Question
Please help get started on this code. Where would be a good beginning place? What issues could you see a new programmer making when attempting
Please help get started on this code. Where would be a good beginning place? What issues could you see a new programmer making when attempting to code this? Please provide as much information as you can.
The objective of this program is to create a list of flight route entries, which allows a user to add, delete, and search for a given route. A table of flights will be contained within a dynamically allocated array in which each array element points to a flight structure. The flights will be initialized by reading them into a table from a text file.
Detail:
The initial flight will be read from a text file. The file will contain the Flight Number (int), Departure City Code (int), Arrival City Code (int), and Cost in dollars (int, with no cents) for each flight. There will be one flight per line. Note that there may be more than one flight with the same Departure and Arrival City code. However, the Flight Numbers are unique. Also, the first line will have a single integer value that identifies the number of flight records contained within the file.
Example Flight Data File:
Flights.txt
4
4536 1 3 456
4543 2 6 1200
5651 2 3 546
6769 1 3 550
A structure (Flight struct) should be used with the following members:
int flightNumber; int depCityCode; int arrCityCode; int cost;
An array of pointers to Flight structs (A Flight pointer array) will be used to contain the table of flights. NOTE: This array is not an array of Flight structs but an array of pointers to Flight structs. It should allow the user to add, remove, and search for given flights based on the Departure City and Arrival City. The Flight pointer array is a dynamically allocated table of pointers to Flight structs. Each time a flight (flight struct) is added to the table, the flight struct variable must be created using malloc() and initialized with the given flight information.
Note that if no Flight struct is assigned to a given array location, that location must be set to NULL. Also if a flight struct is removed from an array location, that location must be set to NULL *after* using free() to remove the given flight from memory.
The syntax for the Flight pointer array is the following:
// array of pointers to Flight structs Flight **FlightPointerArray;
The syntax for accessing the struct variabls is as follows:
FlightPointerArray[i]->depCityCode;
The following functions must be implemented:
int initFlightPointerArray(int maxLength, const char * flightFileName);
int findFlight(int deptCode, int arrCode);
int printMatchingFlights(int depCode, int arrCode);
int addFlight(int flightNum, int depCode, int arrCode, int cost);
int removeFlight(int flightNum);
int initFlightPointerArray(int maxLength, const char * flightFileName);
The initFlightPointerArray() will accept two parameters, the first being the maximum length of the Flight pointer array and the other being the file to use for initializing the Flight pointer array. Note that the inputFile may contain fewer routes than the size of the Flight pointer array. There needs to be code within this function to open, read, and close the inputFile. Also, the code must dynamically allocate each Flight struct. The Flight pointer array must also be dynamically allocated too. You will want to test this by setting the maxLength Flight 0 Flight 1 Flight 2 Flight N NULL(No route) 3 value higher than the number of flight records within the inputFile to allow for additions and removals of flight records. The function should return the number of flights created. If an error occurs (file not found, file read error, etc) it should return -1.
Sample code to creating the flight pointer array
(with maxLength set) FlightPointerArray = malloc( maxLength * sizeof(Flight *) );
Sample code for creating an new flight entry:
FlightPointerArray [location] = malloc(sizeof(Flight)); FlightPointerArray [location]->depCityCode = depCode; int findFlight(int deptCode, int arrCode);
The findFlight() function should search sequentially through the list for a matching route with the given Departure and Arrival City codes. It should stop at the first match it finds and return the array index. If no matches are found, it should return -1. An entry is considered a match if both the given Departure City and Arrival City Codes match the ones in within the Flight struct.
int printMatchingFlights(int depCode, int arrCode);
The printMatchingFlights() function should print out all the data for each of the Flight structs within the Flight pointer array that match the given Departure and Arrival City codes. Be sure to watch for empty (NULL) entries within the table. It must return the total number of matching flights. An entry is considered a match if both the given Departure City and Arrival City Codes match the ones in within the flight pointer array.
int addFlight(int flightNum, int depCode, int arrCode, int cost);
The addFlight() function will create a new Flight with the given parameters and add it to the Flight pointer array if there is an empty slot AND if there is no matching Flight Number (the Flight Numbers must be unique). It should scan the Flight pointer array sequentially looking for an empty slot (NULL Indicates empty slot). It should create a new Flight with the given parameters and assign it to the first empty slot provided there is not an already existing Flight with the same Flight number. It must use malloc() function for creating the Flight struct and store its pointer into the first available slot (array index location). It should return the array index that was used if successful, other return a -1 if there are no 4 empty array locations left or if the given Flight number already exists with the same Flight number. i
nt removeFlight(int flightNum);
The removeFlight() function will remove the flight struct from the Fight pointer array whose Flight Number matches the given Flight Number. It will scan the Flight pointer array sequentially looking for a match. If one is found, the flight struct should be removed by calling the free() function on the matching flight and setting that array location to NULL. The removeFlight() function should return the array index of the Flight that was removed. If the given Flight number does not exist, the function should return -1.
Requirements:
1. The following functions must be implemented as described within the above detail section: int initFlightPointerArray(int maxLength, const char * flightFileName); int findFlight(int deptCode, int arrCode); int printMatchingFlights(int depCode, int arrCode); int addFlight(int flightNum, int depCode, int arrCode, int cost); int removeFlight(int flightNum); The functions will be tested to verify they operate as described in the above section.
2. The array of Flight structs must be implemented as an array of pointers to Flight structs. See the detail section for additional description and sample code.
3. Write your own main() function with various testing routines to test the required functions. I will use my own main() function to test your functions.
4. Submit a Makefile for building your program
5. Use the proper methods of malloc() and free().
6. The program should compile without any errors or warnings.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
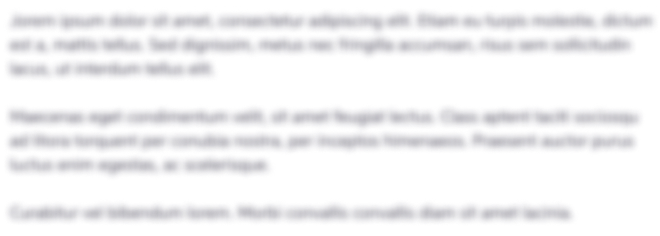
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started