*60*1000}; Time ms2 {14*60*60*1000}; Time ms3{}; cout << "ms1 == " << ms1.asLong() << " or " << ms1 << ", ms2 == " << ms2.asLong() << " or " << ms2 << " "; ms3 = ms1+ms2; cout << "ms1 + ms2 == " << ms3.asLong() << " or " << ms3 << " "; cout << endl; /* Minus: */ cout << "Subtraction test: - "; Time j {20,30,0,0}; /* 5:00:00 AM */ Time k {5,0,0,0}; /* 8:30:00 PM */ Time l{}; l = j-k; cout << "j == " << j << ", k == " << k << endl; cout << "j == " << j.asLong() << "ms, k == " << k.asLong() << "ms" << ", j - k == " << l.asLong() << "ms or " << l << " "; cout << "Subtraction Test 2: millisecond ops "; j = {140500}; k = {200690}; cout << "j == " << j.asLong() << " or " << j << ", k == " << k.asLong() << " or " << k << " "; l = j-k; cout << "j-k == " << l.asLong() << ", or " << l << " "; cout << "Subtraction Test 3: Time code subtraction: "; j = {5,10,0,0}; //5:10:00 AM k = {20,20,0,0}; //8:20:00 PM l = j - k; /* Expected output 8:50:00 AM * */ cout << "j == " << j << ", k == " << k << endl; cout << "j == " << j.asLong() << "ms, k == " << k.asLong() << "ms" << ", j - k == " << l.asLong() << "ms "; cout << "j - k == " << l << " "; /* Assignment Operator */ /* Test standard copy */ cout << "Assignment Test: "; cout << "Copy Constructor Test: Time and_for_what(christmas_morning) "; Time christmas_morning {6,0,0,0}; Time and_for_what(christmas_morning); cout << "christmas_morning == " << christmas_morning << ", and_for_what == " << and_for_what << " "; christmas_morning.set24Hour(true); cout << christmas_morning << " on a Christmas morning, and for what?! "; cout << "Standard Copy Test: noon = lunch "; Time lunch {12,0,0,0}; Time noon = lunch; cout << "lunch == " << lunch << ", noon == " << noon; cout << " "; /* Test 24-hour boolean copy*/ cout << "24 Hour State Copy Test: evening = dinner in 24hr time "; Time dinner = {18,30,0,0}; //6:30 PM dinner.set24Hour(true); //Dinner is now 18:30:0:0 Time evening = dinner; cout << "dinner == " << dinner << ", evening == " << evening << " "; /* Test Copy chaining */ cout << "Copy chaining test: all values should be the same as the farthest time on the right. "; Time Another, Brick, In, The = {0}; Time Wall {13,44,25,650}; //Time is 1:44:25:650 cout << "Wall == " << Wall << " "; cout << "Another = Brick = In = The = Wall "; Another = Brick = In = The = Wall; // All Times should now be 1:44:25:650 cout << "Another == " << Another << ", Brick == " << Brick << ", In == " << In << ", The == " << The << ", Wall == " << Wall << " "; } ------------------------------------------------------------------------------------------------- /* Time.cpp */ #include #include #include #include "Time.h" #include #include using namespace std; //////////////////////Conversion methods/////////////////////////////////////// long hrs_to_ms(int i) { if (i < 0) cerr << "Method hrs_to_ms: non-positive value given. "; return i*60*60*1000; //hrs-->min-->sec-->ms } long min_to_ms(int i) { if (i < 0) cerr << "Method min_to_ms: non-positive value given. "; return i*60*1000; //min-->sec-->ms } long sec_to_ms(int i) { if (i < 0) cerr << "Method sec_to_ms: non-positive value given. "; return i*1000; } int time_in_ms(long t) { return t % 1000; } int time_in_sec(long t) { return (t/1000)%60; } int time_in_min(long t) { return (t/1000/60)%60; } int time_in_24hrs(long t) { return (t/1000/60/60)%24; } int time_in_12hrs(long t) { return (t/1000/60/60)%12; } ////////////////////////End Conversion Methods////////////// //Default Constructor: Time is at 0 ms, not 24 hr format Time::Time() : t_ms{0}, is_24_hr{false} {} //Overloaded Constructor: milliseconds only Time::Time(long time) : t_ms{time}, is_24_hr{false} { if (time < 0) cerr << "Time Constructor: non-positive value given for time "; /* Check for overflow values*/ if (time > numeric_limits cerr << "Time Constructor: time value overflow "; } //Overloaded Constructor: Hours, minutes, seconds, ms format Time::Time(int hours, int minutes, int seconds, int milli ) { /* Error checking: positive values */ if (hours < 0 || minutes < 0 || seconds < 0 || milli < 0) cerr <<"Time Constructor: non-positive value given "; if (hours > 23) cerr << "Time Constructor: invalid hour value "; if (minutes >= 60) cerr << "Time Constructor: invalid minute value "; if (seconds > 60) cerr << "Time Constructor: invalid seconds value "; if (milli > 999) cerr << "Time Constructor: invalid milliseconds value "; long h_conv = hrs_to_ms(hours); long m_conv = min_to_ms(minutes); long s_conv = sec_to_ms(seconds); t_ms = h_conv + m_conv + s_conv + milli; /* Check for overflow values */ if (t_ms > numeric_limits cerr << "Time Constructor: time value overflow "; is_24_hr = false; } /* Copy Constructor: copy long time from Time argument and * store in the new object's t_ms. Copy argument's 24 hr state * and store it in the new object's 24 hr state. */ Time::Time (const Time& t) : t_ms{t.t_ms}, is_24_hr{t.is_24_hr} {} //Destructor Time::~Time(void) {} ////////////////////////////////Methods//////////////////////////////////////////// long