Question
// Please follow the instructions and solve it by C++. (Mac) Thank you. Palindrome using STL Abstract Data Type (ADT) palindrome.cpp In this exercise, we
// Please follow the instructions and solve it by C++. (Mac) Thank you.
Palindrome using STL Abstract Data Type (ADT) palindrome.cpp
In this exercise, we are going to determine whether the user input text string is a palindrome using:
- built-in features of the string class, such as the constructor, iterator, element access and capacity;
- recursion;
- other STL ADT (ref. on Cplusplus.com Containers) such as stack and/or vector to determine whether the user input text string is a palindrome.
A palindrome is any word, phrase, or sentence that reads the same forward and backward. Here are some well-known palindromes:
Able was I, ere I saw Elba A man, a plan, a canal, Panama Desserts, I stressed Kayak
Write a bool function that uses recursion to determine if a string argument is a palindrome. The function should return true if the argument reads the same forward and backward. Demonstrate the function in a program.
Starter
palindrome_starter.cpp (on Github)
Please complete the attached palindrome test starter with STL string library calls.
Test Pattern
string testStrings[6] = { "ABLE WAS I ERE I SAW ELBA", "FOUR SCORE AND SEVEN YEARS AGO", "NOW IS THE TIME FOR ALL GOOD MEN", "DESSERTS I STRESSED", "ASK NOT WHAT YOUR COUNTRY CAN DO FOR YOU", "KAYAK" };
Sample Test Run
Running /home/ubuntu/workspace/COMSC/200/Week02/testPalindrome.cpp "ABLE WAS I ERE I SAW ELBA" is a palindrome. "FOUR SCORE AND SEVEN YEARS AGO" is NOT a palindrome. "NOW IS THE TIME FOR ALL GOOD MEN" is NOT a palindrome. "DESSERTS I STRESSED" is a palindrome. "ASK NOT WHAT YOUR COUNTRY CAN DO FOR YOU" is NOT a palindrome. "KAYAK" is a palindrome.
- palindrome.cpp
- Validation Test Run Result
Step by Step Solution
There are 3 Steps involved in it
Step: 1
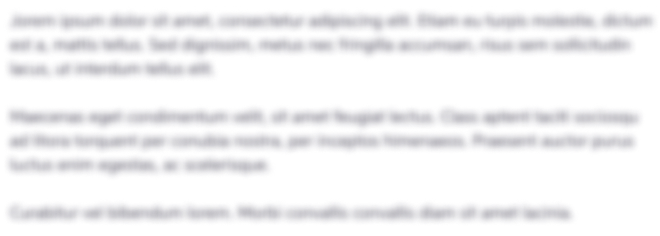
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started