Question
PLEASE HELP FILLING OUT THESE CLASSES FOR HUFFMAN CODING public class BitOutputStream { //add additional protected variables as needed //do not modify the public methods
PLEASE HELP FILLING OUT THESE CLASSES FOR HUFFMAN CODING public class BitOutputStream { //add additional protected variables as needed //do not modify the public methods signatures or add public methods protected DataOutputStream d; public BitOutputStream(String filename) { try { d = new DataOutputStream(new FileOutputStream(filename)); } catch (IOException e) { } } public void writeBit(char bit) { //PRE: bit is a '0' or a '1' } public void close() { } } ------------------------------------------------------------------------------------------------------------------- public class BitInputStream { //add additional protected variables as needed //do not modify the public methods signatures or add public methods protected DataInputStream d; public BitInputStream(String filename) { try { d = new DataInputStream(new FileInputStream(filename)); } catch (IOException e) { } } public int readBit() { //return the next bit in the file } public void close() { } } -------------------------------------------------------------------------------------------------------- public class HuffmanOutputStream extends BitOutputStream { public HuffmanOutputStream(String filename, String tree, int totalChars) { super(filename); try { d.writeUTF(tree); d.writeInt(totalChars); } catch (IOException e) { } } } ------------------------------------------------------------------------------------------------------- public class HuffmanInputStream extends BitInputStream { //add additional private variables as needed //do not modify the public method signatures or add public methods private String tree; private int totalChars; public HuffmanInputStream(String filename) { super(filename); try { tree = d.readUTF(); totalChars = d.readInt(); } catch (IOException e) { } } public String getTree() { } public int getTotalChars() { } } -------------------------------------------------------------------------------------------------- public class HuffmanTree { //add additional private variables as needed //do not modify the public method signatures or add public methods private class Node { private Node left; private char data; private Node right; private Node parent; private Node(Node L, char d, Node R, Node P) { left = L; data = d; right = R; parent = P; } } private Node root; private Node current; //this value is changed by the move methods public HuffmanTree() { root = null; current = null; } public HuffmanTree(char d) { //makes a single node tree } public HuffmanTree(String t, char nonLeaf) { //Assumes t represents a post order representation of the tree as discussed //in class //nonLeaf is the char value of the data in the non-leaf nodes //in classs we used (char) 128 for the non-leaf value } public HuffmanTree(HuffmanTree b1, HuffmanTree b2, char d) { //makes a new tree where b1 is the left subtree and b2 is the right subtree //d is the data in the root } //use the move methods to traverse the tree //the move methods change the value of current public void moveToRoot() { } public void moveToLeft() { } public void moveToRight() { } public void moveToParent() { } public boolean atLeaf() { //returns true if current references a leave other wise returns false } public char current() { //returns the data value in the node referenced by current } //the iterator returns the path (a series of 0s and 1s) to each leaf public class PathIterator implements Iterator { public PathIterator() { } public boolean hasNext() { } public String next() { } public void remove() { //optional method not implemented } } public Iterator iterator() { //return a PathIterator object } public String toString() { //return a post order representation of the tree //using the format we discussed in class } --------------------------------------------------------------------------------------------------------- import java.io.*; import java.util.*; public class HuffmanEncode { public HuffmanEncode(String in, String out) { //Implements the huffman encoding algorithm //Add private methods as needed } public static void main(String args[]) { new HuffmanEncode(args[0], args[1]); } } ----------------------------------------------------------------------------------------------------------- import java.io.*; import java.util.*; public class HuffmanDecode { public HuffmanDecode(String in, String out) { //Implements the huffman decoding algorithm //Add private methods as needed } public static void main(String args[]) { new HuffmanDecode(args[0], args[1]); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
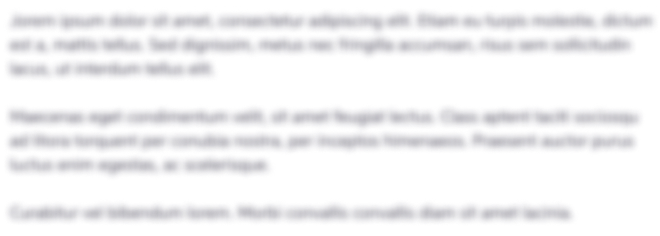
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started