Question
Please help. // -------------------------------------------------------- // DO NOT EDIT ANYTHING BELOW THIS LINE // -------------------------------------------------------- /** * Interface for an abacus for the AbacusGUI to use.
Please help.
// --------------------------------------------------------
// DO NOT EDIT ANYTHING BELOW THIS LINE
// --------------------------------------------------------
/**
* Interface for an abacus for the AbacusGUI to use.
*/
public interface Abacus {
/**
* Gets the number base of the abacus. This base
* will never be an odd number and never be less
* than 2.
*
* @return the number base of the abacus
*/
public int getBase();
/**
* Returns the number of places (bead columns) the
* abacus is using to represent the number. This
* will never be less than one.
*
* @return the number of places in use
*/
public int getNumPlaces();
/**
* Gets the number of beads in the top area of the
* abacus which are in-use for a given place. (The
* number of beads in the top which are pushed down
* to the center.)
*
* @param place the beads column of interest (0 is the right-most place)
* @return the number of beads currently in use
* @throws IndexOutOfBoundsException if the place requested is not in use
*/
public int getBeadsTop(int place);
/**
* Gets the number of beads in the bottom area of the
* abacus which are in-use for a given place. (The
* number of beads in the bottom which are pushed up
* to the center.)
*
* @param place the beads column of interest (0 is the right-most place)
* @return the number of beads currently in use
* @throws IndexOutOfBoundsException if the place requested is not in use
*/
public int getBeadsBottom(int place);
/**
* Adds the given string representation of a number to
* the current value of the abacus. The abacus is updated
* to this new position. It returns the steps to perform
* the add (snap shots of the abacus at each step). The
* abacus may be left in an "improper state" if the
* provided arguements are invalid.
*
*
A snapshot is required for each of the following steps:
*
- - the initial state
*
- - the final state
*
- - expansions (beads should not be moved, the abacus just
* becomes bigger/smaller)
*
- - exchanges (beads are exchanged in one step)
*
- - movement of X beads up OR down (not both at the same time)
* in ONE place on the bottom OR top of the abacus (not both
* at the same time)
*
*
*
* @param value the string representation of the value to add (e.g. "100" in base 10, or "1f" in base 16)
* @return the different positions the abacus was in (including the start and finish states)
* @throws NumberFormatException if string is not correct for the base
*/
public DynArr310
}
import java.util.Scanner;
/**
* A little GUI to help you interact with the abacus.
* Use with the command:
* java AbacusGUI
* or
* java AbacusGUI full
*/
public class AbacusGUI {
/**
* The main method that presents the GUI.
*
* @param args command line args (if first is "full" shows steps, otherwise ignored)
*/
public static void main(String[] args) {
boolean full = (args.length == 1 && args[0].equals("full"));
try (Scanner s = new Scanner(System.in)) {
Abacus a = null;
while(true) {
try {
System.out.print("Enter a base: ");
a = new MyAbacus(s.nextInt());
s.nextLine();
printAbacus(a);
break;
}
catch(RuntimeException e) {
System.out.println(e.toString());
}
}
while(true) {
System.out.print("What would you like to add? (Enter anything invalid to quit.) ");
if(full) {
fullPrintAdd(a, s.nextLine());
}
else {
a.add(s.nextLine());
printAbacus(a);
}
}
}
catch(Exception e) {
System.out.println("Goodbye!");
}
}
/**
* Adds the value to the abacus, then prints all the steps
* this is probably useful for debugging.
*
* @param a the abacus to add to
* @param value the value to add to the abacus
*/
public static void fullPrintAdd(Abacus a, String value) {
DynArr310
System.out.println("------------------------------");
System.out.println("- Starting State");
System.out.println("------------------------------");
printAbacus(steps.get(0));
System.out.println("------------------------------");
System.out.println("- Adding " + value);
System.out.println("------------------------------");
for(int i = 1; i < steps.size(); i++) {
printAbacus(steps.get(i));
}
System.out.println("------------------------------");
System.out.println("- Done");
System.out.println("------------------------------");
}
/**
* Prints a single abacus with amazing graphics.
*
* @param a the abacus to print
*/
public static void printAbacus(Abacus a) {
StringBuilder sb = new StringBuilder();
//build abacus structure
int bottomSize = a.getBase()/2;
int numPlaces = a.getNumPlaces();
int width = (numPlaces*4)+4;
//top
sb.append("/ ");
printRows(sb, numPlaces, "---"); //em-dashes
sb.append(" \\ ");
//top beads
for(int i = 0; i < 3; i++) {
sb.append("| ");
printRows(sb, numPlaces, " | "); //em-dashes
sb.append(" | ");
}
//middle
sb.append("| ");
printRows(sb, numPlaces, "---");
sb.append(" | ");
//bottom beads
for(int i = 0; i < (bottomSize)+2; i++) {
sb.append("| ");
printRows(sb, numPlaces, " | "); //em-dashes
sb.append(" | ");
}
//bottom
sb.append("\\ ");
printRows(sb, numPlaces, "---");
sb.append(" / ");
//put beads in place
for(int i = 0; i < numPlaces; i++) {
//top beads
int topBeads = a.getBeadsTop(i);
for(int j = 0; j < 3; j++) {
if(topBeads != j) {
int spot = calcSpotTop(i, width, 3-j);
sb.setCharAt(spot,'O');
}
}
int bottomBeads = a.getBeadsBottom(i);
for(int j = 0; j < bottomBeads; j++) {
int spot = calcSpotBottom(i, width, j);
sb.setCharAt(spot,'O');
}
for(int j = 0; j < bottomSize-bottomBeads; j++) {
int spot = calcSpotBottom(i, width, bottomSize-j+1);
sb.setCharAt(spot,'O');
}
}
System.out.println(sb.toString());
}
/**
* Calculaton for the placement of a single bead
* on the top.