Time::asLong() const { return t_ms; } string Time::toString() const { int hrs, min, sec, ms; ostringstream s; /*Hour assignment: check for 24 hour flag, adjust hour assignment an AM/PM output */ if (is_24_hr) { hrs = time_in_24hrs(t_ms); min = time_in_min(t_ms); sec = time_in_sec(t_ms); ms = time_in_ms(t_ms); //Pipe data into the stringstream s << hrs << ":" << min << ":" << sec << ":" << ms; } /* Output as 12-hour time, add AM/PM to output string */ else { /* Check for midnight state */ if (time_in_12hrs(t_ms) == 0) hrs = 12; else hrs = time_in_12hrs(t_ms); min = time_in_min(t_ms); sec = time_in_sec(t_ms); ms = time_in_ms(t_ms); /* AM/PM check */ /* Check the time using time_in_24hrs, not time_in_12hrs, which is always < 12 */ if (time_in_24hrs(t_ms) < 12) s << hrs << ":" << min << ":" << sec << ":" << ms << " AM"; else s << hrs << ":" << min << ":" << sec << ":" << ms << " PM"; } return s.str(); } void Time::set24Hour(bool value) { /* DEBUG */ //cout << "set24Hour: value is " << value; is_24_hr = value; } bool Time::is24Hour() const { return is_24_hr; } //////////////////////////* Override Operators *////////////////////////////////////////////// /* Output: << acts as a toString() call */ ostream& operator <<(ostream& s, const Time& t) { s << t.toString(); /*Assign the time output of the passed Time object to the output stream*/ return s; } /* Less than: Compare lhs time to rhs time using asLong() method */ bool operator <(const Time& lhs, const Time& rhs) { bool result; if (lhs.asLong() < rhs.asLong() ) result = true; else result = false; return result; } /* Greater than: return !(a bool operator >(const Time& lhs, const Time& rhs) { bool result; if (lhs.asLong() == rhs.asLong()) result = false; else result = !(lhs < rhs); return result; } /* Equal to: Compare lhs.asLong() to rhs.asLong() for equality */ bool operator ==(const Time& lhs, const Time& rhs) { return (lhs.asLong() == rhs.asLong()); } /* Assignment Operator */ Time& Time::operator= (const Time &rhs) { t_ms = rhs.t_ms; //Assign right-hand time to new object's time is_24_hr = rhs.is_24_hr; //Assign right-hand time's hour-state to new object return *this; //return the newly created object } /* Plus operator: this + arg.asLong() */ Time Time::operator +(const Time& t) const { Time result {}; const long ms_in_day = 24*60*60*1000; result = (this->asLong() + t.asLong()) % ms_in_day; return result; } /* Minus Operator: this - arg.asLong() */ Time Time::operator -(const Time& t) const { Time result{}; const long ms_in_day = 24*60*60*1000; /* If operation is negative, give a positive answer by adding the maximum day millisecond value. * Otherwise, return the result as normal. */ if (this->asLong() - t.asLong() < 0 ) result = this->asLong() -t.asLong() + ms_in_day; else result = this->asLong() - t.asLong(); return result; } --------------------------------------------------------------------------------------------- /* Time.h */ #ifndef TIME_H_ #define TIME_H_ #include #include #include #include using namespace std; /** * Time class * * The Time class contains time as hours:minutes:seconds:milliseconds (AM/PM). */ class Time { public: /** * Constructor with zero values */ Time(); /** * Constructors with arguments */ Time(long time); Time(int hours, int minutes, int seconds, int milli); /** * Copy constructor */ Time(const Time& t); /** * Deconstructor */ virtual ~Time(); /** * Return time as a long value representing time in millisecondsF */ long asLong() const; /** * Display as a string in the format hours:minutes:seconds:milliseconds. * For example 1:45:30:56 PM * * The time is displayed as 24 hours if the 24 hour flag is set true. */ string toString() const; /** * Enable/disable 24 hour time display */ void set24Hour(bool value); /** * Return true if 24 hour time display is enabled */ bool is24Hour() const; /** * Output the time to an output stream */ friend std::ostream& operator <<(std::ostream&, const Time&); // Output a Time to an output stream /** * Define ordering relationships */ friend bool operator <(const Time& lhs, const Time& rhs); friend bool operator >(const Time& lhs, const Time& rhs); friend bool operator ==(const Time& lhs, const Time& rhs); /** * Copy assignment */ Time& operator =( const Time& rhs ); /** * Define addition and subtraction */ Time operator +(const Time&) const; Time operator -(const Time&) const; private: /** * Private members go here */ long t_ms; //Time variable, in milliseconds bool is_24_hr; //24 hour flag /* Define helper methods */ friend long hrs_to_ms(int i); friend long min_to_ms(int i); friend long sec_to_ms(int i); friend int time_in_ms(long t); friend int time_in_sec(long t); friend int time_in_min(long t); friend int time_in_24hrs(long t); friend int time_in_12hrs(long t); }; #endif /* TIME_H_ */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
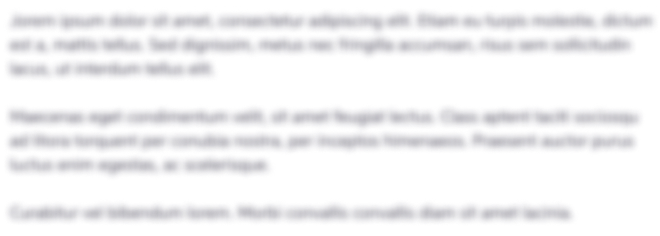
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started