*
* @param place the place (column) of the abacus (0 is rightmost)
* @param width the width of the ascii art
* @param num the bead position (1=top,2=middle,3=bottom)
* @return the index into the ascii art to draw the bead
*/
private static int calcSpotTop(int place, int width, int num) {
int spot = (width*num) + (width - ((place+1)*4) - 1);
return spot;
}
/**
* Calculaton for the placement of a single bead
* on the bottom.
*
* @param place the place (column) of the abacus (0 is rightmost)
* @param width the width of the ascii art
* @param num the bead position (1 to base/2, 1 is at the top)
* @return the index into the ascii art to draw the bead
*/
private static int calcSpotBottom(int place, int width, int num) {
int spot = (width*(num+5)) + (width - ((place+1)*4) - 1);
return spot;
}
// TO DO: add your implementation and JavaDocs
public class MyAbacus implements Abacus {
// ADD MORE PRIVATE MEMBERS HERE IF NEEDED!
// Remember: Using an array in this class = no credit on the project!
public MyAbacus(int base) {
// throws IllegalArgumentException if base is invalid
// remember: an abacus should always have at least one
// column!
}
public int getBase() {
// O(1)
return -1; //default return, make sure to remove/change
}
public int getNumPlaces() {
// O(1)
return -1; //default return, make sure to remove/change
}
public int getBeadsTop(int place) {
// O(1)
return -1; //default return, make sure to remove/change
}
public int getBeadsBottom(int place) {
// O(1)
return -1; //default return, make sure to remove/change
}
public boolean equals(MyAbacus m) {
// O(N) where N is the number of places currently
// in use by the abacus
return false; //default return, make sure to remove/change
}
public DynArr310
// Hints:
// see: https://docs.oracle.com/javase/8/docs/api/java/lang/Integer.html#parseInt-java.lang.String-int-
// and: https://docs.oracle.com/javase/8/docs/api/java/lang/String.html#charAt-int-
// Also... I personally found a recursive helper function really, really useful here...
// Important: each Abacus in the DynArr310
// be a copy of this abacus. If you just add this abacus over and over
// you'll just get the final abacus shown multiple times in the GUI.
return null; //default return, make sure to remove/change
}
// --------------------------------------------------------
// example testing code... edit this as much as you want!
// --------------------------------------------------------
public static void main(String[] args) {
//this is the sequence from the project description
Abacus a = new MyAbacus(10);
DynArr310
AbacusGUI.printAbacus(a);
AbacusGUI.fullPrintAdd(a, "36");
AbacusGUI.fullPrintAdd(a, "12");
AbacusGUI.fullPrintAdd(a, "2");
AbacusGUI.fullPrintAdd(a, "12");
AbacusGUI.fullPrintAdd(a, "10");
AbacusGUI.fullPrintAdd(a, "2");
AbacusGUI.fullPrintAdd(a, "68");
AbacusGUI.fullPrintAdd(a, "50");
AbacusGUI.fullPrintAdd(a, "10");
AbacusGUI.fullPrintAdd(a, "5");
AbacusGUI.fullPrintAdd(a, "3");
AbacusGUI.fullPrintAdd(a, "128");
AbacusGUI.fullPrintAdd(a, "3000000");
}
}
/**
* Prints a row of some pattern to the given StringBuilder.
*
* @param sb the builder to append to
* @param numPlaces the number of the sequence to add
* @param seq the sequence to repeat
*/
private static void printRows(StringBuilder sb, int numPlaces, String seq) {
for(int i = 0; i < numPlaces; i++) {
sb.append(seq);
if(i != numPlaces-1) sb.append(" ");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
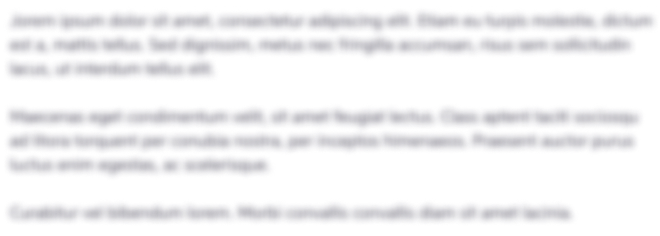
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started